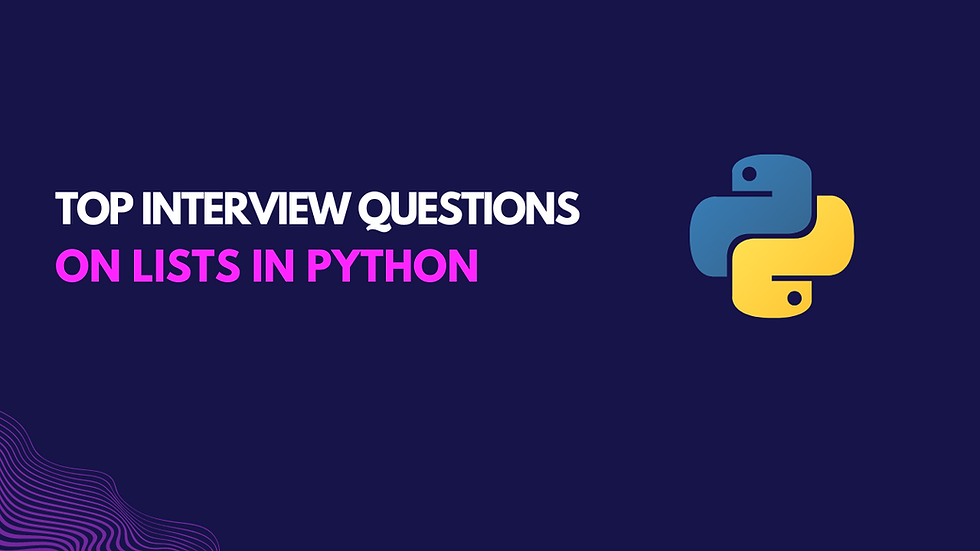
Introduction
In Python, a list is one of four built-in data structures, along with tuples, dictionaries, and sets. It is most likely that the items will be of the same type, but they might be of a different type as well. The purpose of them is to store ordered collections of items. A list is divided into elements by commas and enclosed in square brackets.
Python's lists store ordered collections of items, so we can call them sequence types, since they behave like sequences. Objects such as strings and tuples are also considered sequence types.
You might wonder what makes sequence types so special. In plain English, it means the program will iterate over them! In this sense, lists, strings, tuples, and sets are iterable.
You might see this concept returning in other programming languages that are used in data science, such as Scala.
Check out this basics of python course before starting this lists interview questions to get more deeper understanding
It's time to get down to business! Let's examine the questions that you might have as a Python programmer about lists in your interviews.
Top Interview Questions about Python List
Question 1: Define Lists
Lists are data structures in Python that represent an ordered sequence of mutable elements. An item is an element or value contained in a list. A list is defined by values enclosed in square brackets [ ], just like strings are defined by characters between quotes.
Question 2: Is it possible to iterate over two or more lists simultaneously?
Yes, it is possible by using zip(). Zip objects are iterators of tuples.
In the following code, we iterate over three lists simultaneously and interpolate their values into a string.
list1 = [1, 2,3,4]
list2 = [5,6,7,8]
list3 = [9,10,11,12]
z = zip(list1, list2, list3)
for list1,list2,list3 in z:
print(list1, list2, list3)
Output:
1 5 9
2 6 10
3 7 11
4 8 12
Question 3: If you are given a list of characters how would you convert it into a string??
In Python, you can accomplish this by calling a standard function:
list = ["h", "e", "l", "l", "o"]
r = "".join(list)
print(r)
Output:
hello
Question 4: How to test whether a list is empty or not
The length of a list is all that is needed to determine if it is empty. An empty list has a length of zero. Be sure to check that the list exists before checking its length to determine if it is empty, otherwise you may receive an error:
empty_list = []
non_empty_list = [10, 20, 30]
def check_empty(l):
if list:
print("The list is empty" if len(l) == 0 else "The list not empty"_)
Output:
check_empty(empty_list)
# List is empty
check_empty(non_empty_list)
# List is not empty
Question 5: What is the use of the “del” command
The del command removes an item from a list based on its index. We're going to remove the value at index 2 here.
list = [10,11,12,13,14,15,16]
del list[2]
print(list)
Output:
[10, 11, 13, 14, 15, 16]
del doesn't return the removed element. We have to print the list to make sure the element is removed
Question 6: Can a list be mutated?
YES, they are mutable. Notice how the 2 indexed element's values can be changed in the code below.
list = ['a', 'b', 'c', 'd']
list[2] = "Hello"
print(list)
Output:
['a', 'b', 'Hello', 'd']
Question 7: Are List homogeneous?
Yes, they are. It is possible to mix different types of objects in a list
list = [1,'hello',1.0,3,4]
Question 8: If you are provided with a comma-separated list how would you convert it into a list?
It's a great interview question since real-world programming often involves splitting strings into substrings. Any string can be easily split with the split function by using a delimiter:
string = "Hello World, I am Muthu "
str_list = string.split()
print(str_list)
Output:
['Hello', 'World,', 'I', 'am', 'Muthu']
Question 9: Can you explain the difference between append and extend?
An object is added to the end of a list by using .append().
a = [4,5,6]
a.append(7)
print(a)
Output:
[4, 5, 6, 7]
Note that appending a list adds the whole list, rather than appending individual values
a = [4,5,6]
a.append([7,8])
print(a)
Output:
[4, 5, 6, [7,8]]
Each value from the second list is added as a separate element by .extend(). By extending one list with another, their values are combined.
b = [4,5,6]
b.extend([7,8])
print(b)
Output:
[4, 5, 6, 7,8]
Question 10: How to convert a list to a tuple
Using the tuple() function in Python, you can turn a list into a tuple. Give this function your list, and you'll get a tuple back!
Please note that Tuples are immutable and cannot be changed after they have been created!
list = ["Hello", "world"]
list_tuple = tuple(list)
print(list_tuple)
Output:
('Hello', 'world')
Question 11: Where would you use the list and dictionary
In general, lists and dictionaries have slightly different uses, but there are some overlaps.
For algorithm questions, you can use both but if you need speed you should go for the dictionary as lookups are faster
List:
Lists can be used to store the items in the order. An example of lists is shown below
list = [2,3,4,5]
As of Python 3.7, both lists and dictionaries are ordered, but lists allow duplicate values while dictionaries do not.
Dictionary:
If you want to count the number of times something appears, use a dictionary. Like the number of vehicles in a home.
vehicles = {'honda':2,'pulsar':1,'activa':5}
A dictionary can only contain one key per entry. Note that keys can also be in the form of tuples like the one shown below
{('b',2):3, ('c',3):5}
Question 12: How to calculate the size of a Python list
If you want to know how long your list is, you can use the len() function.
a = len([1, 2, 3])
print(a)
Output:
3
Question 13: How are lists and tuples different?
It is not possible to update a tuple after it has been created. A new tuple must be created in order to add, remove, or update an existing tuple. A list can be modified after it has been created.
A tuple represents an object as if it were a record loaded from a database, where the elements have different data types.
The most common use of lists is to store an ordered sequence of objects of a particular type (but not always).
The values in both are sequences and can be duplicated.
Question 14: How are lists and sets different?
The difference between a list and a set is that a list is ordered, while a set is not. Thus, list( set([4, 4, 3, 2]) ) loses its order when used to find unique values in a list.
The purpose of lists is to track order, while the purpose of sets is to track existence.
Sets are unique by definition, but lists allow duplicates.
Question 15: How does the list constructor create a copy from an existing list?
List constructors create shallow copies of lists passed in. However, using .copy() is less pythonic.
list1 = ['hello','world']
list2 = list(list1)
list2.append('how are you')
print(list1)
print(list2)
Output:
['hello', 'world']
['hello', 'world', 'how are you']
Question 16: How to use a reduction function to subtract values from the first element in a list
It is necessary to import functools in order to use reduce(). When a function is supplied to reduce, it iterates over a sequence and calls it on every element. The output of the previous element is passed as an argument.
from functools import reduce
def subtract(a,b):
return a – b
numbers = [110,20,15,11,12,8,15]
reduce(subtract, numbers)
Output:
reduce(subtract,numbers)
# 29
Above we subtracted 20,15,11,12,8,15 from 110
Question 17: What is the best way to iterate over both the values and the indices of a list
By passing a list to enumerate(), a counter is added to the list.
In the following code, we iterate over the list, passing both value and index into the string interpolation formula.
cars = ['honda','alto','BMW']
for index,value in enumerate(cars):
print("%s: %s" % (index, value))
Output:
0: honda
1: alto
2: BMW
Question 18: How to identify the first matching element by its index
If you are looking for the first "honda" and "bmw" in a list of cars, use .index().
cars = ['honda', 'BMW', 'Benz', 'Audi', 'BMW', 'honda']
cars.index('apple') #=> 2
cars.index('pear') #=> 0
Question 19: How to remove all the elements from a list
We can use .clear() to clear the elements from an existing list rather than creating a new empty list.
cars = ['honda', 'BMW', 'Benz', 'Audi', 'BMW', 'honda']
print( cars )
print( id(cars) ) cars.clear()print( cars )
print( id(cars) )
It is also possible to use del
cars = ['honda', 'BMW', 'Benz', 'Audi', 'BMW', 'honda']print( cars )
print( id(cars) )
del cars[:]print( cars )
print( id(cars) )
Read More: Most Popular Programming Languages in 2024
Question 20: How to multiply every element in a list by 6 with the map function
Using .map(), you can iterate over a sequence of values and update each with another function.
A map object is returned by map(), but I've wrapped it with a list comprehension to see the updated values.
def multiply_6(value):
return value * 6
list = [1,2,3,4,5]
[value for value in map(multiply_5, list)]
Question 21: How to identify the longest string in a list
Our goal is to find the longest string in a list of strings. Since we must check each string's length at least once, O(n) is the best time complexity we can hope for. Ask your interviewer how the algorithm should handle the case where there are multiple longest strings in the list. In order to simplify things, we will return the longest string.
Method 1: Track the longest string by looping through the list
The standard approach to iterating through a list is to use a for loop. Iterating through the list of strings with a for each loop is the best method for this problem since it eliminates indexes and results in more readable code that is less prone to indexing errors.
str_list = ["Hello", "world", "how", "are", "you", "feeling", "today"]
longest_string = ""
for current_str in str_list:
if len(current_str) > len(longest_string):
longest_string = current_str
print(longest_string)
Method 2: Use a functional approach to iterate through the list
For and while loops are commonly used programming constructs, but functional programming replaces them with functions. Often, this produces more readable and bug-free code. A functional approach can earn you major points if you demonstrate to the interviewer that you understand the trade-offs involved.
import functools
str_list = ["Hello", "world", "how", "are", "you", "feeling", "today"]
longest_string = functools.reduce(lambda longest, current_str:
current_str if len(current_str) > len(longest_string) else longest, str_list, "")
print(longest_string)
Question 22: How to test if the string contains a substring from the list
To solve this problem, we must check each string in a given list to see if it exists as a substring in another longer string.
Method 1: A simple iterative process
To check if a string exists in the larger string, iterate through the list and check if it exists:
word = ['hey', 'how']
contains = "Hey how are you"
not_contains = "this string does not contain the needed words"
def check_contains(str_list, larger_str):
for items in str_list:
if items in larger_str:
return True
return False
print(check_contains(word, contains))
print(check_contains(word, not_contains))
Iterating through both words in the list and words in the string exhaustively allows us to find all words in both lists and strings. The complexity of this process is O(n2). It is possible, however, to optimize this in order to achieve better performance in terms of time, as we will see in the next method.
Method 2: By calculating the set intersections
With two sets, we can reduce the time complexity of the algorithm by holding the words in a list in one, and the words in a larger string in another. The two strings can then be checked for intersection:
word = ['hey', 'how']
contains = "Hey how are you"
not_contains = "this string does not contain the needed words"
def check_contains(str_list, larger_str):
return len(set(str_list).intersection(larger_str.split())) > 0
print(check_contains(word, contains))
print(check_contains(word, not_contains))
While this algorithm uses less space to hold the words in sets than the previous algorithm, it has an improved time complexity of O(n) time.
Question 23: How to Remove Duplicates from the List
Several solutions exist to this problem, so let's examine the most common ones.
Method 1: By using the iteration method
There is no better solution than this. As we go through the list, let's make sure there are no duplicates:
list = [6,6, 2, 3, 4, 5]
no_duplicates_list = []
for num in number_list:
if num not in no_duplicates_list:
no_duplicates_list.append(num)
print(no_duplicates_list)
Method 2: By using the sets
A set prevents duplicates, so using one can simplify and make the solution more readable:
num_list = [6,6, 2, 3, 4, 5]
no_duplicates_list = list(set(num_list))
print(no_duplicates_list)
If you are provided with a list of user inputs how would you find the maximum and minimum numbers from that list. We can use the following approach to find the min and max numbers
list = []
number_of_items = int(input('Input the number of items in the list '))
for numbers in range(num):
items = int(input('The entered number is'))
listlang.append(items)
print('The entered list =',list)
print("Max number = :", max(list), "\nMin number :", min(list))
Output:
Enter the number of items in the list 4
The entered number is 25
The entered number is 35
The entered number is 80
The entered number is 70
The entered number is 90
The entered list = [25, 35, 80, 70,90]
Max number = : 90
Min number : 25
Read More: Best books to read as a programmer
Conclusion
The purpose of this article is to show you common interview questions for a Python developer about lists. With these questions and regular practice sessions, you'll be able to crack any Python-based interview. Python has gained a lot of popularity over the years because of its simplicity and ability to perform powerful computations. As a result, Python developers are in high demand. It is worth mentioning, however, that being a Python developer comes with many perks. The emphasis is not only on theoretical knowledge of Python but also on the ability to write good quality code. If you learn and practice problems, you will be able to crack any interview without a doubt.
Check out the free courses offered by Cipher Schools exclusively for professionals if you are interested and upskill yourselves
Comentários