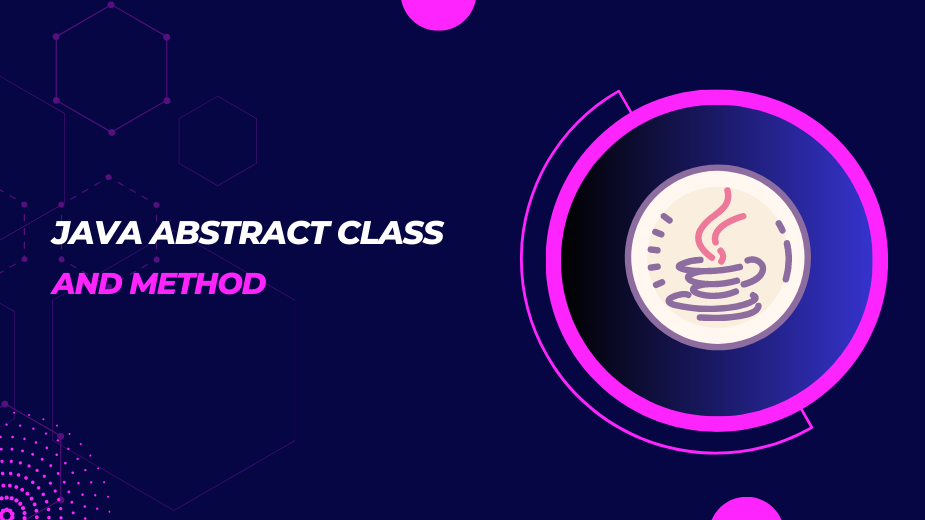
In Java, an Abstract Class hides the sophisticated code implementation details from the user and gives the user the essential information. In Object-Oriented Programming, this is known as Data Abstraction. (Java). Let's take a closer look at it.
What exactly is an Abstract Class in Java?
In Java, an abstract class is a template containing the data members and methods we utilize in our programs. In Java, abstraction prevents the user from seeing complex code implementations while providing the user with relevant information.
In Java, we cannot explicitly instantiate the abstract class. We can instead subclass the abstract class. When we utilize an abstract class as a subclass, the method implementation of the abstract class is made available to all of its parent classes.
Moving on, we shall discover all of the excellent advantages of the abstract class in Java.
Abstract Class Features
Template
The abstract class in Java allows the best technique to execute the data abstraction process by enabling developers to hide the code implementation. It also provides the end user with a template that describes the methods used.
Tight Coupling
Data abstraction in Java promotes loose coupling by exponentially reducing dependencies.
Reusability of Code
In the code, using an abstract class saves time. We can invoke the abstract method wherever it is required. The abstract class eliminates the need to rewrite the same code.
Abstraction
Data abstraction in Java allows developers to hide code complexities from the end user by reducing the project's total characteristics to simply the necessary components.
Resolution in Motion
Using dynamic method resolution support, developers can handle various problems with a single abstract method.
Before we proceed, let's first learn how to declare an abstract class.
Abstract Class Syntax
//Syntax:
<Access_Modifier> abstract class <Class_Name> {
//Data_Members;
//Statements;
//Methods;
}
Abstract Class Program in Java
package abstraction;
public abstract class Person {
private String Name;
private String Gender;
public Person(String nm, String Gen) {
this.Name = nm;
this.Gender = Gen;
}
public abstract void work();
@Override
public String toString() {
return "Name=" + this.Name + "::Gender=" + this.Gender;
}
public void changeName(String newName) {
this.Name = newName;
}
public void Exam() {
// TODO Auto-generated method stub
}
public void Office() {
// TODO Auto-generated method stub
}
}
package abstraction;
public class Employee extends Person {
private int EmpId;
public Employee(String EmployeeName, String Gen, int EmployeeID) {
super(EmployeeName, Gen);
this.EmpId = EmployeeID;
}
public void Office() {
if (EmpId == 0) {
System.out.println("Employee Logged Out");
} else {
System.out.println("Employee Logged In");
}
}
public static void main(String args[]) {
Person employee = new Employee("Cipher Schools", "Female", 100098);
employee.Office();
employee.changeName("Cipher Schools");
System.out.println(employee.toString());
}
@Override
public void work() {
// TODO Auto-generated method stub
}
}
//Output:
Employee Logged In
Name=CipherSchoolsGender=Female
There are some rules to follow while declaring an abstract class. Let us go over them in depth.
Rules for Declaring an Abstract Class in Java
The following are the main rules to remember when utilizing an abstract class in Java:
● When declaring an abstract class in Java, the keyword "abstract" is required.
● Abstract classes cannot be explicitly instantiated.
● There must be at least one abstract method in an abstract class.
● Final plans are included in an abstract class.
● Non-abstract methods may be included in an abstract class.
● Constructors and static methods can be found in an abstract class.
Abstraction in Java: A Step-by-Step Guide
In Java, data abstraction can be accomplished in two ways. The first method uses the abstract class in Java, and the second uses an interface.
Interface
In Java, an interface is a border between a method and the class that implements it. In Java, an interface contains the method signature but never the method implementation. The interface is used in Java to achieve abstraction.
//Syntax:
interface <Class_Name>{
//Method_Signatures;
}
//Example:
//Interface
package cipherschools;
public interface Area {
public void Square();
public void Circle();
public void Rectangle();
public void Triangle();
}
//Class
package cipherschools;
import java.util.Scanner;
public class shapeArea implements Area {
public void Circle() {
Scanner kb = new Scanner(System.in);
System.out.println("Enter the radius of the circle");
double r = kb.nextInt();
double areaOfCircle = 3.142 * r * r;
System.out.println("Area of the circle is" + areaOfCircle);
}
@Override
public void Square() {
// TODO Auto-generated method stub
Scanner kb2 = new Scanner(System.in);
System.out.println("Value of the side the square");
double s = kb2.nextInt();
double areaOfSquare = s * s;
System.out.println("Area of the square is" + areaOfSquare);
}
@Override
public void Rectangle() {
// TODO Auto-generated method stub
Scanner kb3 = new Scanner(System.in);
System.out.println("Enter the length of the Rectangle");
double l = kb3.nextInt();
System.out.println("Enter the breadth of the Rectangle");
double b = kb3.nextInt();
double areaOfRectangle = l * b;
System.out.println("Area of the Rectangle is" + areaOfRectangle);
}
@Override
public void Triangle() {
// TODO Auto-generated method stub
Scanner kb4 = new Scanner(System.in);
System.out.println("Enter the base of the Triangle");
double base = kb4.nextInt();
System.out.println("Enter the height of the Triangle");
double h = kb4.nextInt();
double areaOfTriangle = 0.5 * base * h;
System.out.println("Area of the Triangle is" + areaOfTriangle);
}
public static void main(String[] args) {
shapeArea geometry = new shapeArea();
geometry.Circle();
geometry.Square();
geometry.Rectangle();
geometry.Triangle();
}
}
//Output:
Enter the radius of the circle
5
Area of the circle is78.55
Enter the length of the side of the square
10
Area of the square is100.0
Enter the length of the Rectangle
25
Enter the breadth of the Rectangle
45
Area of the Rectangle is1125.0
Enter the base of the Triangle
20
Enter the height of the Triangle
25
Area of the Triangle is250.0
Benefits of Abstract Classes
● The abstract class in Java is quite useful for writing shorter code.
● Java abstraction prevents code duplication.
● Abstract classes allow for code reuse.
● Internal code implementation changes are made without affecting classes.
What is Interface in Java?
In Java, an interface is a class blueprint. It includes static constants as well as abstract methods.
In Java, an interface is a means for achieving abstraction. The Java interface can only have abstract methods, no method bodies. In Java, it is used to achieve abstraction and multiple inheritances.
In other words, interfaces can have abstract methods and variables. It is not allowed to have a method body.
Java Interface also represents the IS-A relationship.
● It, like the abstract class, cannot be instantiated.
● Secondly, we can have, by default and static methods in an interface with Java 8.
● We can have private methods in an interface with Java 9.
Why should you use a Java interface?
There are three key reasons to use an interface. They're listed below.
● Its purpose is to achieve abstraction.
● We can offer different inheritance functionality through an interface.
● It can be used for slack coupling.
How should an interface be declared in Java?
The interface is a keyword used to declare an interface. It supports complete abstraction, which implies that all methods in an interface are declared with an empty body by default, and all the fields are public, static, and final are at by default. A class that implements an interface must implement all of the interface's functions.
Syntax:
interface <interface_name>{
// declare constant fields
// declare methods that abstract
// by default.
}
Example of a Java Interface
The Printable interface in this Example contains only one method implemented in the A6 class.
interface printable{
void print();
}
class A6 implements printable{
public void print(){System.out.println("Hello");}
public static void main(String args[]){
A6 obj = new A6();
obj.print();
}
}
Nested Interface in Java
The Nesting Interface technique is used to overcome namespace concerns by grouping related interfaces or related interfaces with class.
As a result, this Example is based on nested interfaces. We are attempting to print the first 'n' prime numbers using interface layering.
//Interface Nesting
//Find the First 15 Prime numbers
package InnerInterface;
public interface InterfaceOuter {
void display();
interface InterfaceInner {
void InnerMethod();
}
}
//class
package InnerInterface;
import InnerInterface.InterfaceOuter.InterfaceInner;
public class NestedInterface implements InterfaceInner {
public void InnerMethod() {
int iteration = 0, num = 0, x = 1, y = 1;
while (num < 15) {
y = 1;
iteration = 0;
while (y <= x) {
if (x % y == 0)
iteration++;
y++;
}
if (iteration == 2) {
System.out.printf("%d ", x);
num++;
}
x++;
}
}
public static void main(String args[]) {
NestedInterface obj = new NestedInterface();
obj.InnerMethod();
}
}
//Expected Output:
2 3 5 7 11 13 17 19 23 29 31 37 41 43 47
The Benefits of Java Interfaces
The following are the benefits of using interfaces in Java:
● We can accomplish implementation security without worrying about the implementation component.
● Multiple inheritances are not permitted in Java; however, you can utilize an interface to take advantage of it because you can implement more than one interface.
What is the distinction between an abstract class and an interface?
Abstract classes and interfaces achieve abstraction by allowing us to declare abstract methods. Both the abstract class and the interface cannot be instantiated.
However, there are several distinctions between abstract classes and interfaces, which are listed below.
Abstract Class
Interface
An abstract class may have both abstract and non-abstract methods.
Interfaces can only have abstract methods. Since Java 8, it is also possible to have default and static methods.
The abstract class does not allow for multiple inheritances.
The interface allows for multiple inheritances.
Variables in an abstract class can be final, non-final, static, or non-static.
Only static and final variables are present in the interface.
An abstract class can provide the implementation of an interface.
An interface cannot provide the implementation of an abstract class.
To declare an abstract class, use the abstract keyword.
To declare an interface, use the interface keyword.
An abstract class can extend another Java class and implement various Java interfaces Only another Java interface can be extended by an interface.
The term "extends" can extend an abstract class.
The keyword "implements" can be used to implement an interface.
A Java abstract class may have class members such as private, protected, and so on.
Members of a Java interface are, by default, public.
Example:
public abstract class Shape{
public abstract void draw();
}
Example:
public interface Drawable{
void draw();
}
Nested Class in Java
A class in Java can be defined within another class, and such classes are referred to as nested classes. These classes assist you in logically grouping classes that are only used once. This improves the use of encapsulation and results in code that is more readable and manageable.
This blog section titled "Nested Class in Java" will concisely introduce nested classes in the Java programming language.
The class written within a class is referred to as the nested class, whereas the class that contains the inner class is referred to as the outer class. Here are some things to remember about nested classes in Java:
● A nested class's scope is defined by its enclosing class.
● A nested class gets access to the members of the parent class. However, the surrounding class cannot access the nested class's members.
● The enclosing class is a member of a nested class.
● A nested class's visibility can be public, private, protected, or package-private.
Classifications of nested classes
Inner/non-static nested class: nonstatic classes are a security measure in Java. A class cannot be connected with the private access modifier, but if the class is a member of another class, the non-static class can be declared private.
Inner-class subtypes
● Inner Class
● Method-local Inner Class
● Anonymous Inner Class
Inner Class
To construct an inner class, simply write a class within a class. An object outside the class cannot access a private inner class. The following is a program for developing an inner class. In this case, the inner class is made private and accessed via a method.
class Outer_Test {
int num;
//Inner class
private class Inner_Test {
public void print() {
System.out.println("This is an Our inner class");
}
}
// Accessing the inner class from the method
void display_Inner() {
Inner_Test inner = new Inner_Test();
inner.print();
}
}
public class My_class {
public static void main(String args[]) {
// Instantiating the outer class
Outer_Test outer = new Outer_Test();
// Accessing the display_Inner() method.
outer.display_Inner();
}
}
Method Local Inner Class
A class in Java can be written within a function and is a local type. The scope of an inner class, like that of local variables, is limited within the method. A method-local inner class is used solely within the method that defines the inner class. The program below demonstrates how to use a method-local inner class.
public class Outerclass {
// instance method of the outer class
void my_Method() {
int num = 1001;
// method-local inner class
class StarInner_Test {
public void print() {
System.out.println("This is star inner class
"+num);
}
} // end of inner class
// Accessing the inner class
StarInner_Test star = new StarInner_Test();
star.print();
}
public static void main(String args[]) {
Outerclass outer = new Outerclass();
outer.my_Method();
}
}
Anonyms Inner Class
An anonymous inner class has been declared without a class name. We declare and instantiate an anonymous inner class at the same time. They are typically used to override a class or interface method. The program below demonstrates how to use an anonymous inner class.
abstract class AnonymousInnerTest {
public abstract void mytest();
}
public class Outer_class {
public static void main(String args[]) {
AnonymousInnerTest inner = new AnonymousInnerTest() {
public void mytest() {
System.out.println("This is an example of anonymous
inner test class");
}
};
inner.mytest();
}
}
Static Nested Class
In Java basically, a static class is a nested class that has a static member that is a static member of the outer class. Unlike the inner class, the static nested class cannot access member variables of the outer class since it does not require an instance of the outer class. As a result, OuterClass. Does not refer to the outer class. A static nested class has the form
Static-Nested Class Syntax
class MyOuter {
static class Nested_Test {
}
}
Example of Static-Nested Class
public class Outer {
static class Nested_Test {
public void my_method() {
System.out.println("This is Cipher School’s nested test class");
}
}
public static void main(String args[]) {
Outer.Nested_Test nested = new Outer.Nested_Test();
nested.my_method();
}
}
The Distinction Between Static and Non-Static Nested Classes
Static nested classes have no direct access to other members of the enclosing class. Because it is static, it must use an object to access the non-static members of its surrounding class, which implies it cannot directly refer to the non-static elements of its enclosing class. Because of this limitation, static nested classes are rarely utilized.
Also, non-static nested classes have access to all members of their outer class and can directly reference them as other non-static members of the outer class can.
Important Points to Remember
● The inner class is regarded like any other class member.
● Because the inner class is a member of the outer class, you can apply access modifiers such as protected and private to your inner class.
● Because the Nested class is a member of its surrounding class, you can access the nested class and its members by using. (dot) notation.
● Using nested classes improves encapsulation and makes your code more readable.
● Even if they are designated private, the inner class has access to other members of the outer class.
Strings in Java
A String in Java is essentially an object that represents a sequence of char values. An array of characters functions similarly to a Java string. As an example:
char[] ch={'c','i','p','h','e','r','s','h','o','o','l','s',};
String s=new String(ch); is the same as:
String s="cipherschools";
The Java String class includes many methods for performing string operations such as compare(), concat(), equals(), split(), length(), replace(), compareTo(), intern(), substring(), and so on. Serializable, Comparable, and CharSequence interfaces are implemented by the Java.lang.String class.
A String is a character sequence in general. In Java, however, a string is an object that represents a series of characters. The class java.lang.A string object is created by using the String class.
We'll talk about immutable strings later. Let us first define String in Java and how to create a String object.
How do you make a string object?
String objects can be created in two ways:
● Using a string literal
● By using a new keyword
String Literal
Double quotes are used to construct a Java String literal. As an example:
String s="hello";
When you create or build a string literal, the JVM first examines the "string constant pool." A pointer to the pooled instance is returned if the string is already in the pool. If the string does not already exist in the pool, a new string instance is generated and added. As an example:
String s1="hello";
String s2="hello";//It doesn't create a new instance
Only one object will be produced in the above Example. To begin with, the JVM will not locate any string objects with the value "Welcome" in the constant string pool. Therefore, it will construct a new object. After that, it will look in the pool for the string with the value "Welcome," Instead of creating a new object, it will return a reference to the same instance.
To improve Java's memory efficiency (because no new objects are created if it exists already in the constant string pool).
With New Keyword
String s=new String("hello");//creates two objects and one reference variable
In this example, the JVM will build a new string object in regular (non-pool) heap memory, and the literal "Welcome" will be added to the constant string pool. The variables will correspond to the heap object. (non-pool).
Java String Example
public class StringExample{
public static void main(String args[]){
String s1="java";//creat string by Java string literal
char ch[]={'s','t','r','i','n','g','s'};
String s2=new String(ch);//convert char array to string
String s3=new String("example");//create Java string by new keyword
System.out.println(s1);
System.out.println(s2);
System.out.println(s3);
}}
Conclusion
Finally, Abstract Classes and Interfaces are strong Java tools that enable developers to design blueprints for classes and enforce specific behaviors. Interfaces define a set of methods that must be implemented by classes that implement the interface, whereas Abstract Classes give a framework for a group of related classes. In Java, both can be used to produce abstraction, polymorphism, and encapsulation.
On the other hand, classes defined within another class are known as nested classes. They can be static or non-static and can be used to group related classes together or grant private members of the outer class access.
Strings, which represent a sequence of characters, are a fundamental data type in Java. They are immutable, meaning their value cannot be modified once generated. As a result, they can be used for a wide range of activities, from representing user input to creating complicated data structures.
These four principles are critical components of Java programming, and understanding and implementing them can significantly improve the usefulness and efficiency of Java programs and this will help you clear your Java interview.
Comments