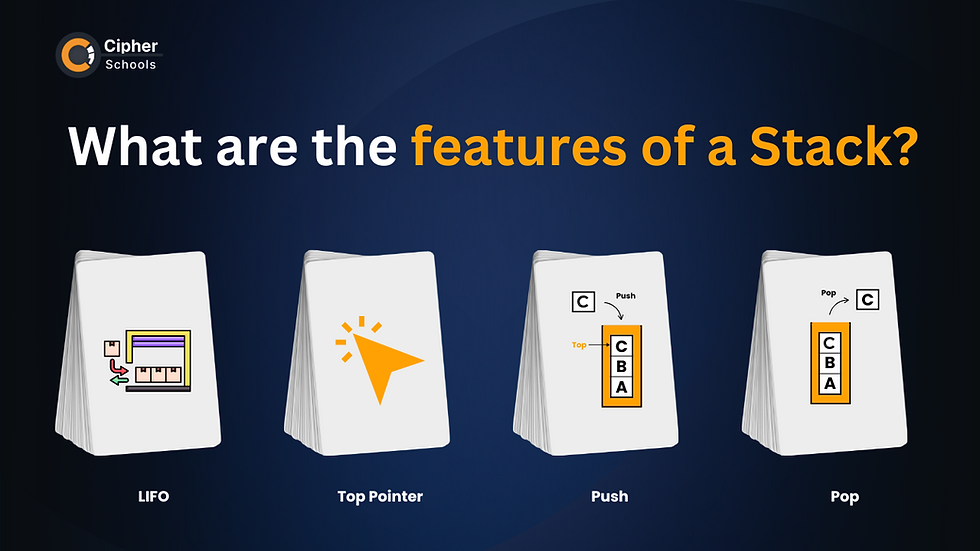
In programming, a stack is an essential data structure that organizes data based on the Last In, First Out (LIFO) principle. Understanding the stack’s features can significantly benefit developers when dealing with specific algorithms, memory management, and certain programming problems.
Key Features of Stack
1. LIFO (Last In, First Out) Principle
The defining feature of a stack is its LIFO structure. This means the last element added to the stack is the first one removed. Imagine a stack of books; the book placed last on top is the first to be removed. This principle is especially useful in applications like backtracking, where the last choice or step made is the first to be undone.
2. Push and Pop Operations
The push operation adds an item to the top of the stack, while pop removes the top item.
Here’s a small C++ snippet to illustrate these:
#include <iostream>
#include <stack>
using namespace std;
int main() {
stack<int> s;
s.push(10); // Push operation
s.push(20);
s.pop(); // Pop operation
cout << "Top element: " << s.top();
return 0;
}
In this example, after adding 10 and 20, the pop operation removes 20, demonstrating LIFO order.
3. Peek Operation
The peek operation, or top function in C++, allows you to view the item at the top of the stack without removing it. This is useful in scenarios where you only need to access the latest added element without altering the stack.
4. Fixed Size or Dynamic Implementation
Stacks can be implemented with a fixed size using arrays or dynamically sized using linked lists. A fixed-size stack is easier to implement but may suffer from overflow if it reaches capacity. In contrast, a dynamic stack grows as needed, allowing for more flexibility.
5. Overflow and Underflow Conditions
When working with stacks, overflow (trying to push onto a full stack) and underflow (trying to pop from an empty stack) are two common issues. Handling these errors is crucial for stable and secure programming.
Applications of Stack
Stacks are used in many areas, such as managing function calls in recursion, implementing undo/redo functionality, and evaluating mathematical expressions. Each application benefits from the stack’s unique structure and operational features.
Conclusion
The features of a stack in data structures make it a versatile tool for handling data that requires ordered access. Understanding these core principles will enhance your ability to work with algorithms and build efficient programs.
Read, Also - Types of Stack in Data Structure
Kommentare