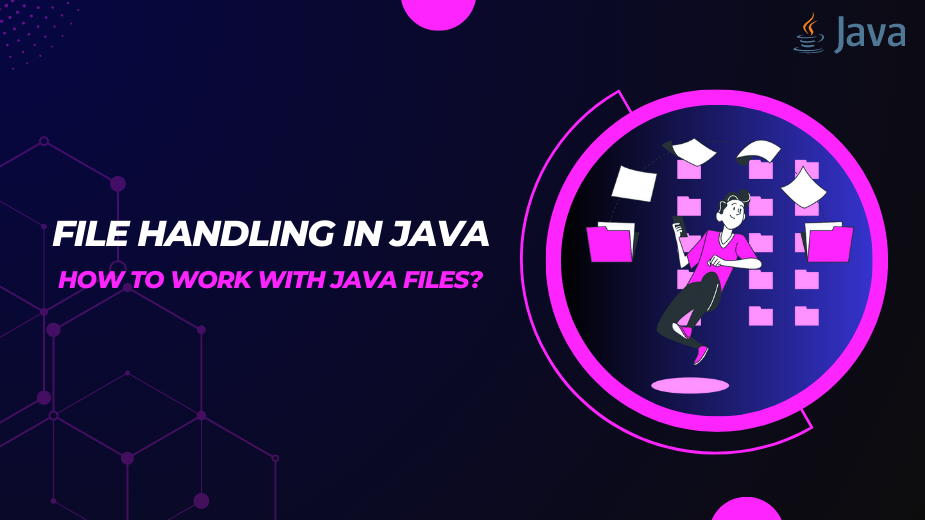
In Java, file handling is described as reading and writing data to a file. The file class from the java.io package enables us to handle and operate with various file types. If we wish to use a file class, we must first create an object of that type and supply the filename or directory name.
The java.io package's File class is used to conduct different operations on files and directories.
Another package that can be used to work with files is Java.nio. However, for the sake of this lesson, we will concentrate on the java.io package.
What are Directory and File in Java?
A file is a named location where relevant data can be stored. As an example, main.java is a Java file that includes program-specific information.
A directory is a grouping of files and folders. A subdirectory is a directory that exists within another directory.
Creating a Java File Object
To build a File object, we must first import the java.io.File package. Here's how we may construct file objects after we import the package.
// creates an object of File using the path
File file = new File(String pathName);
In this case, we've constructed a file object called File. The object supports working with files and directories.
Why is File Handling Necessary in Java?
File handling is an essential aspect of any programming language since it allows us to save the result of any program in a file and execute specific operations on it.
In lay terms, file handling entails reading and writing data to and from a file.
Example:
// Importing File Class
import java.io.File;
class GFG {
public static void main(String[] args)
{
// File name specified
File obj = new File("myfile.txt");
System.out.println("Hurrah File is Created!");
}
}
Output:
Hurrah File is Created!
In Java, the concept Stream is used to conduct File I/O operations. So, first and foremost, let us become acquainted with a Java notion called Stream.
In Java, streams are as follows:
A stream is a type of data sequence in Java.
This notion is used to perform File I/O operations.
Streams are classified into two types: Input and Output Streams.
Input and Output Streams in Java
An output stream writes data in or to a stream. The output stream can be sent to any device that can connect to a hard disc or to any stream that contains a sequence of bytes. An output stream can also be presented on any output screen that can do so. The command line receives stream output from your display.
When you begin writing for your display screen, it can only display the characters and not the graphical material. Graphical output necessitates more specialized assistance.
You read information from an input stream. In theory, this can be any source of serial data. However, it is most commonly a disc file, the keyboard, or a distant computer.
Furthermore, the file input and output you write in a machine can typically only be done through a Java application. It is only available to Java Apple in a restricted capacity.
This has two benefits. First, you don't have to worry about the intricate mechanics of each device, as these are handled behind the scenes.
The streams you create for input and output can only contain a limited amount of data, such as a single character, and no more. Transferring data to or from such a stream can be much more efficient. Hence, the Stream is frequently supplied with a buffer in memory, referred to as a buffered stream.
Input Stream
The superclass of all input streams is the Java InputStream class. The input stream reads data from various input devices such as the keyboard, network, etc. Because InputStream is an abstract class, it is useless on its own. Its subclasses, on the other hand, are used to read data.
The InputStream class has multiple subclasses, which are as follows:
AudioInputStream
ByteArrayInputStream
FileInputStream
FilterInputStream
StringBufferInputStream
ObjectInputStream
Syntax:
// Creating an InputStream in Java
InputStream obj = new FileInputStream();
InputStream Methods
Read(): One data byte is read from the input stream.
read(byte[] array)(): Reads a byte from the Stream and saves it in the array supplied.
mark(): It keeps track of the position in the input stream until the data is read.
available(): The number of bytes accessible in the input stream is returned.
markSupported(): It determines whether the stream supports the mark() and reset() methods.
reset(): Returns the control to the point in the Stream where the mark was set.
skips(): Removes and skips a specific amount of bytes from the input stream.
close(): The input stream is closed.
Output Stream
The output stream is used to write data to various output devices such as the display, File, etc. OutputStream is a superclass that abstractly represents an output stream. Because OutputStream is an abstract class, it is useless on its own. Its subclasses, on the other hand, are used to write data.
The OutputStream class has multiple subclasses, which are as follows:
ByteArrayOutputStream
FileOutputStream
StringBufferOutputStream
ObjectOutputStream
DataOutputStream
PrintStream
Syntax:
// Creating an OutputStream
OutputStream obj = new FileOutputStream();
OutputStream Methods
write(): Writes the supplied byte to the output stream.
write(byte[] array): Writes to the output stream the bytes contained within a given array.
close(): The output stream will get closed.
Flush(): Forces all data in an output stream to be sent to the destination.
There are two sorts of streams based on the data type:
Byte Stream
This Stream reads and writes byte data. The byte stream is further broken into two categories, as follows:
Byte Input Stream: This Stream reads byte data from various devices.
Byte Output Stream: Used to send byte data to various devices.
2. Character Stream
This Stream reads and writes character data. The character stream is further separated into two categories, which are as follows:
Character Input Stream: This Stream reads character data from various devices.
Character Output Stream: This Stream writes character data to various devices.
Now that you understand what a stream is, let's polish up File Handling in Java by learning more about the many valuable methods for performing actions on files such as generating, reading, and writing.
The Common/ Standard Streams in Java
Typically, your operating system will define three standard streams that are available via members of the system class in Java
The default input stream will correspond to the specific keyboard. This is wrapped in a system class member of the type input stream.
A Standard output stream will always conform to the output command line. The system class's out member wraps this and is of the type print stream.
A standard error output stream for error messages, which by default maps to command-line output. It is also surrounded by the err members of a class system and the print stream.
Everyday file handling operations in Java
In Java Common, file-handling operations include:
Creating a new file.
Writing in a file.
Reading an existing file.
Deleting a file.
To conduct any of the primary operations on a file in Java, we must first create an object of the File class, as seen in the code below:
import java.io.File; // Importing the File class
File obj = new File("filename.txt"); // Specifying the file name
Let's look at how we may do the above action on a file in Java.
createNewFile() - Use the createNewFile() function to create a new file. Consider the following example:
import java.io.File;
import java.io.FileWriter;
public class CWH {
public static void main(String[] args) {
File myFile = new File("CWH_file1.txt");
try {
myFile.createNewFile();
System.out.println("File created successfully in Java");
} catch (IOException e) {
e.printStackTrace();
}
}
}
Output:
File created successfully in Java
Writing in a File
The FileWriter class and its write() function are used to write data to a file.
When you're finished writing to a file, use the close() method. Consider the following example:
import java.io.File;
import java.io.FileWriter;
import java.io.IOException;
public class CWH {
public static void main(String[] args) {
File myFile = new File("CWH_file1.txt");
try {
FileWriter fileWriter = new FileWriter("CWH_file1.txt");
fileWriter.write("CipherSchools");
fileWriter.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
Output:
CipherSchools
Read a File
To read a file, the Scanner class is utilized.
To handle the IOException, the method must be enclosed in a try-catch block.
Example:
import java.io.File;
import java.io.FileNotFoundException;
import java.util.Scanner;
public class CWH {
public static void main(String[] args) {
File myFile = new File("CWH_file1.txt");
try {
Scanner sc = new Scanner(myFile);
while(sc.hasNextLine()){
String line = sc.nextLine();
System.out.println(line);
}
sc.close();
} catch (FileNotFoundException e) {
e.printStackTrace();
}
}
}
Output:
CipherSchools
Delete a File
In Java, the delete() function is used to delete a file.
Example:
import java.io.File;
import java.io.FileNotFoundException;
import java.util.Scanner;
public class CWH {
public static void main(String[] args) {
File myFile = new File("CWH_file1.txt");
if(myFile.delete()){
System.out.println("Now We have deleted: " + myFile.getName());
}
else{
System.out.println("Some problem occurred while deleting the file");
}
}
}
Output:
Now We have deleted: CWH_file1.txt
The Overall Code
package com.company;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileWriter;
import java.io.IOException;
import java.util.Scanner;
public class cwh_111_file {
public static void main(String[] args) {
//code to create a new file
/*
File myFile = new File("cwh111file.txt");
try {
myFile.createNewFile();
} catch (IOException e) {
System.out.println("Unable to create this file");
e.printStackTrace();
}
//code for writing to a file
try {
FileWriter fileWriter = new FileWriter("cwh111file.txt");
fileWriter.write("This is our first file from this java course\nOkay now bye");
fileWriter.close();
} catch (IOException e) {
e.printStackTrace();
}
// Reading a file
File myFile = new File("cwh111file.txt");
try {
Scanner sc = new Scanner(myFile);
while(sc.hasNextLine()){
String line = sc.nextLine();
System.out.println(line);
}
sc.close();
} catch (FileNotFoundException e) {
e.printStackTrace();
}
*/
// Deleting a file
File myFile = new File("cwh111file.txt");
if(myFile.delete()){
System.out.println("I have deleted: " + myFile.getName());
}
else{
System.out.println("Some problem occurred while deleting the file");
}
}
}
Features of File Handling in Java
File handling in Java is a vital capability that allows developers to conduct various file operations, such as reading, writing, creating, deleting, and changing files. Some of the most important aspects of file handling in Java are:
File objects: The File class in Java represents a file or directory on the file system. File objects can be used to execute actions such as determining the presence of a file or directory, obtaining file/directory attributes, creating/deleting files or directories, and exploring the file system.
Serialization: Object serialization is supported by Java, allowing objects to be written to and read from files as binary data. This capability allows developers to store and retrieve complicated data structures in files, such as objects and collections.
Directory Operations: Directory operations such as creating, removing, and browsing directories, as well as listing the contents of a directory, are supported by Java. This enables developers to perform file actions on entire directories, including traversing directory hierarchies recursively.
Platform Independence: Java's file-handling APIs are designed to be platform-agnostic, allowing for consistent file operations across operating systems such as Windows, Linux, and macOS.
Applications of File Handling in Java
In Java, File handling offers various uses in various software applications. Some of the most common file-handling apps in Java are:
File Input/Output: File handling in Java allows you to read data from and write data to files, making it useful for tasks like reading and writing configuration files, reading and parsing data from external files (like CSV or JSON files), and storing application data in files for long-term storage.
Database Operations: File handling in Java can be used to execute database file operations such as reading and writing data to and from database files, backing up or restoring database files, and importing/exporting data from and to database files.
Logging: In Java, file handling is widely used to log program events and failures to log files. Using Java's file-handling features, log files may be produced and managed, allowing developers to store and analyze logs for debugging, monitoring, and auditing.
Data Storage: Because Java allows for the storage and retrieval of data in files, it is helpful for applications that require persistent data storage, such as data caching, caching downloaded resources, or saving user preferences and settings.
Image/Video/Audio Processing: File handling in Java can be used to read and write multimedia files, such as images, videos, or audio files. This can benefit multimedia processing applications such as picture editing, video editing, and audio processing.
File System Operations: Various file system operations, such as creating, removing, renaming, and relocating files and directories, are supported by Java file handling. This is important in applications that manage files and directories, such as file explorers, backup tools, and file synchronization software.
Network Communications: File handling in Java can be utilized in network communications applications such as sending or receiving files over a network, downloading from the internet, or uploading files to a remote server.
These are only a few of the many applications of file management in Java. File handling is a versatile feature that gives developers significant capabilities for reading from and writing to files, making it a vital component of many software programs across several fields.
Conclusion
Finally, file handling in Java is a crucial concept for reading from and writing to files, both of which are critical for data storage and manipulation in many software applications. In its standard library, Java includes a complete set of classes and methods for file handling, making it simple to execute different file operations such as reading, writing, creating, deleting, and altering files.
We looked at the core principles of file handling in Java, such as working with file objects, reading and writing to text and binary files, using file streams and readers/writers, and dealing with exceptions that may arise during file operations. Advanced subjects covered included file serialization, random access file operations, and working with directories and file information.
In Java, file handling necessitates careful consideration of potential exceptions such as File not found, permission problems, and I/O faults. Proper error handling and exception management are essential to enable robust and dependable file operations in Java programs.
Furthermore, we addressed best practices for File handling in Java, such as appropriately closing file resources, leveraging buffered I/O for enhanced efficiency, and adhering to platform-independent file-handling strategies to ensure code portability across multiple operating systems.
Finally, file handling is integral to Java programming because it allows developers to read from and write to files fast and effectively. Developers may construct sophisticated programs that interface with files, store data persistently, and handle massive datasets with a strong understanding of Java's file-handling features.
Also Read: Basic Introduction to Java Programming
Comments