What are the advantages of stack?
- Pushp Raj
- Nov 20, 2024
- 2 min read
Updated: Dec 3, 2024
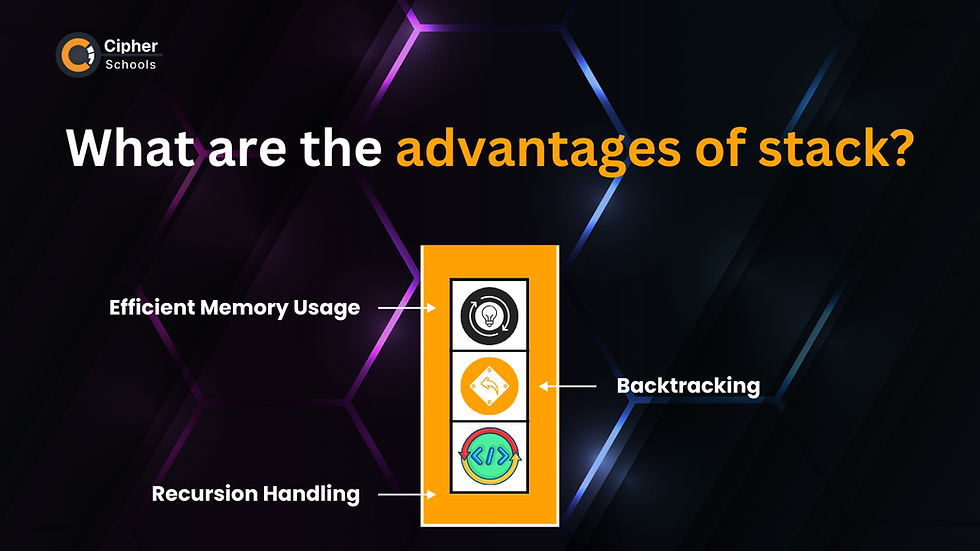
Introduction
In computer science, selecting the right data structure is vital for writing effective and well-organized code. The stack in the data structure is one such fundamental type that offers distinct advantages, making it suitable for a range of specific tasks. But what makes the stack so advantageous, and why should developers care?
Easy Memory Management
Stacks simplify memory management by adhering to the Last-In-First-Out (LIFO) principle. This means that the last element added is the first one removed, creating a predictable and straightforward way to manage data. When functions or procedures are executed, their information is pushed onto the stack and then popped off when completed. This automatic memory handling ensures that developers can efficiently track active data.
Efficient for Reversal Tasks
One of the most practical uses of stacks is for reversing data operations. For example, stacks are ideal for string reversal, undo features in applications, and checking if a word is a palindrome.
In C++, this can be demonstrated through a simple function:
#include <iostream>
#include <stack>
void reverseString(const std::string &input) {
std::stack<char> charStack;
for (char ch : input) {
charStack.push(ch);
}
while (!charStack.empty()) {
std::cout << charStack.top();
charStack.pop();
}
}
int main() {
std::string str = "World";
reverseString(str); // Output: dlroW
return 0;
}
Ideal for Recursion and Backtracking
Stacks are an integral part of recursion and backtracking algorithms. In recursion, the call stack stores information about the function calls, allowing the program to return to the previous state once the function completes. Similarly, in algorithms like maze solving or depth-first search, stacks help keep track of paths, making it easier to backtrack when needed.
Simplifies Expression Parsing
When it comes to evaluating mathematical expressions, especially in prefix or postfix notation, stacks are invaluable. They make it easy to convert infix expressions into a format that a computer can process and evaluate quickly. This capability is crucial in compilers and interpreters where expression parsing is a regular task.
Conclusion
The stack in data structure is essential for solving various computational problems due to its unique properties and advantages. From efficient memory management to facilitating recursive functions and expression parsing, stacks simplify numerous programming tasks. Exploring these applications will help developers appreciate the utility and versatility of stacks in real-world scenarios.
Read, Also - What is the principle of stack?
Comentários