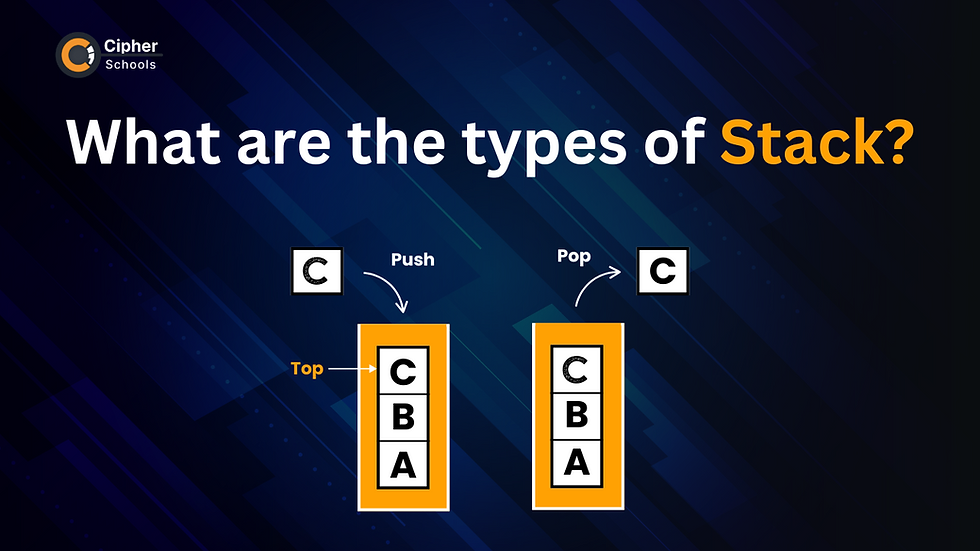
In data structures, a stack is a linear structure that operates on a Last In, First Out (LIFO) principle, meaning the last element added is the first to be removed. Stacks are widely used in algorithms and various applications due to their data handling efficiency and structured approach to managing tasks. Different types of stacks exist to fulfill specific requirements, offering added flexibility, efficiency, and memory management.
Simple Stack In Data Structure
A simple stack is the most fundamental type, where elements are added and removed from one end, typically called the "top" of the stack. In this stack type, only two operations are essential: push (to add elements) and pop (to remove elements). Simple stacks are useful in tasks that follow a strict order, such as evaluating mathematical expressions, managing function calls in recursion, and implementing undo operations in software.
Example in C++:
#include <iostream>
#include <stack>
using namespace std;
int main() {
stack<int> myStack;
myStack.push(10);
myStack.push(20);
cout << "Top element: " << myStack.top() << endl;
myStack.pop();
cout << "Top after pop: " << myStack.top() << endl;
return 0;
}
Doubly Ended Stack
A doubly-ended stack also called a deque (double-ended queue), allows for insertion and deletion at both ends of the stack. This stack type retains the LIFO property while allowing more flexible operations. Doubly-ended stacks are helpful in situations where elements need to be accessed and manipulated from both ends, such as managing windowed buffers or complex algorithmic processes. In addition to push and pop operations, these stacks support functions for pushing and popping elements from both ends.
Circular Stack
A circular stack is a variation where the last position connects back to the first, creating a circular loop. This approach allows for efficient memory use, as elements wrap around when they reach the end of the stack’s allocated memory. Circular stacks are ideal for applications requiring continuous, cyclical operations like buffering or round-robin task scheduling, where a finite amount of memory is reused cyclically.
Example in C++:
#include <iostream>
using namespace std;
class CircularStack {
int arr[5];
int top = -1;
int size = 5;
public:
void push(int val) {
top = (top + 1) % size;
arr[top] = val;
}
int pop() {
int val = arr[top];
top = (top - 1 + size) % size;
return val;
}
};
Application-Specific Stacks
Some stack variations are designed for particular applications:
Evaluation Stack: Utilized in evaluating expressions, this stack stores operands and operators, processing them in LIFO order to maintain proper precedence.
Call Stack: Common in programming languages, a call stack manages function calls and returns, ensuring functions execute in the correct sequence and retain necessary information for each call. It enables tracking of function states and local variables, making it indispensable for handling recursive calls and nested function calls.
Conclusion
The different types of stacks in data structure enable developers to solve a wide range of problems by optimizing how data is managed and accessed. Understanding and utilizing stack variations—simple, doubly-ended, circular, and application-specific—allows for more efficient, flexible solutions in programming, especially for algorithms, memory management, and process scheduling. Whether implementing a simple undo function or managing complex function calls, exploring stack types empowers developers to build more capable and efficient systems.
Read Also - What is stack and queue?
Comments