Where is Stack Used in Real Life?
- Pushp Raj
- Nov 27, 2024
- 3 min read
Updated: Dec 3, 2024
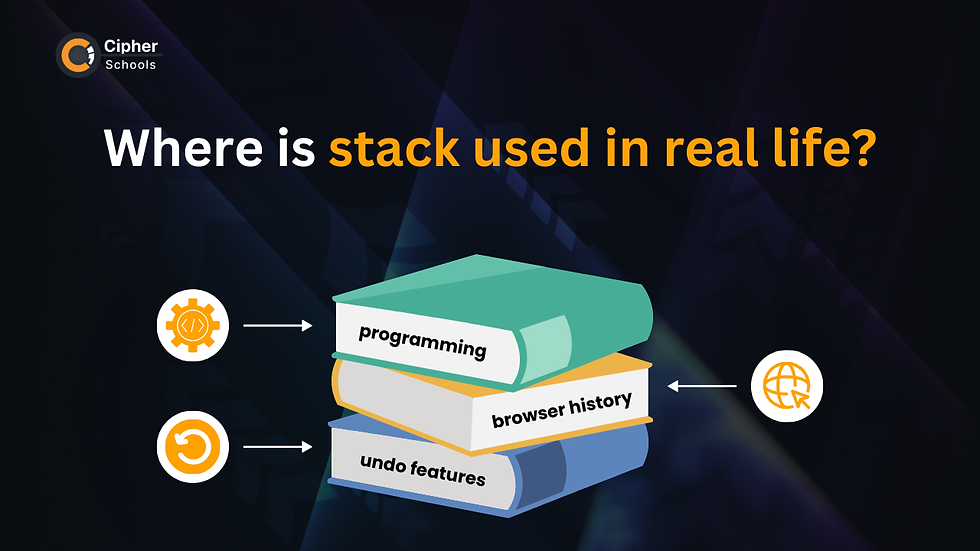
Data structures are fundamental in computer science, providing the framework for managing and organizing data. The stack in data structure stands out for its simplicity and practical applications. From software to daily life analogies, stacks are essential in handling specific tasks efficiently.
Understanding the Stack in Data Structure
A stack is a linear data structure that operates on the Last-In-First-Out (LIFO) principle. This means that the last item added to the stack is the first one removed. Stacks' straightforward nature makes them ideal for a variety of real-life and software-based tasks where order and reversibility are key.
Sample Stack Code in C++:
#include <iostream>
#include <stack>
using namespace std;
int main() {
stack<int> myStack;
// Pushing elements onto the stack
myStack.push(10);
myStack.push(20);
myStack.push(30);
// Displaying and popping elements from the stack
while (!myStack.empty()) {
cout << "Top element: " << myStack.top() << endl;
myStack.pop();
}
return 0;
}
Explanation: This code snippet demonstrates how to create a stack, add (push) elements, and remove (pop) them using C++.
Real-Life Applications of Stack
Undo/Redo Functionality in Applications: Most text editors and design software use stacks to implement undo and redo features. Each user action is pushed onto a stack. When an undo is triggered, the last action is popped from the stack and reversed, allowing users to revert changes efficiently.
Call Stack in Programming: The call stack manages function calls and recursion in programming. Each time a function is invoked, it is pushed onto the call stack, and once the function completes, it is popped off. This mechanism ensures that function calls are executed in the correct order, maintaining the program's flow.
Web Browser Back Functionality: Browsers use a stack to manage the history of visited web pages. Each new page visited is pushed onto the stack. When the back button is clicked, the current page is popped off, and the user is taken to the previous page, providing a seamless navigation experience.
Expression Parsing and Evaluation: Compilers and calculators often use stacks for parsing and evaluating mathematical expressions. By pushing operands and operators onto a stack, expressions can be processed efficiently to produce results following the order of operations.
Plate Stacks in Cafeterias: A simple, relatable example is a stack of plates in a cafeteria. Plates are added one on top of the other, and when someone needs a plate, they take the top one first—illustrating the LIFO concept.
Why Stacks are Effective in Real-Life Applications
The stack in data structure is efficient due to its LIFO nature, making it ideal for tasks that require reversing actions or managing function calls. Stacks allow quick access and modification, ensuring operations are performed smoothly and in an organized manner.
Conclusion
The stack in data structure is not just a theoretical concept but has a broad range of real-life applications. Its efficiency and simplicity make it perfect for tasks that require a LIFO order. Understanding how stacks are used in programming and everyday scenarios can help appreciate their importance and implement them effectively in coding exercises.
FAQ:
What is the purpose of the stack?
A stack is used to store data in a Last In, First Out (LIFO) order. Its purpose is to manage tasks where the last item added needs to be accessed first, such as in function calls, parsing, and undo operations.
How does a stack work in programming?
What are common applications of a stack?
Stacks are used in function call management, expression evaluation, backtracking algorithms, and undo/redo features in software.
What are the main advantages of using a stack?
Read, Also - What is push and pop in stack?
CoreGamer is a news portal that will become your main source of information about the world of video games. We publish the latest news about new releases, updates on popular games, and important industry events on a daily basis. On CoreGamer https://coregamer.net/ you will find detailed reviews, professional analytical articles and exclusive interviews with developers. Immerse yourself in the world of gaming with us and stay up to date with all the latest developments!