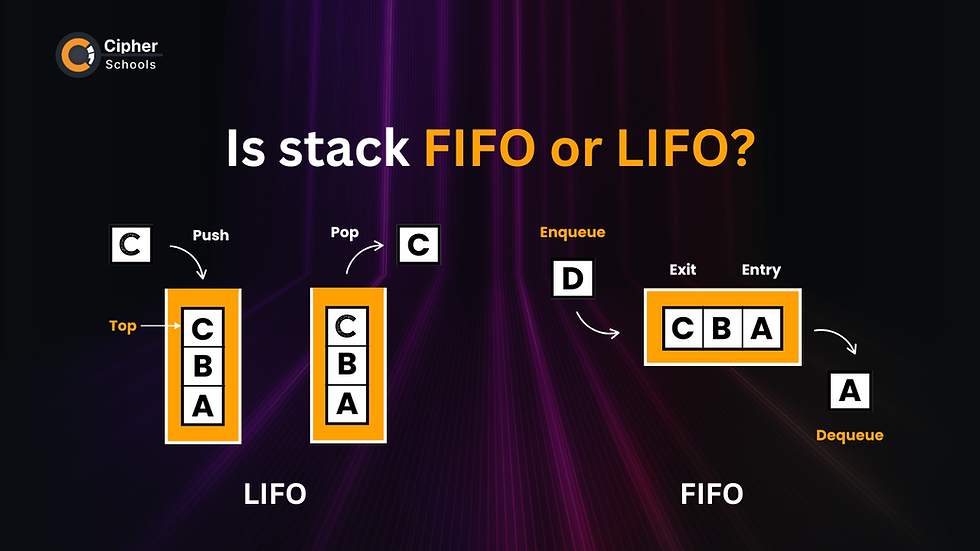
When discussing data structures, terms like FIFO (First-In-First-Out) and LIFO (Last-In-First-Out) are often used to describe how elements are accessed. Understanding the difference between these two can help you grasp the fundamental nature of various data structures. This article focuses on the stack in the data structure, answering whether it operates as FIFO or LIFO.
Understanding FIFO and LIFO
Before diving into stacks, let’s break down what FIFO and LIFO mean:
FIFO (First-In-First-Out): The first element added is the first one to be removed. This is like waiting in a queue at a store where the person at the front of the line is served first.
LIFO (Last-In-First-Out): The last element added is the first one to be removed. This is similar to a stack of plates where the last plate placed on top is the first to be taken off.
Why a Stack is LIFO
A stack follows the LIFO principle, which means the last item added is the first one to be removed. This is evident in how push and pop operations work:
Push: Adds an element to the top of the stack.
Pop: Removes the top element from the stack.
The LIFO nature of stacks makes them perfect for applications where the most recent addition should be the first to be accessed or removed, such as function call stacks in programming or the undo operation in text editors.
Practical Examples of LIFO in a Stack
Consider the example of an undo feature in text processing software. Each action (typing, deleting, formatting) is pushed onto a stack. When the user presses undo, the last action performed is popped from the stack, reverting it.
Code Snippet in C++:
#include <iostream>
#include <stack>
int main() {
std::stack<int> myStack;
myStack.push(1);
myStack.push(2);
myStack.push(3);
std::cout << "Top element: " << myStack.top() << std::endl; //
Outputs 3
myStack.pop();
std::cout << "Top element after pop: " << myStack.top() << std::endl; // Outputs 2
return 0;
}
In this example, 3 is the last element added and the first to be removed, showcasing the LIFO behavior.
Conclusion
A stack is indeed a LIFO data structure. Unlike a queue, which operates under FIFO principles, a stack ensures that the most recent element added is the first one to be removed. Understanding this principle helps in implementing stacks effectively in programming, whether it’s for navigating function calls, managing browser history, or supporting undo operations in applications.
Read, Also - What are the three stack methods?
Comments