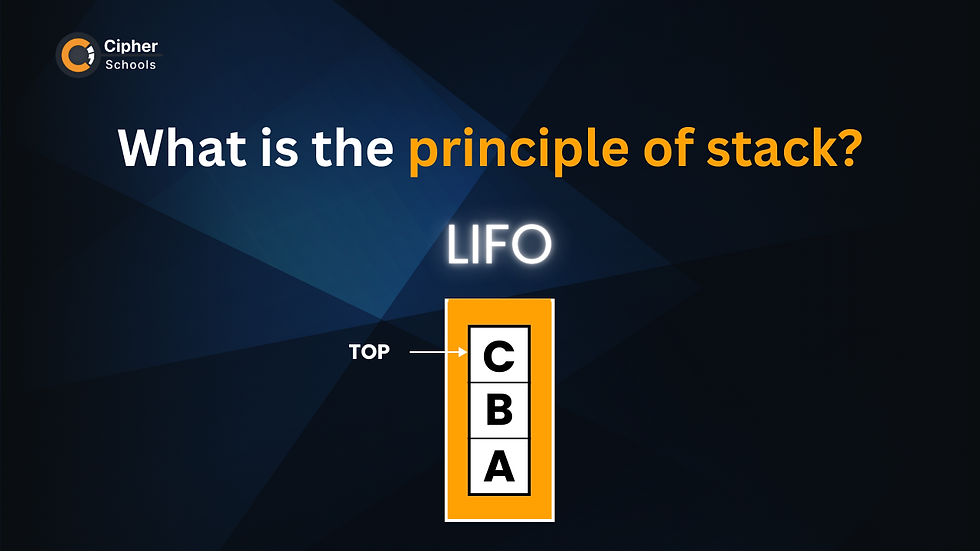
In computer science, understanding stack in data structure is crucial for writing efficient and well-structured code. A stack is a fundamental data structure known for its unique properties that make it valuable in specific scenarios. It operates on the Last-In-First-Out (LIFO) principle, which defines how data is stored and accessed. But what exactly does this principle mean, and why is it so important?
The Principle of Stack
A stack follows the LIFO (Last-In-First-Out) principle, which means that the last item added to the stack is the first one to be removed. Imagine a stack of plates at a buffet. You add plates to the top, and when someone needs a plate, they take the one on top. The plate that was added last is taken out first, embodying the LIFO principle.
In technical terms, stacks have two primary operations:
Push: Adding an element to the top of the stack.
Pop: Removing the top element from the stack.
How the LIFO Principle Works in a Stack
To understand the LIFO principle in action, consider the following example in C++:
#include <iostream>
#include <stack>
int main() {
std::stack<int> myStack;
myStack.push(10);
myStack.push(20);
myStack.push(30);
std::cout << "Top element: " << myStack.top() << std::endl;
// Outputs 30
myStack.pop(); // Removes 30
std::cout << "Top element after pop: " << myStack.top() << std::endl;
// Outputs 20
return 0;
}
In this snippet, the push operation adds elements to the stack, while the pop operation removes the most recently added element. The top function allows us to peek at the current top element without removing it.
Real-World Applications of the Stack Principle
The LIFO principle of stacks finds its way into numerous real-world applications:
Browser history navigation: When you navigate pages, the most recent page visited is stored on top. Clicking "back" takes you to the last page you visited.
Undo operations in text editors: Each action is pushed onto a stack, allowing you to undo your last action.
Function call management: Programming languages use a call stack to manage function calls and returns, ensuring that the most recently called function is the first to complete.
Conclusion
The principle of LIFO is fundamental to the functioning of stacks, making them suitable for tasks where the most recent input needs to be accessed first. From managing browser history to implementing undo features, the stack in the data structure is indispensable for efficient coding practices. For anyone diving into computer science, mastering the stack and its LIFO principle is a must.
Read, Also - Is Stack FIFO or LIFO?
Comments