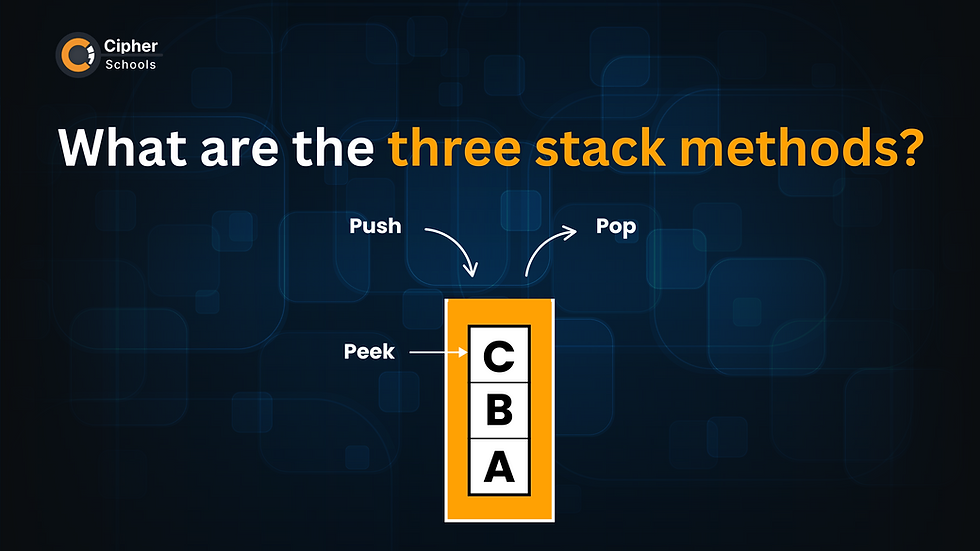
In data structures, a stack is a linear collection of elements managed in a Last-In-First-Out (LIFO) order. This means the last element added is the first one removed, making stacks ideal for scenarios where you need to reverse order or trace back through actions. Three essential operations are commonly used to manipulate data within a stack: push, pop, and peek (or top). Let’s take a closer look at each of these methods, along with examples in C++ to illustrate their functionality.
1. Push Operation
The push operation adds an element to the top of the stack. It’s akin to stacking books: each new book is placed on top of the stack, and it becomes the first item to access next.
How Push Works:
When an element is pushed, it is stored at the highest available position in the stack. This operation is integral in situations where data needs to be sequentially added for later retrieval in reverse order.
Example in C++:
#include <iostream>
#include <stack>
int main() {
std::stack<int> myStack;
myStack.push(5); // Adds 5 to the stack
myStack.push(10); // Adds 10 to the stack
std::cout << "Top element after pushes: " << myStack.top() << std::endl; // Outputs 10
return 0;
}
In this example, pushing 5 and then 10 onto the stack makes 10 the top element. The push method is critical for sequentially adding data to the stack, following the LIFO order.
2. Pop Operation
The pop operation removes the element at the top of the stack, which is the most recently added element. This operation is essential for retrieving and discarding items in the reverse order of their insertion.
How Pop Works:
When pop is called, the element on the top is removed, and the next item beneath it becomes the new top. This is helpful in scenarios where an order needs to be reversed or backtracked.
Example in C++:
myStack.pop(); // Removes the top element (10) from the stack
std::cout << "Top element after pop: " << myStack.top() << std::endl; //
Outputs 5
Here, calling pop removes the most recent addition, 10, leaving 5 as the top element. The pop operation helps keep the stack clean and accessible by removing elements as needed.
3. Peek (or Top) Operation
The peek or top operation allows you to view the element at the top of the stack without removing it. This is useful when you need to check the most recent element added without modifying the stack.
How Peek Works:
You can access the current top element without any changes by calling top. Peek operations are commonly used in applications requiring frequent reference to the top element while maintaining stack integrity.
Example in C++:
std::cout << "Current top element: " << myStack.top() << std::endl; // Outputs 5
The top function displays the top item without removing it, making it ideal for situations where you need to inspect the stack's most recent addition without modifying the stack itself.
Conclusion
The push, pop, and peek methods are fundamental operations for managing stacks in data structures, enabling a systematic way to add, remove, and access elements in a LIFO manner. Mastering these three operations is essential for working with stacks effectively and is a key skill for data manipulation in programming. With a solid understanding of push, pop, and peek, you’re well-prepared to utilise stacks in a variety of applications, from managing browser history to implementing algorithms in data structures.
Read Also - What is stack and its uses?
Comments