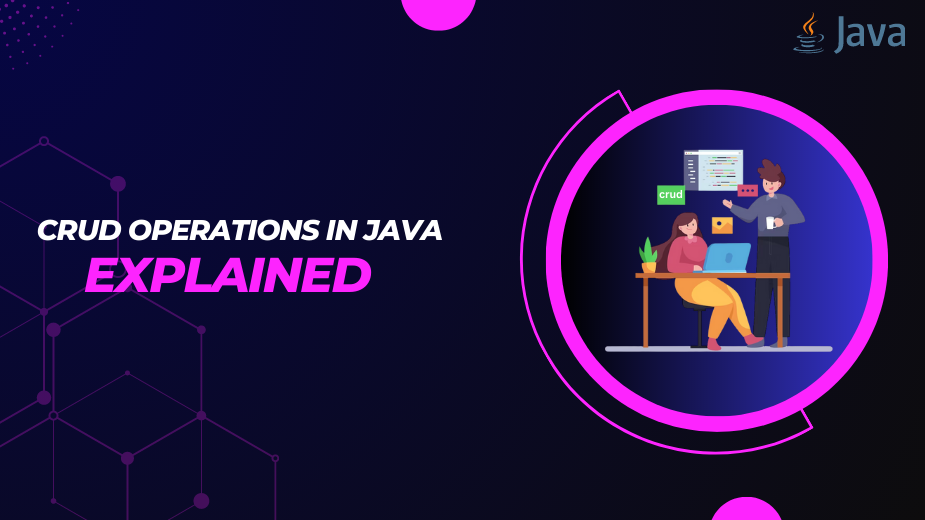
JDBC (Java Database Connectivity) definition
JDBC is a database API used in Java programming to interact with databases. JDBC classes and interfaces enable the program to communicate user queries to the selected database.
What exactly is JDBC?
JDBC is an abbreviation for Java Database Connectivity, a Java API allowing Java programs to interface with databases. It specifies a standard interface that Java programs can use to communicate with databases, regardless of the underlying database management system (DBMS).
Java programs can use JDBC to execute SQL queries, receive database result sets, and perform other database-related tasks such as inserting, updating, and removing records.
JDBC is a collection of classes and interfaces that define a common way to connect to a database. Driver, Connection, Statement, PreparedStatement, CallableStatement, ResultSet, and SQLException are the fundamental classes and interfaces of JDBC.
The Driver class is in charge of connecting to the database and creating a Connection object. The Connection object is used to build Statement and PreparedStatement objects, which are subsequently used to run SQL commands. The query results are stored in the ResultSet object.
JDBC is a database access mechanism that is type-safe, efficient, and portable. It eliminates the need to create specialized code for each database management system while reducing the amount of code that must be developed and maintained.
Furthermore, JDBC provides a consistent API for accessing databases, making designing and maintaining Java programs that interact with databases more accessible.
JDBC Connection
JDBC (Java Database Connectivity) is a Java API connecting Java programs to relational databases. It offers interfaces and classes for connecting to a database, running SQL commands, and processing the results. JDBC allows Java programs to interact with various databases, regardless of their underlying architecture.
What exactly is a JDBC Driver?
A JDBC driver uses the JDBCTM (Java Database Connectivity) API designed by Sun Microsystems, now owned by Oracle, to provide a standard method of accessing data using the JavaTM programming language.
An application can use JDBC to access several databases and operate on any Java Virtual Machine platform. Creating separate apps to access different database systems (for example, Oracle and Salesforce) is optional.
You may create a single application that can transmit SQL commands to several data sources using JDBC. SQL is the industry standard for accessing relational databases.
JDBC Driver Types
Four sorts of drivers serve as a link between the Java program and the database. These are their names:
1. JDBC-ODBC Bridge Driver
This Driver uses the ODBC driver to connect to the database. It is the most basic type of JDBC driver and is frequently used for testing and prototyping.
2. Native-API/Partially Java Driver
This Driver uses a library built in the same language as the database to convert JDBC calls into database-specific calls. It outperforms the JDBC-ODBC Bridge driver but only supports one database.
3. Network Protocol Driver (Middleware Driver)
This Driver communicates with the database through a database-independent network protocol, which is then translated into database-specific calls. This Driver has the advantage of being able to connect to several databases. Still, it requires the installation of a middleware component.
4. Pure Java Driver
This Driver is written in Java and communicates directly with the database via a database-specific interface. It provides the best performance and is the recommended Driver for use in manufacturing.
JDBC Process
The processes for connecting to a database using JDBC (Java Database Connectivity) are as follows. These are the core steps for using JDBC to connect to a database and conduct CRUD operations.
1. Loading the JDBC driver
A Java program must first load the appropriate JDBC driver before connecting to a database. There are four types of JDBC drivers: JDBC-ODBC bridge drivers, Native-API drivers, Network Protocol drivers, and thin drivers. The Driver you need is determined by the database to which you are connected and your needs.
After loading the JDBC driver, use the DriverManager to connect to the database. Using the getConnection() function and entering the database's URL and credentials.
2. Building the Statement
Once you've established a connection, you may use a statement object to execute SQL commands. A statement can be a simple SQL query or a set of SQL instructions.
3. Executing the SQL Statement
You can execute the statement by running the executeQuery(), executeUpdate(), or execute() methods, depending on the type of SQL command. ExecuteQuery() is used for SELECT statements, executeUpdate() for INSERT, UPDATE, DELETE, and other data manipulation operations, and execute() for every other type of SQL command.
4. Processing the Result
If the SQL command is a SELECT statement, the executeQuery() function's result set can be processed with a ResultSet object. You can get data from the result set using methods like next(), getInt(), getString(), and so on.
5. Closing the Connection
When you finish working with the database, close the connection by calling the terminate() function on the connection object.
Data Types in JDBC
JDBC data types are classified into two types:
Java data types: These data types are supported by the Java programming language. Java data types include both primitive (such as int, float, and double) and non-primitive (such as String, Date, and so on).
Database data types: These are the data types supported by the database to which you're linked. SQL data types (such as INT, VARCHAR, DATE, and so on) and NoSQL data types (such as BSON, JSON, and so on) are examples of database data types.
When using JDBC, ensure that the data types in your Java code match the data types in the database. You must utilize an INT in the database if you have an int in your Java code. If your Java code uses a String, the database must utilize a VARCHAR.
Architecture of JDBC
The JDBC design enables the Java program to interact with the database consistently, regardless of the database type. To carry out the request, the Java program calls methods from the JDBC API, which then calls methods from the JDBC driver. The JDBC driver connects to the database and returns the results to the Java program via the JDBC API.
The layered architecture design offers a high level of abstraction, allowing developers to write Java programs that interact with several databases using a single, standard API.
The JDBC architecture (Java Database Connectivity) consists of many components that work together to allow Java programs to interact with databases. The components of the JDBC architecture are as follows:
Application in Java
The Java application is the client-side component that communicates with the database. The Java application generates and sends JDBC queries to the database.
1. The JDBC API
This component contains a set of Java classes and interfaces for interacting with databases. The JDBC API specifies methods for connecting to databases, executing SQL commands, and analyzing results.
2. Driver Manager for JDBC
This component keeps track of available JDBC drivers and sends requests to the appropriate Driver. The Driver Manager loads the JDBC driver, connects to the database, and generates statements and result sets.
3. The JDBC Driver
This component implements the JDBC API for a specific database at a low level. The JDBC driver establishes a direct connection to the database and translates JDBC queries into database-specific instructions. There are four types of JDBC drivers:
JDBC-ODBC bridge drivers
Native-API drivers
Network Protocol drivers
Thin drivers.
4. Database
The database is the component responsible for storing and retrieving data. The database can be either SQL (such as MySQL or Oracle) or NoSQL (such as MongoDB, Cassandra, and so on).
JDBC is a database access protocol that is extensively used in Java programs. It provides a versatile and efficient way of working with databases. JDBC is a critical tool for accessing databases and saving, retrieving, and changing data, whether building a simple app or an extensive commercial system.
JDBC Architecture Types (2-tier and 3-tier)
The JDBC design uses two-tier and three-tier processing models to access a database. They are the following:
1. 2-Tier Architecture Model
A Java program communicates with the data source directly. The JDBC driver allows the program to communicate with the data source. When a user submits a query to the data source, the answers are sent to the user as results.
The data source could be on a different machine on the network to which the user is connected. This is referred to as a client/server configuration. The user's system operates as a client, and the machine running the data source acts as the server.
2. 3-Tier Architecture Model
The user's requests are sent to middle-tier services, from which commands are routed back to the data source. The results are returned to the middle tier and then to the user. And management information system directors appreciate this type of model.
Working of JDBC
JDBC is an abbreviation for Java Database Connectivity. JDBC is a Java API that connects to and executes queries against databases. It is included in JavaSE (Java Standard Edition). JDBC API connects to the database using JDBC drivers. JDBC drivers are classified into four types:
JDBC-ODBC Bridge Driver,
Native Driver,
Network Protocol Driver, and
Thin Driver
JDBC API can access tabular data stored in any relational database. We may save, edit, remove, and retrieve data from the database using the JDBC API. It is similar to Microsoft's Open Database Connectivity (ODBC).
JDBC is currently at version 4.3. It has been steady since September 21st, 2017. Its foundation is the X/Open SQL Call Level Interface. The java.sql package contains JDBC API classes and interfaces. The following is a list of popular JDBC API interfaces:
Driver interface
Connection interface
Statement interface
PreparedStatement interface
CallableStatement interface
ResultSet interface
ResultSetMetaData interface
DatabaseMetaData interface
RowSet interface
The following is a collection of popular JDBC API classes:
DriverManager class
Blob class
Club Class
Types class
Creating a JDBC Application
package com.vinayak.jdbc;
import java.sql.*;
public class JDBCDemo {
public static void main(String args[])
throws SQLException, ClassNotFoundException
{
String driverClassName
= "sun.jdbc.odbc.JdbcOdbcDriver";
String url = "jdbc:odbc:XE";
String username = "Scott";
String password = "tiger";
String query
= "insert into students values(109, 'bhatt')";
// Load driver class
Class.forName(driverClassName);
// Obtain a connection
Connection con = DriverManager.getConnection(
url, username, password);
// Obtain a statement
Statement st = con.createStatement();
// Execute the query
int count = st.executeUpdate(query);
System.out.println(
"number of rows affected by this query= "
+ count);
// Closing the connection as per the
// requirement with connection is completed
con.close();
}
} // class
The preceding example shows how to use JDBC to access a database. To connect to the database, the application employs the JDBC-ODBC bridge driver. You must import the java.sql package and use its classes to offer basic SQL functionality.
Why Should JDBC Be Used?
JDBC (Java Database Connectivity) is a widely used API that allows Java programs to communicate with relational databases consistently. Here are some of the benefits of using JDBC:
JDBC lets Java programs interact with many relational database systems, making creating database applications that can be deployed across multiple platforms more accessible.
JDBC offers secure database connectivity through industry-standard security techniques such as SSL/TLS and Kerberos.
JDBC delivers high-performance database access and efficient processing of massive datasets with capabilities such as connection pooling and batch updates.
JDBC allows developers to work at several levels of abstraction, from raw SQL queries to high-level ORM frameworks, allowing them the freedom to choose the optimal technique for their use case.
JDBC has been around for nearly two decades. It is a mature and robust technology that is widely used by developers and organizations all over the world.
JDBC is extendable, meaning that developers can construct their own API implementations to handle non-relational databases or bespoke data sources.
JDBC provides a powerful and versatile interface for Java applications to connect with relational databases, making it a necessary tool for developing robust and scalable database systems.
Features of JDBC
JDBC (Java Database Connectivity) is an API that allows Java programs to communicate with relational databases in a standard manner. Here are some of its essential characteristics:
JDBC allows Java applications to connect to various relational databases, including Oracle, MySQL, Microsoft SQL Server, and PostgreSQL.
JDBC provides a straightforward interface for executing SQL statements, such as queries, updates, and deletes, and processing the results.
Pre-compiled SQL statements that can be performed numerous times with varying parameter values are supported by JDBC. By lowering the overhead of constructing SQL statements and eliminating SQL injection threats, prepared statements can increase efficiency and security.
Transactions allow many SQL statements to be executed as a single unit of work, ensuring that all statements are committed or rolled back as a group.
JDBC allows developers to retrieve metadata about the database and its objects, such as tables, columns, and indexes. This data can be used to build SQL statements dynamically or offer end users metadata.
JDBC offers batch updates, which allow numerous SQL statements to be run in a batch, boosting efficiency by minimizing the number of database round trips.
JDBC offers connection pooling, which allows several clients to share a pool of database connections, boosting efficiency and scalability by lowering the overhead of generating and removing connections.
JDBC supports numerous data kinds, including numeric, text, date, time, and binary data.
Thus, JDBC is a powerful and flexible API for developing resilient and scalable database applications in Java.
Conclusion
Finally, JDBC (Java Database Connectivity) is a robust API that allows Java programs to communicate with relational databases. It offers a consistent interface for connecting to databases, running SQL queries, and altering data. Developers can use JDBC to create portable Java database applications between platforms and database systems.
JDBC is an established and extensively used technology that has been in use for more than two decades. It is a required component of the Java platform. It is supported by almost all relational database systems, including Oracle, MySQL, Microsoft SQL Server, and PostgreSQL.
The API is adaptable, allowing developers to work at many degrees of abstraction, ranging from raw SQL queries to high-level ORM frameworks. JDBC is also expandable, allowing developers to construct API implementations to accommodate non-relational databases or bespoke data sources.
JDBC is a valuable tool for developing solid and scalable Java database applications. Its ease of use, versatility, and widespread adoption make it popular among developers and organizations worldwide.
Comments