Learn JavaScript Function Generator
- preetikatiyar5
- Dec 8, 2022
- 4 min read
Updated: Jan 25, 2024
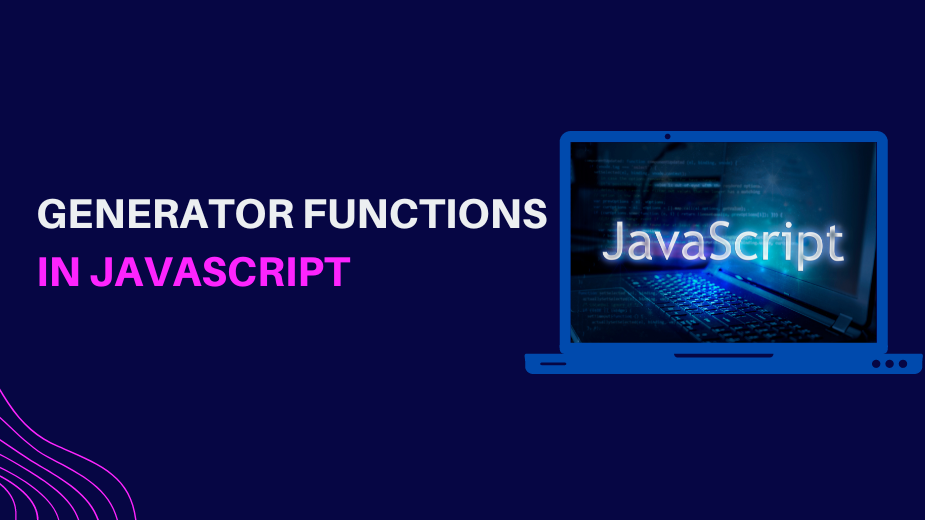
A generator function returns an iterator that can be used to stop the function in the middle, do something, and then resume at any time. A normal function starts executing and returns when the function completes, but a generator function can pause and resume later any number of times.
In JavaScript, generators offer a new way of working with functions and iterators.
“A generator is just a function that can stop in the middle of something and then start executing from the stop point.”
Using generator : -
1. You can stop function execution from anywhere inside the function
2. Resume code execution from where it was interrupted
SYNTAX –

What is yield ?
The yield keyword pauses the execution of the generator function and returns the value of the expression that comes after it to the generator's caller. It can be compared to the return keyword's generator-based equivalent.
Only the generator function that contains yield can call it directly. It cannot be called from call-backs or nested functions.
Generator-Object –
A generator function returns a generator object. A generator object is used by calling the next method on the generator object.
Generator objects are returned from generator functions and confirm to both iterable and iterator protocols.
Example –
Code
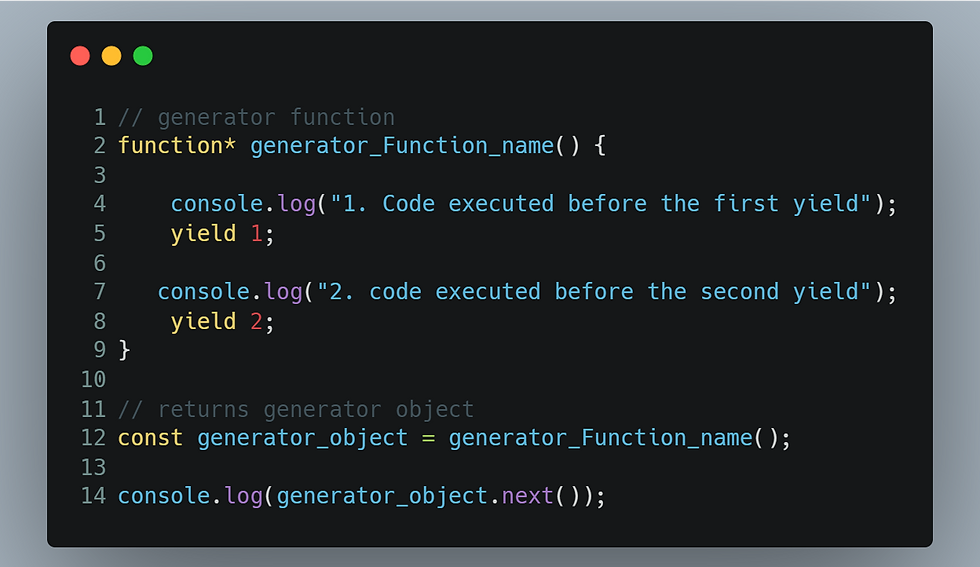
Output
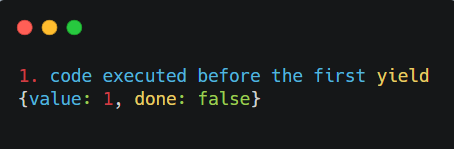
Let’s understand in detail :
The asterisk (*) after the function keyword (Line number 2), it denotes that the generator_Function_name() is a generator not a normal function.
A generator object named generator_object is created.
When generator_object.next() is called, the code up to the first yield is executed. When yield is encountered, the program returns the value and pauses the generator function.
NEXT() –
The main method of generators is next(). When called, it continues to execute until the next yield statement (value is optional and undefined) is encountered. Execution of the function is then paused, and the resulting value is returned to the external code.
The result of next() is always an object having two properties:
Value – The yielded value
Done –
True – IF the function code has finished
False – If the function code is not finished
Working of multiple yield Statements
The main method of generators is next() . When called, continue execution until the next yield statement (value is optional and undefined). Execution of the function is then paused and the resulting value is returned to the external code.
Example –
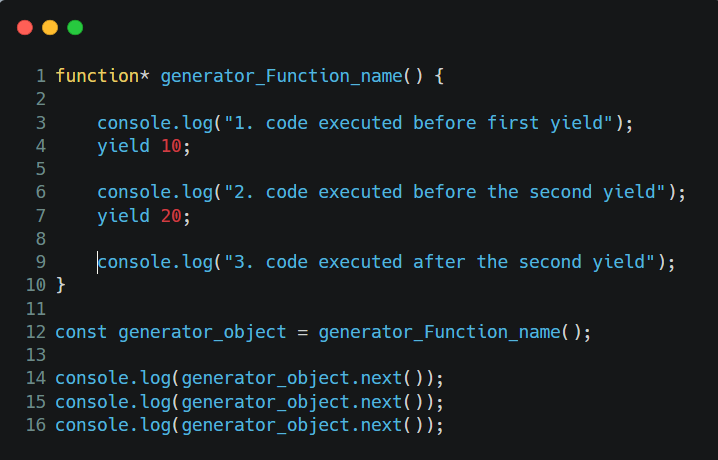
Output –
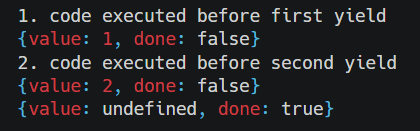
Let’s understand in detail :
The first generator_object.next() executes the code up to the first yield statement and pause the execution of the program.
The second generator_object.next() starts the program from the paused position.
When all the elements are accessed, it returns {value: undefined, done: true}
Passing Arguments to Generator Functions
Can I pass arguments to the generator?
Yes, you can also pass arguments to a generator function, similar to normal function. Again, pass parameters to .next() and use them with the yield keyword inside the generator function.
Example –

Output –

Let’s understand in detail :
1. The first generator_object.next() returns a yield value ("first next" in this case). However, let x = yield ‘first next’; does not assign a value to the variable x.
{value: "hello", done: false}
2. When it encounters generator_object.next(1) the code starts again with let x = yield ‘firstnext'; Argument 1 is assigned to x. Also, the rest of the code runs until the second yield.
Code executed ; argument passing
{value: 2, done: false}
3. When the third next() is executed, the program returns {value: undefined, done: true }. This is because there are no other yield statements.
{value: undefined, done: true}
JavaScript Generator Function with “return”
You can use return statements in generator functions. A return statement returns a value and exits a function (just like a normal function).
Example –
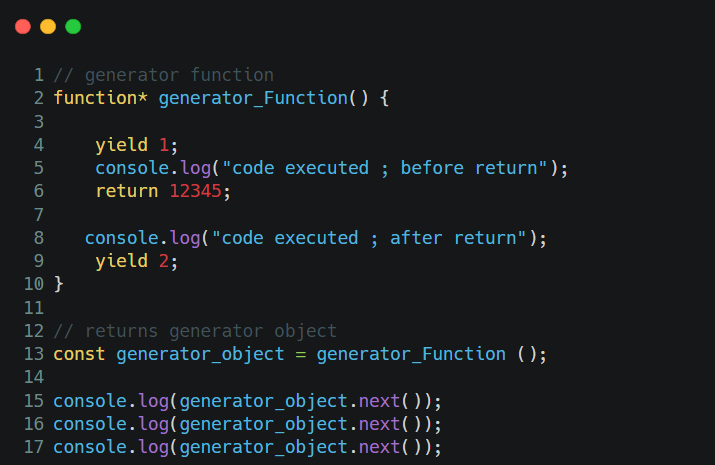
Output –

In the program above, when the return statement is encountered, the value is returned, the done property becomes true, and the function exits. Therefore the next() method returns nothing after the return statement.
Generator are Used for Implementing Iterables
Generators make it easy to implement iterators.
When implementing an iterator, you must manually define the next() method. The next() method also requires you to manually save the state of the current element.
Since generators are iterables, it helps simplify the code for implementing iterators.
Example –
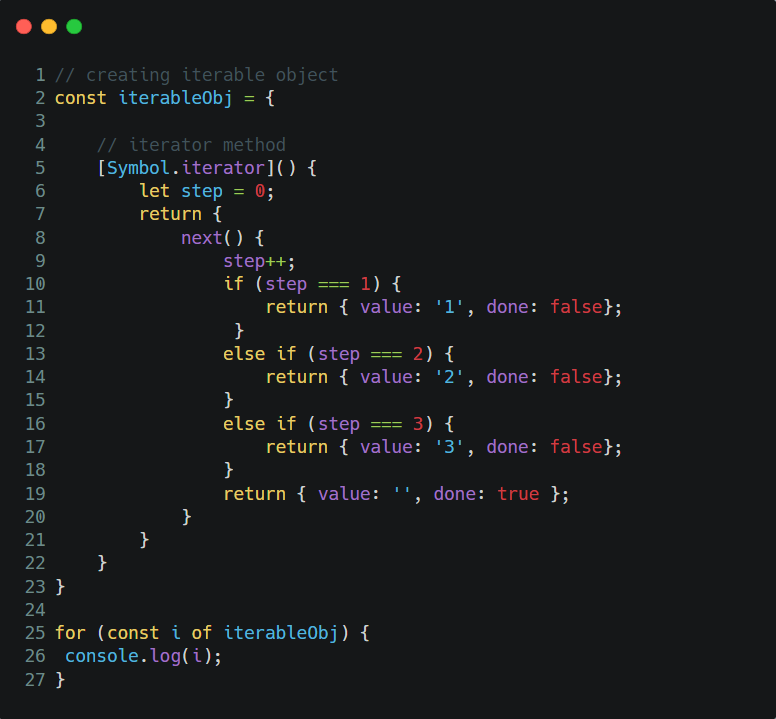
Output –
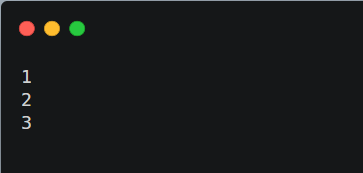
Methods of Generator Object
Method | Description |
next() | Returns a value of yield |
return() | Returns a value and then terminates the generator |
throw() | Throws an error and then terminates the generator |
Status of Generator Object
Method | Description |
suspended | Generator has halted execution but has not terminated |
closed | Generator has terminated by either encountering an error, returning, or iterating through all values |
JavaScript return Vs yield Keyword
Return | Yield |
Return a value and exit the function | Return a value and stop the function, but don't exit the function. |
It can be used in both regular functions and generator functions | Can only be used in generator functions |
Uses of Generators
Generators allow you to write cleaner code while writing asynchronous tasks.
Generators make it easy to implement iterators.
Generators only run code when it needs to.
Generators are memory efficient.
Advantages of Generators
Lazy Evaluation : This technique improves performance by only evaluating expressions as needed instead of evaluating all of the required data.
Memory Efficient : It is memory efficient because the model is only run when it is needed. This means that the value is evaluated on each next() method call.
Limitations of Generators
One cannot define a generator function with the yield keyword inside a call back function.
A generator object can no longer be used once it is closed. To repeat again, we need to create a new generator object.
If your generator object gives you a set of data and you want to access any element, random access is not possible.
Program to print 10 natural number using generators –
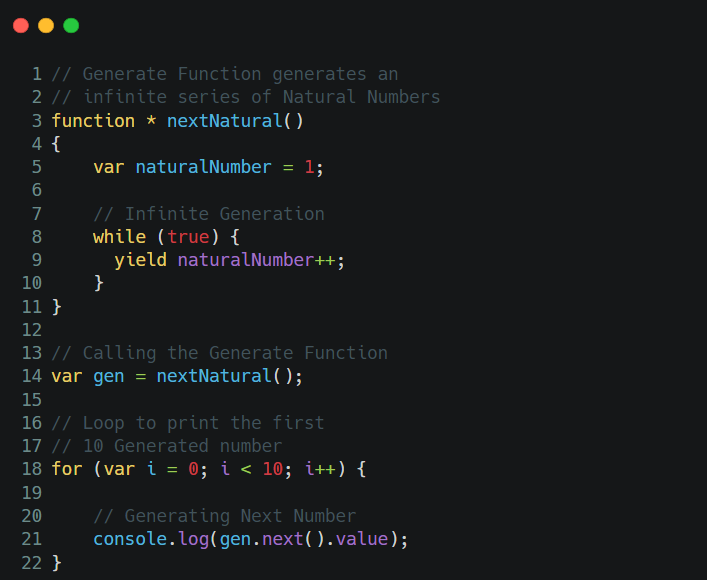
Output –

SUMMARY
Generators are created only by generator functions function* fun_name(..){…}
Only inside generators there exists a operator; yield.
External code and generators can exchange results via next/yield calls.
Generators are rarely used in modern JavaScript. However, the ability of functions to exchange data with calling code during execution is so unique that it can be useful in some cases. And indeed, they are great for creating alterable objects.
Also, in the next chapter, we'll learn about async generators, which are used to read asynchronously generated streams of data (for example, paginated fetches over the network) into for await ... loops. Web programming often deals with streaming data, so this is another very important use case.
Also Read: What is JavaScript?
Comments