Java Class and Objects (With Example)
- Pushp Raj
- May 8, 2023
- 9 min read
Updated: May 26, 2023
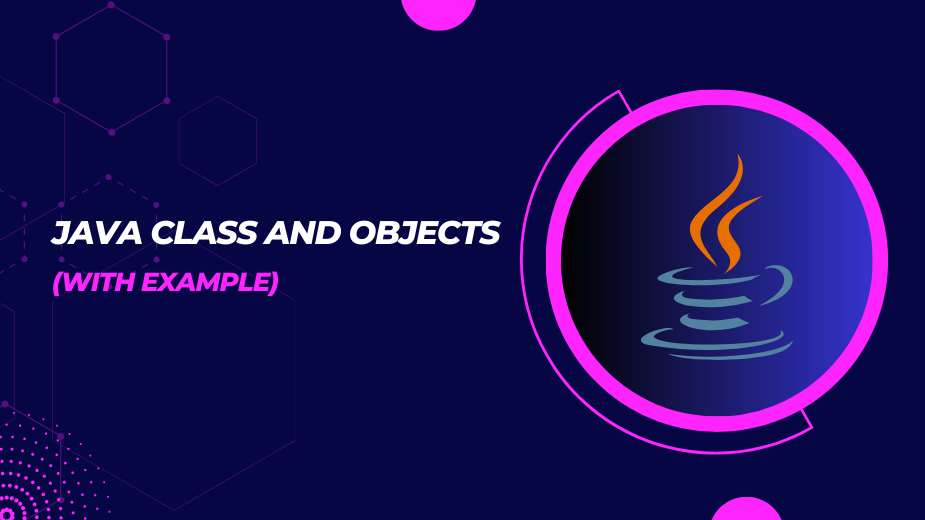
Classes and objects are the two most important Java notions or concepts that any programmer should understand. Classes and objects are inextricably linked and collaborate. An object is a class instance with behaviors and states. A cat, for example, is an object—it is color and size state, while its meowing and scratching furniture are behaviors. A class is a blueprint or template that outlines the state or behavior that objects of that kind support.
Let's look at Java classes and objects, beginning with the class.
What Exactly Is a Class in Java?
A class can be defined as a container that holds data members and methods. All objects share these data elements and methods in a given package. The syntax for declaring Class in Java:
class <class_name>{
field;
method;
}
Every Java class has the following components, which are outlined below in this article.
Access Modifiers in Java
Object-oriented programming languages like Java give programmers four types of access modifiers.
Public
Private
Protected
Default
These access modifiers define the accessibility and user permissions of the class's methods and members.
1. Class Name
This describes the name assigned to the class by the programmer per the preset naming rules.
2. Class Body
The class's body is mainly made up of executable statements.
Aside from these, a class may add keywords such as "super" if a superclass is used.
Use " implements if you're inheriting members, methods, and instances from the same class, use "implements."
If you are inheriting any members, methods, or instances from another class, use "interface."
Types of Classes
In Java, classes are classified into two types:
Built-in Classes
Built-in classes are the predefined classes included with the Java Development Kit. (JDK). These built-in classes are also referred to as libraries. Here are some instances of built-in classes:
java.lang.System
java.util.Date
java.util.ArrayList
java.lang.Thread
2. User-defined Classes
Self-explanatory are user-defined classes. The name is self-explanatory. They are real-time programming classes that the user defines and manipulates. User-defined classes are classified into three types:
A. Concrete Class
Concrete classes are standard classes in which the user defines and stores methods and data members.
Syntax:
class con{
//class body;
}
B. Abstract Class
Abstract classes in java are comparable to concrete classes, except that they must be defined with the "abstract" keyword. If you want to instantiate an abstract class, it must have an abstract method. It can only be inherited otherwise.
Syntax:
abstract class AbstClas{
//method();
abstract void demo();
}
C. Interfaces
Interfaces are analogous to classes. The distinction is that a class represents an object's attitudes and behaviours, whereas an interface contains the behaviours that a class implements. These interfaces will only include the method signatures and not the actual method.
Syntax:
public interface demo{
public void signature1();
public void signature2();
}
public class demo2 implements demo{
public void signature1(){
//implementation;
}
public void signature2(){
//implementation;
}
}
In Java, you may define a typical class using the following syntax:
<Access Specifier> class <class name>{
//Body
}
Example:
public class Student{
String Name;
int rollno;
String section;
void study() {
}
void write() {
}
void play() {
}
}
Class Creation Guidelines
When working with Java classes, the following rules must be followed:
To declare a class, use the term "class."
Every class name should begin with an upper-case character, and if you intend to include more than one word in a class name, use camel case.
A Java project can include an unlimited number of default classes but no more than one public class.
When naming classes, you should avoid using special characters.
Multiple interfaces can be implemented by writing their names before the class, separated by commas.
A Java class should only inherit from one parent class.
What exactly is an object in Java?
An object is a class instance. In OOPS, an object is a self-contained component with methods and properties that make a specific data type useful, such as color name, table, bag, and barking. When you send a message to an object, you invoke or execute one of the methods described in the class.
An object in OOPS might be a data structure, a variable, or a function from a programming standpoint. It has a memory area set aside for it. Java Objects are organized into class hierarchies.
In Java, an object is made up of the following elements:
Identity
Behaviour
State
A. Identity: This is the user-specified name that allows it to interact with other objects in the project.
Example:
Name of the employee
B. Behaviour
The method that you declare inside an object defines its behaviour. This method communicates with other objects in the project.
Example:
Resting, Working, Eating
C. State
The attributes mirrored by the parameters of other objects in the project are used to express the state of an object's parameters.
Example:
Department, Employee id, Designation
How Do You Make an Object in Java?
In Java, a class, as previously said, is a blueprint for any object. So, once you've declared the class, the only thing left to do is instantiate it. Creating an item consists of three stages. They are as follows:
Declaration
Instantiation
Initialization
Syntax:
ClassName ReferenceVariable = new ClassName();
Example:
public class Student {
public Student (String name) {
System.out.println("parameters sent are :" + name );
}
public static void main(String []args) {
Student mystudent = new Student( "Henry" );
}
}
Output:
Henry
3 Methods for Object Initialization
There are three ways to initialize an object in Java. Let us investigate them.
· By reference variable
· By method
· By constructor
A. Example of an Object and a Class: Initialization by Reference
Initializing an object entail storing data in it. Let us look at a simple example where we'll use a reference variable to initialize the object.
Example:
class Student{
int id;
String name;
}
class TestStudent2{
public static void main(String args[]){
Student s1=new Student();
s1.id=1909;
s1.name="Cipherschools";
System.out.println(s1.id+" "+s1.name);//printing members with a white space
}
}
Output:
1909 Cipherschools
Note: We can also build several objects and store data using reference variables.
B. Example of an Object and a Class: Method Initialization
In this example, we create two Student class objects and initialize their values using the insertRecord function. By running the displayInformation() method, we display the objects' status (data).
Example:
class Student{
int rollno;
String name;
void insertRecord(int r, String n){
rollno=r;
name=n;
}
void displayInformation(){System.out.println(rollno+" "+name);}
}
class TestStudent4{
public static void main(String args[]){
Student s1=new Student();
Student s2=new Student();
s1.insertRecord(101,"Geeta");
s2.insertRecord(102,"Kiran");
s1.displayInformation();
s2.displayInformation();
}
}
Output:
101 Geeta
102 Kiran
C. Example of an Object and a Class: Initialization via a Constructor
Let us look at an example where we're keeping employee records to comprehend this concept better.
class Employee{
int id;
String name;
float salary;
void insert(int i, String n, float s) {
id=i;
name=n;
salary=s;
}
void display(){System.out.println(id+" "+name+" "+salary);}
}
public class TestEmployee {
public static void main(String[] args) {
Employee e1=new Employee();
Employee e2=new Employee();
Employee e3=new Employee();
e1.insert(01,"Ajay",5000);
e2.insert(02,"Imran",5000);
e3.insert(03,"Nikita",5000);
e1.display();
e2.display();
e3.display();
}
}
Output:
01 ajeet 5000
02 irfan 5000
03 nakul 5000
What are the many methods for creating an object in Java?
In Java, there are numerous methods for creating an object. They are as follows:
A. Anonymous Method
Anonymous means "without a name." An anonymous object does not have a reference. It can only be used when creating an item.
If you need to utilize an object once, an anonymous object is a smart choice. As an example:
new Calculation();//anonymous object
Calling method through a reference:
Calculation c=new Calculation();
c.fact(5);
Calling method through an anonymous object
new Calculation().fact(5);
Let's look at a complete example of an anonymous object in Java.
class Calculation{
void fact(int n){
int fact=1;
for(int i=1;i<=n;i++){
fact=fact*i;
}
System.out.println("The factorial value is "+fact);
}
public static void main(String args[]){
new Calculation().fact(5);//calling method with anonymous object
}
}
Output:
The factorial value is 120
B. Creating several objects with one type
As with primitives, we can only build numerous objects of the same type.
Primitive variable initialization:
int a=10, b=20;
Initialization of reference variables:
Rectangle r1=new Rectangle(), r2=new Rectangle();//creating two objects
Example:
class Rectangle{
int length;
int width;
void insert(int l,int w){
length=l;
width=w;
}
void calculateArea(){System.out.println(length*width);}
}
class TestRectangle2{
public static void main(String args[]){
Rectangle r1=new Rectangle(),r2=new Rectangle();//creating two objects
r1.insert(11,5);
r2.insert(3,15);
r1.calculateArea();
r2.calculateArea();
}
}
Output:
55
45
Example 2:
class Account{
int acc_no;
String name;
float amount;
//method to initialize an object
void insert(int a,String n,float amt){
acc_no=a;
name=n;
amount=amt;
}
//deposit method
void deposit(float amt){
amount=amount+amt;
System.out.println(amt+" deposited");
}
//withdraw method
void withdraw(float amt){
if(amount<amt){
System.out.println("Insufficient Balance");
}else{
amount=amount-amt;
System.out.println(amt+" withdrawn");
}
}
//method to check the balance of the account
void checkBalance(){System.out.println("Balance is: "+amount);}
//method to display the values of an object
void display(){System.out.println(acc_no+" "+name+" "+amount);}
}
//Creating a test class to deposit and withdraw an amount
class TestAccount{
public static void main(String[] args){
Account a1=new Account();
a1.insert(91166,"Vijay",5000);
a1.display();
a1.checkBalance();
a1.deposit(50000);
a1.checkBalance();
a1.withdraw(10000);
a1.checkBalance();
}}
Output:
91166 Vijay 5000.0
Balance is: 5000.0
50000.0 deposited
Balance is: 55000.0
10000.0 withdrawn
Balance is: 45000.0
Learning Java Classes and Objects with Real-life Examples
Consider building a pet management system designed just for cats. You will need information about the cats, such as their breed, age, size, etc.
It would be best to convert real-world beings, like cats, into software entities. Furthermore, the million-dollar question is how such software is designed.
Let us start with an exercise. The images below show three different breeds of cats.
Some distinctions you may have mentioned are breed, age, size, color, and so on. If you think about it, these differences are also standard features these cats share. These attributes (breed, age, size, and color) can be used to create data members for your object.
Next, list the common behaviours of these cats, such as sleeping, sitting, eating, and so on. As a result, these are the behaviours of our program/software objects.
So far, we have defined the following terms:
Class – cats
Data members or objects– size, age, color, breed, etc.
Methods– eat, sleep, sit, and run.
You will now obtain different dog objects in the Java class for different values of data members (breed size, age, and color).
Using this OOPs method, you can construct any program.
The following guidelines must be followed when establishing a class.
· The Single Responsibility Principle (SRP) states that a class should only have one reason to change.
· Open Closed Responsibility (OCP)- It should be able to extend any classes without affecting them.
· Liskov Substitution Responsibility (LSR) states that derived classes must be interchangeable with their base classes.
· The Dependency Inversion Principle (DIP) states that you should rely on abstraction rather than concretions.
· Interface Segregation Principle (ISP)- Create fine-grained client-specific interfaces.
Classes and Objects in Java Example
// Class Declaration
public class Cat{
// Instance Variables
String breed;
String size;
int age;
String color;
// method 1
public String getInfo() {
return ("The Breed is: "+breed+" Size is:"+size+" Age is:"+age+" color is: "+color);
}
public static void main(String[] args) {
Cat Persian = new Cat();
Persian.breed="Persian";
Persian.size="Small";
Persian.age=2;
Persian.color="white";
System.out.println(Persian.getInfo());
}
}
Output:
The breed is: Persian, Size is:Small Age is:2 color is: white.
Example of a Java Object and Class: main outside class
In the last application, we created the main() method within the class. Now we'll make some classes and define the main() method in another. This method is superior to the previous one.
Example:
// Class Declaration
class Cat {
// Instance Variables
String breed;
String size;
int age;
String color;
// method 1
public String getInfo() {
return ("The Breed is: "+breed+" Size is:"+size+" Age is:"+age+" color is: "+color);
}
}
public class Execute{
public static void main(String[] args) {
Cat Persian = new Cat();
Persian.breed="Persian";
Persian.size="Small";
Persian.age=2;
Persian.color="white";
System.out.println(Persian.getInfo());
}
}
Output:
The breed is: Persian Size is: Small Age is: 2 color is: white.
Advantages of Classes and Objects in Java
Objects in Java classes and objects have three key advantages:
Modularity: Objects can be disassembled and reassembled with one another; that is, a mental picture of an object can be modularized into multiple elements that can work together.
Abstraction: We think of a model-driven approach to problem-solving in object-oriented programming, which results in an abstract representation of the world where we can conveniently articulate our answers.
Reusability: Classes provide a prototype for the instantiated object; this prototype remains consistent across all class instances, eliminating the need to reimplement methods defined in an object. Furthermore, subclassing adapts the code of a superclass to another object.
Difference Between a Class and an Object
· In a program, a class is a template for constructing objects, whereas an object is an instance of a class.
· An object is a physical entity, but a class is a mental entity.
· A class does not allocate memory space; conversely, an object does.
· A class can only be declared once but can be used to create multiple objects.
· Classes cannot be altered, although objects can.
· Classes are devoid of values, whereas objects have their own.
· In Java, you can create a class using the "class" keyword, whereas you may create an object using the "new" keyword.
Conclusion
Finally, classes and objects are essential ideas in Java and object-oriented programming (OOP), allowing developers to design robust and adaptable software solutions. Classes define objects' structure, behavior, and state by acting as blueprints. In contrast, objects are instances of classes that represent real-world entities and may be manipulated to accomplish tasks or interact with other objects.
Encapsulation, inheritance, and polymorphism are all advantages of Java's implementation of classes and objects. Encapsulation is the ability to encapsulate data and behavior within a class, shielding it from unauthorized access and alteration. Inheritance creates new classes that inherit properties and methods from existing classes, encouraging code reuse and extensibility.
Polymorphism allows objects of different classes to be treated as if they were of the same type, increasing code flexibility and modularity.
Furthermore, classes and objects help construct modular and maintainable code by encouraging concern separation and functionality encapsulation. They also promote code reusability by allowing classes to be instantiated several times to produce objects with distinct states and behaviors, hence avoiding repetitive code.
To summarize, Java classes and objects are powerful tools that allow developers to design modular, flexible, and maintainable software solutions. They serve as the foundation for object-oriented programming, enabling the development of complex systems and applications.
Understanding and efficiently using classes and objects is vital for any Java developer, as is understanding these concepts for building efficient, scalable, and robust Java code.
Also Read - Arrays in Java with Example : Declare, Define
Comments