Introduction to Variables and Data Types in Game Development
- Pushp Raj
- Jun 17, 2023
- 8 min read
Updated: Jan 22, 2024
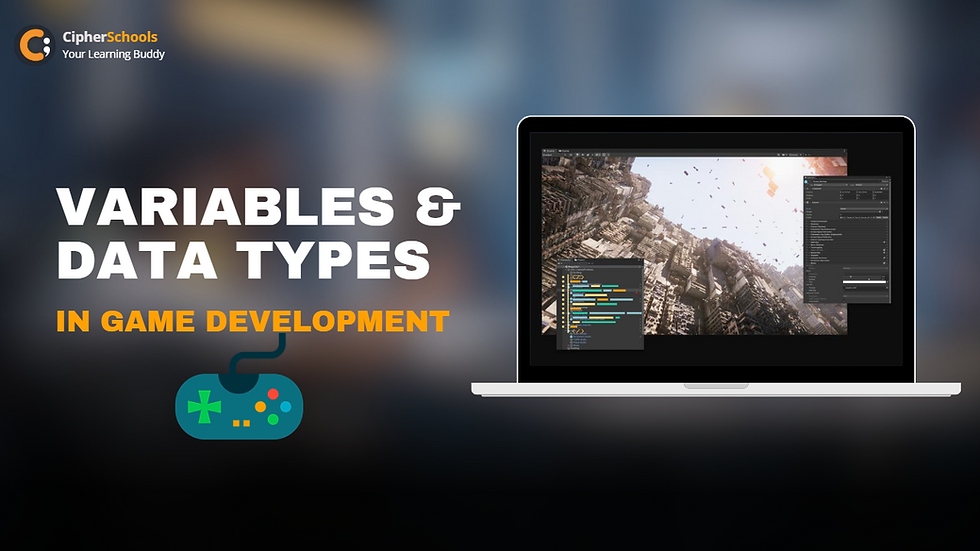
Data types are the building pieces that enable the construction of immersive and interactive experiences in the dynamic realm of game development. These data types are a foundation for organizing, storing, and manipulating data within a game. They are crucial in determining the appearance and behavior of game elements ranging from characters and objects to environments and game mechanisms.
Data types are distinct categories or classifications that define the nature of the data used in a game. They govern how data is kept in the game's memory, the actions that can be performed, and the range of values that can be represented. Understanding and utilizing the appropriate data types is critical for efficient data management and flawless gameplay as a game producer.
In game creation, numerous data types are often utilized. Let's have a look at them all.
What is a Data Type?
In software programming and game development, data type refers to a variable's value and the mathematical, relational, or logical operations that can be performed without generating an error. Many computer languages, for example, use the data type string to represent text, integer to represent whole numbers, and floating point to represent numbers with decimal points.
The data type specifies which actions can safely perform on the variable to generate, transform, and use it in another computation. Variable values in most programming languages have a static type. However, the importance of those static types can still reside within numerous variable classes. While some classes describe how the value of a data type will be compiled or interpreted, there are certain classes whose values are only annotated with their class during runtime.
Type safety refers to how much a programming language discourages or prevents type errors.
A computer language is said to be strongly typed when it requires a variable to be used only in ways that respect its data type. If datatypes do not align, such as when attempting to multiply an integer by a string, a highly typed language will most likely prevent the program from starting to avoid operational mistakes.
Read More: Introduction to Game Development
What exactly is a variable in programming?
So, after delving deep enough into the fundamental structure of a Unity C# script, we've arrived at the topic of today's article, Variables.
Variables can be considered Data Containers that will be utilized to hold data throughout your program. In your situation, the program refers to the game you're working on or a specific C# Script in your game's Unity Project.
Meanwhile, weakly typed programming languages allow a variable of one data type, which can be used as if it were a value of another data type. While the program may be entitled to execute, data discrepancies may result in errors or crashes.
Using An RPG Analogy to Explain Variable Use
Imagine you're creating an RPG (Role Playing Game).
In this RPG, your Player Character will have Stats such as Name, Strength, Endurance, Mana, Charm, and so on, which you will utilize to display and compute many things in your game.
Your game may include many goods and weapons, each with stats and perks.
When your game runs, all of these distinct stats must be stored somewhere; that location is variable.
How can You Declare a Variable and Assign It a Value?
Now that we've gotten to the coding section let's go over the syntax for declaring a variable and assigning a value to it.
The syntax for Declaring a variable can be separated into three sections, as illustrated in the image above.
Access Modifier
Data Type
Variable Name
Access Modifier
Access Modifier determines the visibility of your variable to other portions of your program. That is it, Itine, where you can access and edit the specified variable.
Defining the access modifier is deemed optional; however, it should be done every time because it makes your code more transparent.
If you do not define an access modifier, the variable will be private by default if it is a class member, i.e., part of a class.
In this article, pay attention to what a variable is and how you can utilize variables when creating a game with C# and the Unity Game Engine.
Data Type
The Data Type of your variable determines what kind of data you can put into the variable.
If you attempt to insert data into your variable that is incompatible with the Defined Data Type of your variable, you will receive an error.
For example, if you try to put text into a variable that is only supposed to hold Whole Numbers, you will get a compile-time error.
After we discuss discussing and final point, we will go over reviews of Data Types in C#.
Variable Name
Finally, there is the Variable Name. This is the name we will use to access this variable when we want to obtain the data stored inside it or when we want to set or edit the data contained inside it.
For example, if we want to access the Player Character Stats like Strength, Mana, Charm, and so on when the player opens his Stats Window, we will utilize the Variable Name in the code inside the C# Script to get the data stored inside this variable.
Finally, just as you would use a full stop (.) to end a sentence in English, whenever you write a statement in C#, such as when you declare a method, set a value to a variable, or do anything else in your code, you must end your line with a Semi-Colon (;), as shown in the image above.
Variables and Data Types in Game Development
Look at the various Data Types available when working in C#.
Boolean
A Boolean value can be true or false. This can be used to store data with only two sides.
Let's return to the RPG analogy for more context.
Consider this game a town with residences where players can enter and exit anytime, from 9 a.m. to 5 p.m.
To make this scenario function in your game, you'll need residences with open gates that close at the appropriate times.
A gate can now be opened or closed, which is ideal for using a Boolean. So, in your script, first declare a Boolean called _isGateOpen as follows:
private bool _isGateOpen;
After that, you can open the house doors by setting _isGateOpen to true in the script that manages the game world time and day-night cycle when the time reaches 9 a.m.
_isGateOpen = true;
You can close the house doors at 5 p.m. by setting _isGateOpen to false.
_isGateOpen = false;
However, simply changing the boolean values will not solve the problem because you must also code the game logic for what happens when _isGateOpen is set to true or false.
So, in the script that allows the player to enter and exit the house, you can use the _isGateOpen Boolean to establish a condition that enables the player to enter only if _isGateOpen is true and
prevents the player from entering if _isGateOpen is false.
There are other ways to make this a much more flexible system, but this is a basic example to demonstrate when you would use Booleans.
Byte, Short, Int, Long - Data Types
Consider the four Whole Number data types: Byte, Short, Int, and Long. The following four data types can be used to store whole numbers with different ranges.
byte : 0 to 255
short: -32768 to 32767
int : -2,147,483,648 to 2,147,483,647
long : -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807
This information may be found in the Spec Sheet image at the top of this section. The spec sheet also shows that each data kind will require a different amount of memory space.
byte: 1 Byte (8 Bits)
short: 2 Bytes (16 Bits)
int: 4 Bytes (32 Bits)
long: 8 Bytes (64 Bits)
You must ensure that the Data Type you are using for the variable has an acceptable range for the purpose for which it was designed.
To return to the RPG comparison, we discussed the need to save attribute data for your player character, such as Strength, Mana, Endurance, Charm, etc.
By doing so, we can now use all of these Data Types to store something similar.
private byte _strength = 9;
private short _endurance = 2;
private int _mana = 2;
private long _playerMoney = 3474674747738L; // L Suffixed
However, if you know that your character's Strength, Endurance, Mana, and so on will always be Positive Values, say, will never exceed 25. Using anything other than Byte data type to record this data would be a waste of memory.
Our goal with this lengthy discussion is to demonstrate how simple it is to use the Int data type to store whole numbers, which I do frequently.
Making a habit of only using what you need from the start will save you time and money in the long run and make you a better Game Programmer.
As you may have seen, we do not use any suffix when setting a value for Byte, Short, or Int data types, but you must suffix the value with the letter L when establishing a value for the
Long data type.
Sbyte, Ushort, Uint, Ulong – Data Types
Byte, Short, Integer, and LongWhen it comes to these four Whole Number Data Types, another idea needs to be taught before going on to Fractional data types, which is the concept of Signed and Unsigned.
So, if you create a variable with the Short, Int, or Long keywords, you will be constructing a Signed Variant of Short, Int, or Long because the ranges of these data types cover both negative and positive numbers.
If you know the variable you're generating will only have positive values, you can define it using Unsigned Variants.
The Unsigned Variants' ranges are as follows:
short : 0 to 65,535
uint : 0 to 4,294,967,295
ulong : 0 to 18,446,744,073,709,551,615
And you can declare them as follows
private short _weaponWeight = 35984;
private uint _playerMoney = 837333U; // U Suffixed
private ulong _maxXP = 78627691836748UL; // UL Suffixed
The opposite is true in the case of byte because byte is unsigned by default.
The Signed Variant of the Byte range
sbyte: -128 to 127
... you can declare a Signed Variant of Byte, i.e., byte, similarly.
private byte _charm = 6; // It is because Byte is intrinsically unsigned; there is a Signed Variant of Byte.
Another thing you may have noticed is that uint values must be suffixed with the letter U, whereas long values must be suffixed with UL.
Float, Double, Decimal – Data Types
The fractional data types, Float, Double, and Decimal, can hold Numbers with a floating point.
All three data types will hold fractional values in the ranges shown in the image of the Spec Sheet above. Although they appear to be very similar, there is a distinction between them that you should be aware of.
Because they store values as a Binary Floating Point Type, float and double data types are neither "decimally accurate" nor "decimally precise."
While decimal is "decimally accurate" and has substantially higher precision since it stores the numbers as a Decimal Floating Point Type, binary is not.
As a result, even if utilizing decimal data type would reduce performance, you should use it when performing crucial operations requiring precision, such as financial calculations.
You can do further research on that, but because you're reading this article on game creation,
We'll assume you're making a game; in this case, you don't need such high precision and can get by with float or double.
Returning to the code, you can declare these Fractional Data Types as follows.
private float _floatExample = 27.32f; // F Suffixed
private double _doubleExample = 4434049875.6533d; // D Suffixed
private decimal _decimalExample = 2.7325367890642635896m; // M Suffixed
Char, String – Data Types
Let's talk about the data types you'd use to store text-based data, w: Chard String.
The Char data type can be used to record a single character in the form of
Unicode – e.g., \u0046
Hex – e.g., \x0046
Just a character – e.g., b, *
A string is a sequence of characters that can store any text length with a theoretical upper limit of 2GB per string or around 1 billion characters.
You can declare and initialize char using single quotes in the code, as seen below.
private char _charExample = 'b';
Strings can be declared and initialized using double quotes, as shown below.
private string _stringExample = "This is your gaming host VP.";
Returning to the RPG model, you'll often use Strings to display Dialogues, Game Menus, and your HUD.
Any text in your game will use a string in some way or another.
Conclusion
Finally, data types are critical in game development since they serve as the foundation for organizing and managing information within a game. They allow creators to describe the traits, behavior, and structure of game elements, such as people and objects, as well as environments and game mechanics.
Developers can optimize memory use, improve performance, and create immersive and interactive game experiences by understanding and utilizing the proper data types.
Also Read: Core Loop in Game Development
Comentarios