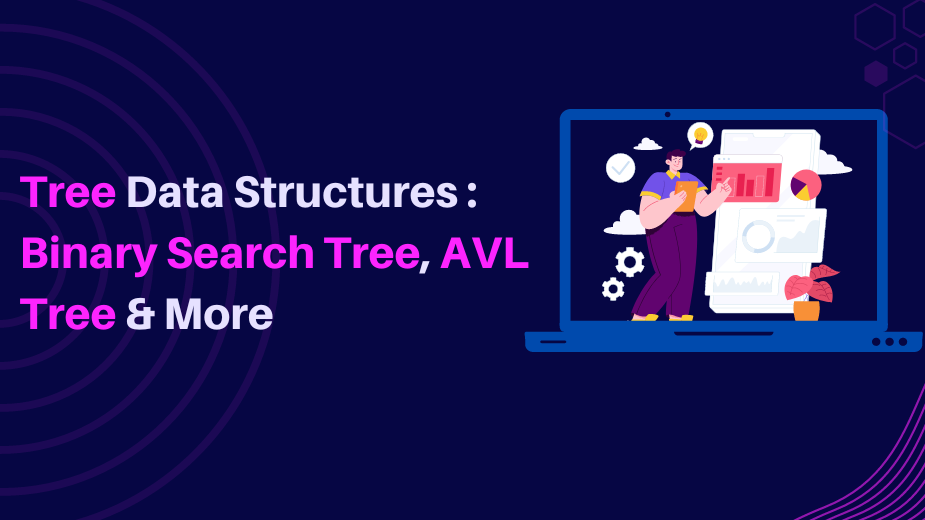
WHAT IS A TREE?
Trees are non-linear hierarchical data structures. A tree is a collection of bumps connected by directed or undirected" edges". One of the bumps is called the" root-knot", and the other bumps are called child bumps or splint bumps of the root
knot.
Unlike other data structures like an array, mound, line, and linked list, which are direct, a tree represents a hierarchical structure. The ordering information of a tree is insignificant. A tree contains bumps and two-pointers. These two pointers are the parent knot's left child and the right child.
Let us understand the terminologies of trees in depth.
Root: A tree's root is the tree's first knot with no parent knot. There's only one root-knot in every tree.
Parent knot: The knot, a precursor of a knot, is called the parent knot of that
knot.
Child knot: The knot which is the immediate successor of a knot is called the child knot of that knot. Stock Children of the same parent knots are called siblings.
Edge: The edge acts as a link between the parent knot and the child knot.
Leaf: A knot with no child is known as the splint knot. It's the last knot of the tree. There can be multiple splint bumps in a tree.
Subtree: The subtree of a knot is the tree considering that particular knot as the root-knot.
Depth: The depth of the knot is the distance from the root-knot to that particular knot.
Height: The height of the knot is the distance from that knot to the deepest knot of that subtree. Height of tree The Height of the tree is the maximum height of any knot. This is the same as the height of the root-knot.
Position: A position is the number of parent bumps corresponding to a given knot of the tree.
Degree of knot: The degree of a knot is the number of its children.
NULL: The number of NULL bumps in a Binary tree is (N+1), where N is the number of bumps in a Binary tree.
Trees are one of the most important parts of trees, in coding or in placement point of view. Leave no doubt clear with our best tutorials with cipher schools - Understanding tree in C++
Why Was Tree Data Structure Introduced?
One reason for using trees is to store information that naturally forms a scale. For illustration, the train system on a computer. Like Linked Lists and unlike Arrays, Trees don't have an upper limit on the number of bumps, as bumps are linked using pointers.
Trees (with some orders., BST) give moderate access/ hunt (quicker than Linked List and slower than arrays).
Trees give moderate insertion/ omission( quicker than Arrays and slower than Unordered Linked Lists).
What are the Major Usage Ranges of Tree Data Structures?
Converting and manipulating the hierarchical data. To make the information convenient to search (see tree traversal). To manage and manipulate sorted lists of data. As a workflow for combining digital images for visual goods. Router algorithms in the form of multi-stage decision-timber (see business chess).
Trees can be used to represent the structure of a judgment and can be used in parsing algorithms to dissect the alphabet of a judgment. Trees can represent the decision-making process of computer-controlled characters in games, similar to decision trees. Huffman's rendering uses a tree to represent the frequency of characters in a textbook, which can be used for data contraction.
Trees represent the syntax of a programming language and can be used in compiler design to check the syntax of a program and induce machine law.
Understanding The Types of Trees and Their Applications
Binary Tree
A binary tree is considered a java tree data structure where each knot can have at most two children, which are appertained to as the left and right child.
The top knot of a binary tree is called the root, and the bottom-most bumps are called leaves. A binary tree can be considered a hierarchical structure with the root at the top and the leaves at the bottom.
A Binary tree can be represented by a dot, a pointer to Left Chile, pointer to the right child.
Applications That Include Binary Tree
Syntax trees are used for utmost notorious compilers for programming like GCC and AOCL to perform:
computation operations.
For enforcing precedence ranges.
Used to find rudiments in lower time( Binary hunt tree) and used to enable fast memory allocation in computers.
Used to perform garbling and decrypting operations. Binary trees can organize and recoup information from large datasets, similar to reversed ones.
Indicator and k- d trees.
Binary trees can represent the decision-making process of computer-controlled characters in games, similar to decision trees.
Binary trees can be used to apply searching algorithms similar to those in binary hunt trees, which can be used to snappily find an element in a sorted list.
Binary trees can be used to apply sorting algorithms similar to the mound kind which uses a Binary mound to sort rudiments efficiently.
Binary Search Tree (BST)
A binary search tree is a data structure that snappily allows us to maintain a sorted list of figures.
It's called a Binary tree because each tree knot has outside of two children. It's called a search tree, as it can be equipped to search for the presence of a number in O( log( n)) time.
The parcels that separate a Binary hunt tree from a regular Binary tree are All bumps of the left subtree that are lower than the root-knot. All bumps of the right subtree are further than the root knot Both subtrees of each knot are also BSTs, i.e. they have the below two parcels.
The algorithm completely depends on the property of BST that if each left subtree has values below the root and each right subtree has values above the root, and still, we can say for sure that the value isn't in the right subtree then we need to only search in the left subtree, and if the value is situated above the root, we can say for sure that the value isn't in the left subtree and we need to only search in the right subtree if the value is below the root.
Also Read: Linear and Binary Search in Data Structure
Applications Of Binary Search Tree (BST)
In multilevel indexing in the database
For dynamic sorting
For managing virtual memory areas in the Unix kernel
Code To Insert an Element Into BST
struct node *insert(struct node *root, int val)
{
if(root == NULL) //if root = NULL or tree traversal reaches NULL
return getNewNode(val);
else if(root->key < val) // insert into right subtree
root->right = insert(root->right,val);
else if(root->key > val) // insert into left subtree
root->left = insert(root->left,val);
return root; // Return the original root after insertion
}
struct node *getNewNode(int val)
{
struct node *newNode = malloc(sizeof(struct node));
newNode->key = val;
newNode->left = NULL;
newNode->right = NULL;
return newNode;
}
AVL TREE
AVL tree is a tone-balancing Binary hunt tree in which each knot maintains redundant information called a balance factor whose value is either-1, 0 or 1. AVL tree was named after its innovator Georgy Adelson- Velsky and Landis.
The balance factor of a knot in an AVL tree is the difference between the height of the left subtree and that of the right subtree of that knot. Balance Factor = ( Height of Left Subtree- Height of Right Subtree) or( Height of Right Subtree- Height of Left Subtree)
The balance factor maintains the tone-balancing property of an AVL tree. The value of the balance factor should always be-1, 0, or 1.
Applications of AVL Tree
For the addition of large records in databases
For research in large databases
Compiler in C++
A syntax tree represents the abstract syntactic structure of source law written in a programming language. Each knot of the tree denotes a construct being in the source law.
A compiler constructs a syntax tree during the syntax analysis phase of the compiler. This tree checks if the correct syntax is followed when rendering a computer program.
Expression Trees are used in compilers, which is an application of binary trees.
Recursive Data Structure
The recursive data structure in which the tree is also known as a recursive data structure. A tree can be defined as recursively because the distinguished knot in a tree data structure is known as a root-knot.
A knot of the tree contains a link to all the roots of its subtrees. The left subtree is shown in the unheroic color below, and the right subtree is shown in red.
The left subtree can be further split into subtrees shown in three different colors. Recursion means reducing commodity in a tone-analogous manner. So, this recursive property of the tree data structure is enforced in colorful operations.
Disadvantages of Tree in C++
Slow performance in worst-case scripts in worst-case writing, a Binary tree can degenerate, meaning that each knot has only one child. In this case, search operations can degrade to O( n) time complexity, where n is the number of bumps in the tree.
Space inefficiency Binary trees can be space hamstrung compared to other data structures. Each knot requires two child pointers, which can be a significant quantum of memory outflow for large trees.
Limited structure Binary: Trees are limited to two child bumps per knot, which can limit their utility in certain operations. For illustration, if a tree requires further than two child bumps per knot, a different tree structure may be more suitable.
Complex balancing algorithms: To ensure that Binary trees remain balanced, similar to AVL trees and red-black trees, colorful balancing algorithms can be used.
These algorithms can be complex to apply and bear fresh outflow, making them less suitable for some operations.
Conclusion
Trees are an anon-linear hierarchical data structure that's used in numerous operations in the software field. Unlike direct data structures with only one way to cut the list, we can cut trees in various ways. We had a detailed study of traversal ways and the colorful types of trees in this environment.
B-tone-balancing trees are used for database indexing for effective information reclamation.
There are several kinds of trees in computer wisdom that can be used for multiple purposes.
Trees are one of the most important tools every inventor needs to know. This makes problems- working briskly and lightly, especially for issues dealing with scale.
Read, Also - Best Programming Languages To Learn
Comments