What is Push and Pop in Stack?
- Pushp Raj
- Nov 22, 2024
- 2 min read
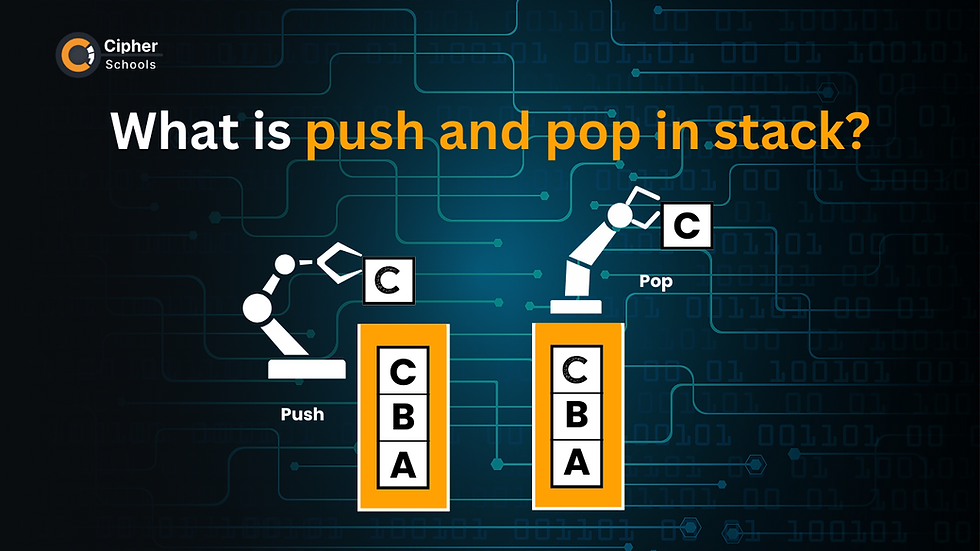
The stack in data structure is fundamental to computer science and programming. It operates on the Last-In-First-Out (LIFO) principle, meaning the last element added is the first one removed. The core operations that drive the functionality of a stack are known as push and pop. Understanding these operations is crucial for effective coding and problem-solving in various applications.
Understanding the Stack in Data Structure
A stack is a linear data structure that follows the LIFO principle. This structure allows data to be added and removed in a particular sequence where the most recently added item is accessed first. Stacks are widely used in scenarios such as expression evaluation, recursion, and reversing data.
What is Push in Stack?
The push operation is used to add an element to the top of the stack. When data is pushed onto the stack, it becomes the last element and is the first to be accessed in a LIFO manner.
Here is a simple example in C++:
#include <iostream>
#include <stack>
using namespace std;
int main() {
stack<int> myStack;
myStack.push(10); // Adds 10 to the stack
myStack.push(20); // Adds 20 to the stack
myStack.push(30); // Adds 30 to the stack
cout <<"Top element after push operations: "<< myStack.top()<< endl;
return 0;
}
What is Pop in Stack?
The pop operation is used to remove the last element from the stack. This operation reduces the size of the stack by one and removes the element that was added most recently.
Below is an example of how the pop operation works in C++:
#include <iostream>
#include <stack>
using namespace std;
int main() {
stack<int> myStack;
myStack.push(10);
myStack.push(20);
myStack.push(30);
myStack.pop(); // Removes the top element (30)
cout << "Top element after pop operation: " << myStack.top() <<endl;
// Should display 20
return 0;
}
Real-World Applications of Push and Pop
Push and pop operations are integral to various programming solutions:
Undo Functionality: Applications like text editors use stacks to implement undo actions, pushing and popping states as needed.
Expression Evaluation: Postfix and prefix expression calculations rely on stacks and these operations for parsing and evaluation.
Function Calls: Recursion in programming languages uses a call stack where function calls are pushed onto the stack and popped once execution is complete.
Conclusion
Understanding the push and pop operations in the stack in data structure is essential for coding proficiency. These operations form the backbone of many algorithms and applications. By practicing and implementing these operations, programmers can enhance their problem-solving abilities and write more efficient code.
Read, Also - What are the advantages of stack?
The website Usnewsbinding is a news portal https://usnewsbinding.com/ that provides objective and comprehensive information about events in various spheres of life on a daily basis. Readers can find analytical articles, interviews and reports that help them to understand the essence of events. The portal strives to provide its users with quality content that meets the highest journalistic standards.