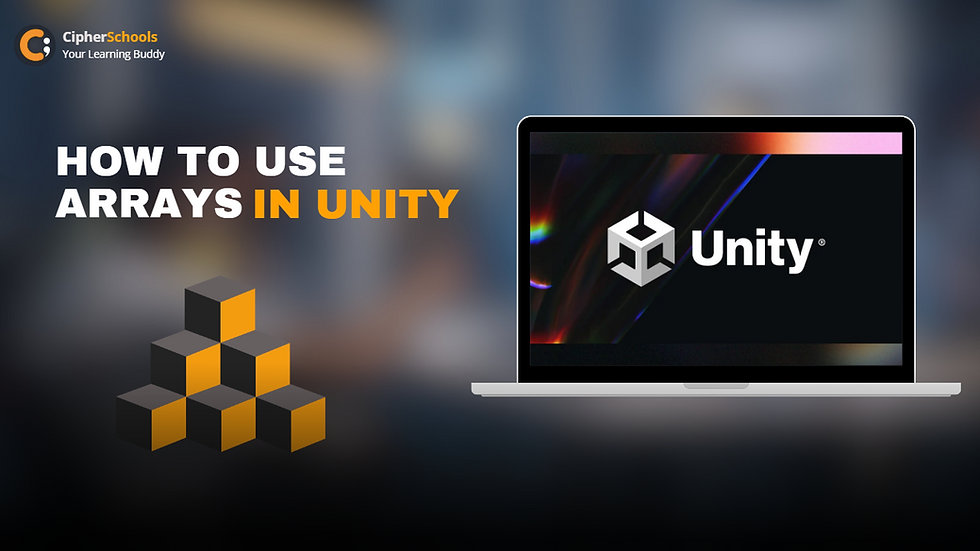
An array is one of the essential components of programming, and you should learn about them and understand how they work before beginning to design your games.
So, in today's article, we'll walk you through everything related to Arrays and all the concepts associated with them using real-world RPG analogies.
We will go over everything regarding arrays starting with:
What exactly is an array?
Why do we employ arrays?
Overwriting / Modifying a value at an Array Index Syntax for Defining and Initialising an Array in C#
determining the size of an array
Common Array Error | Index Out Of Range Exception and much more
So, let's get right to it.
What exactly is an array?
The previous post discussed variables and the various data types needed when programming in C#.
Check out our Variables and Data Types article if you need to know a variable or want to improve your knowledge.
It would be pretty simple to comprehend what an array is based on that article.
You already know that every Variable has a Data Type and that a Variable is similar to a container that can hold a value of the stated Data Type.
private int _playerHP = 300;
For example, we declared and initialized a variable named _playerHP with the Data Type set to int in the preceding code.
As a result, the _playerHP variable can only contain whole numbers within the ranges permitted by the int Data Type.
Arrays can now be viewed as a crate in which these container-like variables with identical Data Types are stored, and that crate is then given a name.
What is the RPG analogy?
In the RPG analogy, consider the numerous NPCs in the game and the need to remember their names (NPC - Non-Player Characters).
Let's pretend you know nothing about Arrays and only know what a Variable is.
Then you'd probably declare and set the values for these Variables like follows.
private string _npc1 = "Thor";
private string _npc2 = "Nazos";
private string _npc3 = "Zknight";
...
And the list goes on.
And it's perfectly fine if you need to know what an array is. However, doing it this way is somewhat redundant.
Why Do We Make Use of Arrays in Game Development?
You will notice two things if you look at this code and utilize your intellect.
First, you will notice that all three variables include the names of NPCs in the game.
Second, all three variables have the same Data Type and string.
Arrays are an excellent approach to categorizing this data type, allowing us to eliminate many unneeded redundancy.
Using arrays makes it much easier to manage and access data as needed. So, to avoid redundancy, declare an array for this circumstance.
Array Syntax in C#
private string[] _npcNames = new string[3]{"Thor", "Nazos", "Zknight"};
The line of code above may have made perfect sense to some of you.
While some of you are probably wondering, VP, what kind of chimera horror have you made?
So, let's disassemble this little beast and figure out the syntax.
Left Side of Array Defining Syntax in C#
private string[] _npcNames = new string[3]{"Thor", "Nazos", "Zknight"};
The first, as you well know, is the Access Modifier, which is private. The Variable's Data Type, which is a string, is next.
However, as you can see, we have a pair of opening and closing Square Brackets immediately following the Data Type.
This combination of opening and closing Square Brackets indicates we are constructing an Array.
And the Data Type preceding the Square Brackets will be the Data Type of this declared Array, which is _npcNames.
Right Side of Array Defining Syntax in C#
private string[] _npcNames = new string[3]{"Thor", "Nazos", "Zknight"};
Next is = new string[3,] which essentially says, Create a new string array with three elements at indexes 0, 1, and 2.
You can replace the three inside the square brackets, which represent the array's size, with any whole number.
In place of 3, you can even use a variable with a whole number datatype, such as byte, short, int, or long. Then we have everything inside the Curly Braces, which is us assigning values to our array.
Thor has an index of zero, Nazos has an index of one, and Zknight has an index of two.
Remember that an array and most things in programming begin at 0 and not 1. That is, the first member of an Array, in this example, Thor, is put at the _npcNames Array's 0th position rather than the 1st index.
Alternative Method to Defining An Array in C#
private string[] _npcNames = new string[3]{"Thor," "Nazos," "Knight"};
We declared and initialized the array in a single line of code in this piece of code. However, most of the time, you will not do things this way.
Instead, you'll divide the Array Declaration and Initialization into many phases, as seen above.
// All The Code Below Is Inside The Class
// Array Declaration.
private string[] _townLocations;
void Start()
{
int totalLocationsInGame = 2;
// Array Initialization.
_townLocations = new string[totalLocationsInGame];
_townLocations[0] = "Safe Cave";
_townLocations[1] = "President’s House";
// Logging Array Index Values In The Unity Console.
Debug.Log("Index 0 : " + _townLocations[0]);
Debug.Log("Index 1 : " + _townLocations[1]);
}
As you can see in the code above, we declare the array, _townLocations, but we don't initialize it.
In the Start Method, we initialize _townLocations with an int variable totalLocationsInGame as an argument.
Then we set the Dragon Cave to the 0th index and the Mayor's House to the 1st index of the _townLocations array.
When dealing with Unity, we use the two Debug—logs to log anything on the Unity Console for testing and debugging.
We want to log the information in the _townLocations array.
at the 0th index, which is located at _townLocations[0]
and at the first index, _townLocations[1].
We anticipate that this piece of code will do the following:
When we compile and run it, it will display in the Unity Console; you will get the following:
Index 0 : Safe Cave
Index 1 : President's House
That means we've successfully declared, initialized, and assigned values to the _townLocations array.
Changing / Overwriting A Value At An Array Index
Returning to the code, you can overwrite a value at a specific index using the same syntax you used to set the value.
For example, if you wish to alter the 0th index, which now contains Dragon Cave, to Lake
House, you can do it as follows.
_townLocations[0] = "Boat House";
After that, you can debug.Like this, log its value in the Unity Console.
Debug.Log("Index 0 Changed To : " + _townLocations[0]);
So, if we add these two lines of code after the two Debug.Logs in the code image above; we should get this in the Unity Console.
Index 0 : Safe Cave
Index 1 : President's House
Index 0 Changed To : Boat House
Searching the size of an array
Now, let's look at how to determine the size of an array.
This is important to understand because you will use it frequently while working with various loops.
To discover the size of the _npcNames and _townLocations arrays and then log it in the unity console, type
Debug.Log("Total NPCs : " + _npcNames.Length);
Debug.Log("Total Town Locations : " + _townLocations.Length);
So you type the array's name, in this example, _npcNames & _townsLocation. Then you put length followed by a dot (.).
If we add these two lines to the existing code, we will see the following logs in the Unity Console:
Total NPCs : 3
Total Town Locations: 2
We set the lengths of both arrays to these values when we declared and initialized them.
Common Array Error | Index Out Of Range Exception
Moving on, there is one more item we want to mention before we close up our today's article, and that is one of the most common errors you will experience while working with arrays.
So, let's purposely produce an error by adding the line below to our code.
Debug.Log("Name of the Third Location : " + _townLocations[2]);
This line of code should throw an error because we declared that the _townLocations array had only two members when we initialized it.
Now we're attempting to access the second index, which is the third item of the array, but it doesn't exist. (As a reminder, the array index begins at 0.)
When we run this code in Unity, we will get a big red exclamation mark in the Unity Console.
The error will display IndexOutOfRangeException and provide additional information about where this problem originated.
Let us now explain what is going on. Here, attempting to read a value at an index more significant than the array's size minus one.
Then you'll get a runtime error claiming the index was outside the array's boundaries. Which is just your program saying, "Dude, where's the index? There ain't nothing there."
The length of the _townLocations array in our case is 2. So, you would get this error if you use a number greater than the array's size - 1, i.e., 2 - 1, which is 1.
These are known as Runtime Errors, which occur while the program operates.
The compiler does not catch them when you compile the code and will only be noticed when you run the program, and things start to go wrong.
To avoid receiving this error, we can only say that you are attempting to access something that does not exist, which indicates that you made a logical error when writing the code.
Just remember that an array begins at 0 and ends at -> size of the array - 1.
Arrays are used in software development to tackle a relatively complex but exciting problem.
Arrays are used in PHP, Python libraries, and Lua tables. While the names are different, the problem they solve is the same.
Consider yourself playing a video game with guns or food if you prefer nonviolent games like Animal Crossing or Cooking Mama. How do you keep track of all the objects in your game? And how do you keep track of the attributes of each object? For example, if the video game has weapons, how do you maintain track of any weapon images that occur onscreen?
This is the problem that arrays, libraries, and tables (and whatever else they may be called) are intended to solve. Arrays provide a consistent framework for retrieving data from computer memory.
If you are developing the game in PHP or Python, do not worry. We have covered this also for you.
Here are some examples of how arrays are utilized in various programming languages. These examples merely emphasize the importance of array structures. They do not contain detailed production-ready code.
· LUA
weapons = { } -- create the table to store data
weapons[rock] = 1 -- in this crude example, set rock's power to 1
weapons[paper] = 2 -- set paper power to 2
weapons[scissors] = 3 -- set scissors power to 3
weapons[axe] = 4 -- set axe power to 4
weapons[scimitar] = 0 -- set scimitar power to 0
print(weapons[axe]) -- retrieves power setting for the axe
To save complexity, in this example, we merely create the database and then utilize the names of the weapons as keys to acquire a value. The worth of any weapon is defined as its level of power. In the actual world, each weapon key would have a table including all of the weapon's attributes. This example also demonstrates how to construct a table line by line. Lua tables, like PHP and Python, can be created with a single long line of code.
This code also uses print() to display the findings on the screen. Instead, actual live code would assign the value of weapons[axe] to a variable so that you could compare the power levels of various weapons.
PHP
$arrWeapons = array[
"rock" => 3,
"paper" => 1,
"scissors" => 2,
"axe" => 3,
"scimitar" => 0,
];
var_dump($arrWeapons);
While PHP, like Lua, allows you to nest arrays within arrays, this example assigns one value to each key. Also, note using a single set of square brackets to store the entire list of keys and their corresponding values. And the => symbol is allocated to each value. Finally, the PHP var_dump function would return this array's complete set of keys and values.
Python
weapons = {'rock': 1, 'paper': 2, 'scissors': 3, 'axe': 4, 'scimitar': 0 }
weapons[axe]
A dictionary can be created in Python as a single list. A value is assigned to a key by inserting a colon (:) character between it and its value. If you use a command prompt to work with Python, the second line outputs the value of the Rock key to the screen.
Perks of Using Array in Game Development
Using arrays in game creation has various advantages:
Data Storage Efficiency
Arrays provide a continuous memory block for storing several elements of the same type. Arrays are often used in game development to store vast amounts of data, such as game object coordinates, 3D model vertices, or animation frames. This effective data storage enables quick access to and modification of game-related data.
Random Access
Array elements can be accessed at random using their index. This functionality is useful in games when you need to fast access certain elements, such as accessing a specific enemy in a list of foes or retrieving a particular tile in a tilemap. Random access reduces lookup times, improving performance.
Iteration and Looping
Arrays can be readily iterated using loops such as "for" or "for each." This makes it possible to perform operations on several items simultaneously. You can, for example, update the locations of all game objects in an array, do physics calculations on each element, or check for collisions with other objects.
Flexibility
Arrays can be dynamically resized or updated during runtime, providing more flexible data management. This capability is beneficial when the number of elements can change during gameplay, such as adding or deleting adversaries, bullets, or power-ups.
Data Organisation
Arrays aid in the organization of linked data. Arrays can be used to record character traits, inventory items, or level information. Arrays provide a logical framework that simplifies game development and makes data management and manipulation easier by grouping relevant data.
Speed Enhancement
When used appropriately, arrays can aid in game speed optimization. Arrays increase cache coherence and reduce memory access overhead by storing data in a compact, contiguous architecture. Minimizing cache misses and optimizing data processing can lead to enhanced speed.
Conclusion
Finally, using arrays in game creation has various advantages and benefits. Arrays provide efficient storage and access to game-related data, allowing for speedy information retrieval and manipulation.
They enable quick lookup of individual elements based on their index, facilitating random access. Because arrays are easily iterable, performing operations on several elements simultaneously is possible. They offer flexibility and dynamic size, allowing parts to be added or removed during runtime.
Arrays also help with data organization, code organization, and data management. Arrays can also help with performance optimization by boosting cache coherence and lowering memory access costs. Overall, arrays are an essential data structure in game development since they improve efficiency, performance, and code readability. Arrays are not only used in games; they are also used in data analysis, scientific computing, picture processing, and other real-life settings.
Read, Also - Core Loop in Game Development
Comments