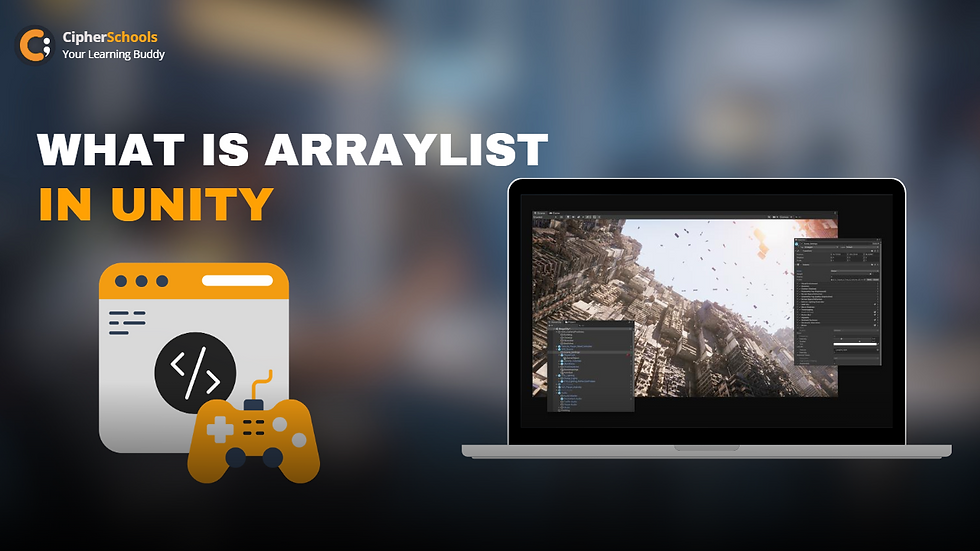
Lists, often arrays or collections, are essential in game creation. They are strong data structures that let developers efficiently organise and manage massive volumes of data. Lists provide a framework for managing game items, recording game information, and implementing complicated gameplay systems, whether you're making a basic 2D platformer or a considerable open-world RPG.
Lists serve several functions in game creation. They allow you to save and retrieve game objects like characters, goods, enemies, and missiles. Developers may efficiently cycle through these pieces, perform actions on them, and change their states as the game advances by using lists. Furthermore, lists make incorporating complex features like pathfinding algorithms, event triggers, and particle systems easier.
Keeping Items on the List
Using a List instead of an array can be much easier to work within a script. If you visit several C# and Unity forum sites, you'll notice that many programmers prefer a List instead of an array unless necessary. It is entirely dependent on the developer's preference and task. For the time being, let's keep to lists.
Here are the fundamental reasons why a List is superior to an array:
An array has a fixed size and cannot be changed.
A List's size can be changed.
A List element can be added and removed.
To simulate adding a new element to an array, we must first construct a new array with the necessary amount of items and then copy the previous elements.
The first thing to grasp is that, like an array, a List can store any object. Similarly to an array, we must indicate which type of item we want a specific List to contain. This means that if you want a List of integers of the int type, you can build a List that stores only integers of the int type.
Returning to the initial array example, let's store the same data in a List. To utilise a List in C#, add the following line at the start of your script:
using system.Collections.Generic;
The system is being used.Collections. As you can see, using Lists differs slightly from using arrays. The family members List is declared and assigned on line 9. There is a requirement for the type of items that will be stored in the list when declaring it. Simply type the type in the space between the< > characters. In this scenario, we're going to use string.
Instead of assigning elements in the declaration line, we must assign an empty List to be temporarily stored in the family member's variable because we will add the actual elements.
List operations that are commonly used
List<T> is straightforward to use. There is a long number of procedures that can be performed with it. We've already discussed inserting an element at the end of a List. Let's take a quick look at the most frequent ones that we might need later on:
Add: This function adds an object to the end of list <T>.
Remove: Remove a particular object's first instance from list <T>.
Clear: Clears all elements from list <T>.
Contains: This determines whether or not an element is in list <T>. Checking if an entry is stored in the list is quite helpful.
Insert: Insert an element at the provided index into list <T>.
ToArray: This function duplicates the elements of list <T> into a new array.
You don't have to grasp everything at this point. We want you to know that numerous out-of-the-box operations are available to you. We recommend searching for the list <T> class in the C# docs if you want to view them all.
Arrays vs list <T>
"Okay, which one should we use?" you're presumably thinking. There is no universal rule for this. Arrays and List<T> can both serve the same function. A wealth of supplementary material is available online to persuade you to choose one or the other.
In general, arrays are faster. We don't need to be concerned about processing speeds at this point. However, if your game slows down in the future, you may require more speed, so keep this in mind.
List<T> provides a lot of versatility. During declaration, you do not need to know the size of the list. The number has a large number of out-of-the-box procedures that you can utilise. Thus, that is my recommendation. The array is faster, while List<T> is more versatile.
Data retrieval from an Array or List<T>
Declaring and saving data in an array or list is now second nature. The next step is to learn how to retrieve stored elements from an array. Write an array variable name followed by square brackets to get a stored element from the array. Within the braces, you must enter an int value. This is referred to as an index. The index is merely the array's location. So, to get the first element in the array, we'll write the code below:
myArray[0];
Unity will return the data placed in myArray in the first place. It operates in the same manner as the return-type methods. So, if myArray stores a string value at index 0, that string will be returned to the location from whence you called it. Complex? It isn't. Let me give you an example.
The index value begins at 0, no 1; therefore, the first member in an array of ten items will be available via an index value of 0 and the last via a value of 9.
Examining the size
This is typical; we must check the array or list size. There is a subtle distinction between a C# array and a List<T>.
To get the size as an integer number, put the variable name, followed by a dot, and then the Length of an array or Count for List<T>:
arrayName.Length: This function produces an integer number indicating the size of the
array list.Name.Count: This function creates an integer indicating the size of the list.
Because we need to focus on one of the options here and move on, we'll use list <T> from now on.
What is an ArrayList
We certainly understand how to use lists now. We also understand how to create a new list and add, remove, and retrieve elements. You've also learnt that the data in list <T> must be of the same type across all components. Let's try something different.
ArrayList is essentially a List<T> without a data type that can be defined. This means that we can keep whatever we choose. It is also possible to store elements of various sorts. The ArrayList class is quite versatile.
Difference Between Arrays and Lists
Lists are similar to arrays but differ in a few ways. Lists, like arrays, can accept several items and build a container to hold them all. When we mention multiple types, you can select a list/array of ints, floats, or other types, but not both simultaneously.
One distinction between lists and arrays is that lists can be updated in real-time (in-game), whereas arrays cannot and have a fixed value. If you wanted to "update" an array, you would have to create a new array that was identical to the value of the old array while adding or subtracting what you wanted. It can be inconvenient.
Arrays are static storage, whereas lists are dynamic storage. The declaration of a list and an array varies somewhat in syntax.
Public List<GameObjects> listTest = new List<GameObject>();
Public GameObject[] arrayTest = new GameObject [15];
When declaring an array, we must provide its number of slots.
Arrays and lists are extensively used in game development to store and modify data sets. While they have certain commonalities, they also have considerable variances. In the context of game development, here are some significant distinctions between arrays and lists:
Size and adaptability:
Arrays have a fixed size that is determined during setup. The size of an array cannot be modified once it has been constructed unless a new array is allocated. When the amount of elements varies during gaming, this can be restricting.
Lists, however, are dynamic data structures that can be expanded or contracted as needed. They enable adding and removing pieces at runtime, giving games more flexibility in managing changing data requirements.
Memory Control:
Arrays typically utilise a fixed amount of memory that is allocated sequentially. This can benefit efficiency and performance, but it can also result in wasted RAM if the array is more extensive than necessary.
Lists employ dynamic memory allocation to consume only the memory required for their elements. When the collection size varies over time, this can be more memory-efficient.
Element Insertion and Access:
Elements in arrays are accessed and inserted using index-based referencing. Each element is given an index, beginning with zero, allowing immediate access to any element by giving its index.
Lists, on the other hand, often enable more flexible access and insertion options. Similar to arrays, entries in a list can be retrieved using indices. Still, lists also include extra techniques such as appending, inserting at a specific position, and removing components from anywhere in the list.
Performance:
Because of their continuous memory layout and consistent index-based referencing, arrays often provide incredible speed for direct element access. This is particularly important in real-time games that demand quick access to game objects or rapid iteration over vast databases.
Lists may operate slightly slower than arrays because they employ dynamic memory allocation and often involve additional overhead for handling the list's internal structure. However, the performance difference is frequently insignificant, and the flexibility and simplicity of lists can compensate for this disadvantage in many cases.
Operations and functionality:
Everyday operations supported by arrays and lists include iterating over elements, sorting, searching, and filtering. Lists, however, frequently include built-in functionality such as dynamic resizing, concatenation, sorting, and numerous ways to modify the collection. These features can eliminate the requirement for manual implementation and simplify game development duties.
Thus, arrays provide fixed-size memory allocation and direct index-based access, making them appropriate for situations where the collection size is known and constant. On the other hand, Lists offer dynamic memory allocation, flexible resizing, and extra convenience techniques, making them more suited for scenarios when the collection's size varies often.
Both arrays and lists have advantages and disadvantages and are utilised in game creation depending on the game's specific requirements and the nature of the data being maintained.
Real-Life Applications of Lists
Using lists in game creation has several real-world applications that improve gameplay, increase efficiency, and simplify content management. Here are some instances of list usage in game development:
· Game Object Management
Lists are frequently used in games to store and manage game objects such as characters, monsters, items, projectiles, and interactive elements. Developers may cycle through these objects, alter their states, and execute operations such as rendering, collision detection, and AI behaviour calculations by putting them in lists.
· Inventory and Item Management
Lists are pretty helpful when implementing inventory and item systems in games. The inventory is shown as a list of goods, allowing players to store, organise, and interact with the stuff they have collected. Lists make it simple to add and delete objects, manage numbers, sort by different properties, and perform operations such as equipping, using, and trading items.
Using lists in game creation has several real-world applications that improve gameplay, increase efficiency, and simplify content management. Here are some instances of list usage in game development:
· Game Object Management
Lists are frequently used in games to store and manage game objects such as characters, monsters, items, projectiles, and interactive elements. Developers may cycle through these objects, alter their states, and execute operations such as rendering, collision detection, and AI behaviour calculations by putting them in lists.
· Inventory and Item Management
Lists are pretty helpful when implementing inventory and item systems in games. The inventory is shown as a list of goods, allowing players to store, organise, and interact with the stuff they have collected. Lists make it simple to add and delete objects, manage numbers, sort by different properties, and perform operations such as equipping, using, and trading items.
· Design of the Level and Environment
In level and environment design, lists are frequently employed. They can represent tile maps, grid layouts, or other spatial data structures. Lists keep track of terrain types, object placements, collision zones, and interactive features, allowing for faster rendering, physics calculations, and interaction management.
· Leaderboards and high scores
Lists are used in games to construct high-score tables and leaderboards. Players' scores can be saved in a list, which can be sorted and updated as new entries arrive. Lists enable the presentation of top scores, the management of player ranks, and the facilitation of competition among players.
Conclusion
Finally, lists are essential tools in game creation, with a wide range of uses that improve gameplay, increase efficiency, and streamline content management. Lists give the flexibility, organisation, and data manipulation skills required for managing game objects, developing inventory systems, making quest logs, building levels, or aiding AI behaviour.
Because lists are dynamic, they can be added and removed at runtime to accommodate changing game conditions and player interactions. Because of this adaptability, developers may construct immersive and engaging experiences that respond to player choices and progress.
Game developers may efficiently store and manage vast amounts of data, optimise gaming systems, and add complicated features by harnessing the power of lists. Lists serve as the foundation for organising game materials, managing game states, and implementing complex gameplay mechanics, all of which contribute to the immersive and interactive experiences that keep players engaged.
Lists remain an essential and versatile data structure in the ever-changing game development field. Their apps cover various game development topics, allowing developers to create intriguing environments, engaging gameplay, and unforgettable player experiences.
Read, Also - How to use Arrays in Unity
Comments