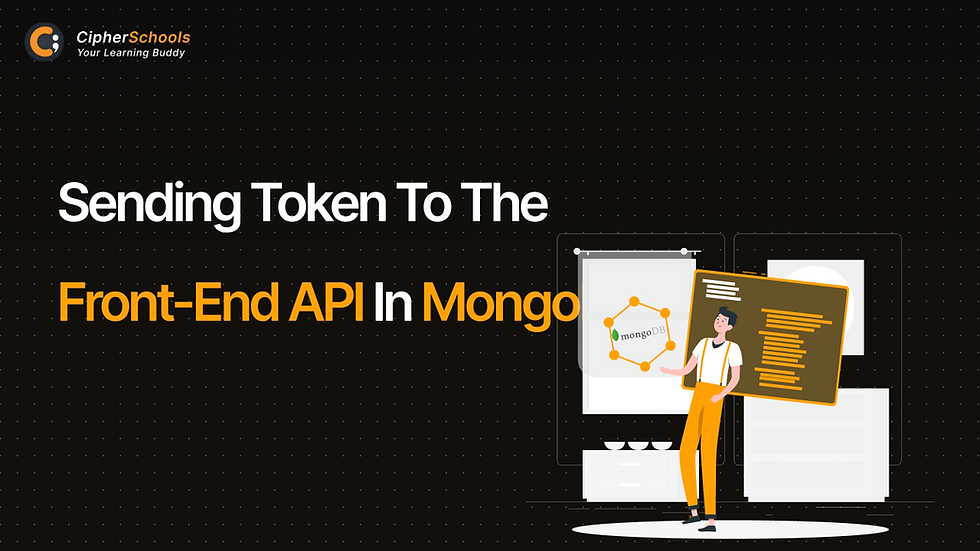
Securing user data and maintaining robust authentication processes are critical in today's digital ecosystem for any website. As more services rely on APIs to communicate with front-end interfaces, developers must create secure and fast methods of transmitting and verifying user identity.
One popular technique is tokens, which provide a streamlined way to authenticate users and authorize their access to protected resources.
This article focuses on the steps and best practices in sending tokens to the front-end API in a MongoDB context. MongoDB, a robust NoSQL database, provides a versatile framework for developing scalable and secure applications.
Developers can improve the security of their apps and deliver a seamless user experience by exploiting MongoDB's capabilities and implementing token-based authentication.
Throughout this MERN stack article series, we will look at the fundamentals of token-based authentication, emphasizing its benefits and how it fits into the context of MongoDB.
We will review the implementation specifics, including how to generate and validate tokens safely and incorporate them into the front-end API smoothly.
This article's main points are as follows:
Understanding token-based authentication: We will define tokens and their significance in safeguarding API connections. We'll go over the benefits of token-based authentication, such as statelessness, scalability, and simplicity of implementation.
Configuring a MongoDB environment: We will walk you through the procedures required to set up a MongoDB database for token-based authentication. This involves the creation of user collections, the management of roles and permissions, and the establishment of secure connections.
Token generation and issuance: We will examine different token generation techniques and the need to use cryptographic algorithms to ensure token integrity and prevent manipulation. We will also discuss token expiration and refresh mechanisms.
Token authentication in the front-end API: We will show how to implement token-based authentication into the front-end API. We'll review methods for securely transmitting tokens, storing them on the client side, and adding token verification into API queries.
Best practices and security considerations: This section will highlight the most important best practices to follow while establishing token-based authentication in a MongoDB environment. We will review tactics for avoiding common security flaws and recommend token management, revocation, and renewal.
By the end of this tutorial, you'll have a firm grasp of how to use tokens to secure your front-end API in a MongoDB context.
You will be given the information and tools you need to improve the authentication and security of your web apps, resulting in a robust and user-friendly experience for your users.
Configuring a MongoDB environment
Setting Up MongoDB in Windows
MongoDB's website has all installation instructions, and the database is compatible with Windows, Linux, and Mac OS.
Step 1: After clicking on the link (http://www.mongodb.org/downloads),
Step 2: After downloading, double-click this setup file to install it. Take the following steps:
Click on the Next button.
Step 3: Select Complete to finish installing MongoDB.
Step 4: Finally, click the option labeled "Run services as Network service user."
Step 5: The setup system will also prompt you to install MongoDB Compass, MongoDB's official graphical user interface (G.U.I.). You can install it as well if you check the box.
Step 6: Once the installation is complete, you must start MongoDB by following the steps below:
Launch Command Prompt.
Filename: C:Program FilesMongoDBServer4.0bin
Enter the command mongod to start the server.
You can now start your MongoDB database in this manner. You must now use the following command to start the MongoDB primary client system:
C:\Program Files\MongoDB\Server\4.0\bin>mongo.exe
Token generation and issuance in Mongodb
In MongoDB, token creation and issuance entail establishing secure tokens and distributing them to authenticated users for later API authentication and authorization. Let's go over the steps one by one:
User Authentication: Users must authenticate using their credentials, such as a username and password, before creating and issuing tokens. Typically, this authentication method entails querying the MongoDB database to validate the user's credentials.
Token Generation: After successful authentication, a token must be generated for that user. Tokens often comprise a random string of characters or encrypted data. Tokens can be stored within user documents or in separate token-specific collections in MongoDB.
Token Payload: The token's payload may contain useful information, such as the user's I.D., roles, or permissions, which can be utilized for authorization. To maintain security, the token payload should not include sensitive information such as passwords.
Token Expiry: Tokens frequently include an expiration date to ensure they are only valid for a certain amount of time. The expiry time can be set based on the requirements of the application and security concerns. MongoDB has options for automatically invalidating or removing expired tokens.
Token Distribution: Once the token has been generated, it must be distributed to the user. This can be performed by returning the token as part of the authentication response or securely keeping it on the client side (for example, via browser cookies or local storage). Security requirements and implementation preferences determine the mechanism of token transfer.
Token Validation: When the user makes subsequent API queries, the token must be validated to confirm its authenticity and integrity. The validation process entails inspecting the token's signature, securing its expiration date, and ensuring it has not been changed.
Token Revocation: In some instances, it may be required to revoke a token before it expires. This can occur due to user logout, password reset, or suspicious activity. MongoDB supports efficient token revocation by keeping a blacklist or invalidation list that prevents revoked tokens from being used.
Token Refreshment: Token refreshment can extend a user's session without re-authentication. This entails creating a new token with a longer expiry time while keeping the user's authentication status.
By following these methods, developers may efficiently implement token generation and issuance in MongoDB, improving the security and authentication of their apps.
To maintain the integrity and protection of user data, it is critical to employ secure practices such as strong token encryption, secure token storage, and proper token lifecycle management.
Token authentication in the front-end API
Step 1: We'll make a new response and set the status code to 200 because we successfully authenticated here, and we'll send a JSON response where we can send a message but, more importantly, where we can set our token. So, there, we will return the previously produced token.
res.status(200).json({
token: token
});
Step 2: Go to the front end once the backend is complete. We'll add a new login user () method to the auth.service.ts file. We need an email address and a password there because we need to communicate data to the backend.
And then we will submit a post request 0 and a post request to that same URL, well almost, and the end will be /login to target that newly established route, but we will also build our auth data as we did for signing up and append out AuthData as follows:
loginUser(email: string, password: string){
const authData: AuthData= {email: email, password: password};
this.http.post("http://localhost:3000/api/user/login",authData);
}
Step 3: Now, we must subscribe to it, and to do so, we console log the answer to see what is contained within it. For valid credentials, we should see the token there.
loginUser(email: string, password: string){
const authData: AuthData= {email: email, password: password};
this.http.post("http://localhost:3000/api/user/login",authData)
.subscribe(response => {
console.log(response);
});
}
Step 4: Now, we need to connect that newly connected service method to the login component, and for that, we will inject our auth service of type AuthService into the login component once more. We must add the import to that at the top, as follows:
import {AuthService} from '../auth.service';
constructor(public authservice: AuthService){}
Step 5: In our login () method, we'll look for instances where our form is invalid. So, if our form is invalid, we'll add a return statement. Otherwise, we will send that request by invoking our authservice's loginUser() method. We send the email and password values to that method in the following manner:
onLogin(form: NgForm){
if(form.invalid){
return;
}
this.authservice.loginUser(form.value.email, form.value.password);
}
If we try to log in after signing up, we will get an error saying, "user1 is not defined."
Step 6: To resolve this, we will return to our user.js file, where we use the user1 variable, which is not within the scope of the second blocks. So, we'll make another variable, fetch the user, and set the user1 value to it so that it can be accessed in the second and then block.
When we return to our application and attempt to log in, the token will appear in the developer console.
Best practices and security considerations
Token authentication in MongoDB's front-end API entails implementing the methods required to authenticate and authorize requests using tokens. Let's get into the specifics of token authentication in the front-end API:
Token Transmission: The created token must be securely communicated from the server to the client-side application following successful authentication. The token can be included in the response payload, as a response header, or by using cookies or local storage.
Token Storage: The token must be safely saved on the client side. HTTP-only cookies are suggested for web applications since they are not accessible to JavaScript code and provide additional protection against cross-site scripting (XSS) attacks. Local storage can also be used, although efforts must be taken to avoid XSS issues.
Request Authorization: The token must be supplied in the request headers for authentication and authorization when making subsequent queries to the front-end API. The most popular technique is to include the token as a bearer token in the "Authorization" header, using the format: "Bearer token."
Token Validation: To ensure the authenticity and integrity of the token, the front-end API must include token validation procedures on the server. This entails authenticating the token's signature and determining its expiration date. Token validation in MongoDB-based apps can be simplified using libraries such as JSON web token or Passport.js.
Middleware Implementation: Middleware methods can be used in the front-end API to manage token authentication and authorization. These middleware routines intercept incoming requests, extract and validate the token, and implement access control based on the token's payload (for example, user roles and permissions).
Access Control: The token payload can include information about the user's roles and permissions. The front-end API can use this information to construct access control logic that allows or denies access to specified resources or actions. This can be accomplished through the use of role-based access control (RBAC) or the implementation of custom authorization logic.
Token Revocation: If a token needs to be invalidated or revoked (for example, due to user logout or suspicious activity), a means for managing a token blacklist or invalidation list should be in place. This prohibits revoked tokens from being received by the front-end API, even if they are still valid.
Token Refreshment: To ensure a consistent user experience and extend sessions without requiring frequent re-authentication, token refreshment can be used. This entails issuing a new token with a longer expiration time while keeping the user's authentication state intact. For this purpose, refresh tokens can be used.
Developers may provide secure access to protected resources while maintaining a robust authentication method by implementing token authentication in MongoDB's front-end API. To avoid potential security risks and protect user data, it is critical to consider security best practices such as secure token transfer, proper token storage, and regular token validation.
Conclusion
In this post, we looked at how to transmit tokens to the front-end API in a MongoDB context, focusing on improving authentication and security. Token-based authentication is a strong and adaptable solution to securing API connections, ensuring seamless user experiences and comprehensive data security.
Developers can execute token generation, issuance, and validation securely and quickly by exploiting MongoDB's features. We discussed the significance of user authentication, token generation, and including essential information in the token's payload for authorization purposes. We also discussed token expiry, revocation, and refreshment techniques to enable secure token management.
Token authentication in the front-end API necessitates careful thought about secure token transmission and storage strategies. Developers can safeguard the confidentiality and integrity of tokens by following best practices such as using secure protocols, HTTP-only cookies, and protecting against XSS vulnerabilities.
Furthermore, we emphasized the importance of server-side token validation and the creation of middleware functions for seamless token authentication. We addressed token-based access control, which allows for fine-grained authorization for various user roles and permissions.
It is critical to prioritize security and validate and update token authentication systems regularly. Developers must be on the lookout for potential flaws such as token theft, misuse, or unauthorized access. Implementing token revocation procedures and keeping an invalidation list can mitigate such issues.
By adopting these practices into your MongoDB-powered applications, you can create a strong authentication framework, protect user data, and deliver a secure user experience. Token-based authentication in the front-end API improves security, scalability, ease of deployment, and the capacity to adapt to changing application requirements.
As technology progresses and security risks evolve, staying current on the newest best practices and security procedures is critical to ensure that your MongoDB applications are fully protected.
Developers can confidently construct secure and efficient online apps that match the needs of today's digital ecosystem by combining the strength of MongoDB's NoSQL capabilities with token-based authentication.
Interesting F.A.Q.s
What is the starting package for a MERN full stack developer?
According to Glassdoor, the average income for a MERN Stack Developer in India is Rs.420,000 annually.
Which are the top firms looking for MERN stack developers
According to Glassdoor, the companies looking for MERN Stack Developers with high salaries are listed below.
Stay tuned to CipherSchools for more interesting tech articles.
留言