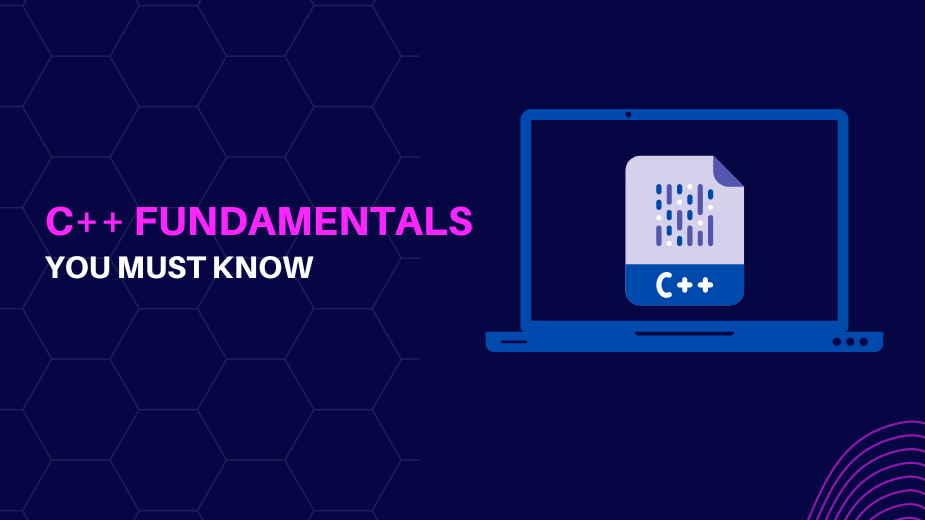
Introduction To C++
C++ is general-purpose programming. It is an extension of the C programming language and provides object-oriented features such as classes, inheritance, and polymorphism. C++ is used for developing system software, application software, device drivers, embedded software, high-performance server and client applications, and entertainment software such as video games.
Data types - Datatype is a classification of data that describes the type of information a particular piece of data holds and how it can be used
Eg-
Integer types (e.g. int, short, long)
Floating-point types (e.g. float, double)
Character types (e.g. char)
Boolean type (bool)
And more
Pointers – A type of variable that stores the address of another variable. Pointers are also used to dynamically allocate memory and to create data structures such as linked lists and trees.
Structure – A user-defined data type that groups related data elements of different data types together under one name. A structure can be used to represent a record. It contains data members.
Function – A function in C++ is a block of code that performs a specific task. It is a subprogram that is used to perform a particular task. It helps in reducing the complexity of the main program.
Questions for C++ –
What is the difference between a pointer and a reference in C++?
A pointer is a variable that holds the memory address of another variable and can point to different memory locations during its lifetime, whereas a reference is an alias to another variable and cannot be reassigned to point to a different memory location once it is initialized.
What are the advantages of using structures in C++?
Structures are useful for organizing related data items together, making them easier to access and manipulate. Structures can also be used to create user-defined data types.
What is an argument of a function and how are arguments passed to a function in C++?
An argument of a function is a value passed to the function when it is called. Arguments are passed to a function in C++ using either pass-by-value or pass-by reference.
OOP – Object-Oriented Programming
Oops is all about classes and objects
Features of Oops –
Inheritance - a mechanism of reusing and extending existing classes without modifying them.
Single
Multiple
Multilevel
Hierarchical
Hybrid
Polymorphism - that a call to a member function will cause a different function to be executed depending on the type of object
Compile-time Polymorphism
Function Overloading
Operator Overloading
Runtime Polymorphism
Virtual Functions
Encapsulation – a concept in which data and functions are combined in a single unit called class
Abstraction - The process of only showing the necessary details to the user and hiding the other details in the background.
Constructors – A constructor in C++ is a special member function of a class that is executed whenever we create new objects of that class.
Destructor - member function that is invoked automatically when the object goes out of scope or is explicitly destroyed by a call to delete
Access specifiers – It is used to restrict access to certain parts of a program.
Public
Private
Protected
Friend Function – a function declared with the keyword “friend” that is given special access rights to the private and protected members of a class. It is not a member of the class, but it has access to all the private and protected members of the class. It is usually used when two classes are tightly coupled and need access to each other's data
Questions –
What are the various types of constructors in C++?
The most common classification includes –
Default
Parameterized
Copy
Are class and structure the same? If not, what's the difference between a class and a structure?
No, class and structure are not the same. Though they appear to be similar, they have differences that make them apart. For example, the structure is saved in the stack memory, whereas the class is saved in the heap memory. Also, Data Abstraction cannot be achieved with the help of structure, but with class, Abstraction is majorly used.
What is an abstract class?
An abstract class is a special class containing abstract methods. The significance of an abstract class is that the abstract methods inside it are not implemented and are only declared. So as a result, when a subclass inherits the abstract class and needs to use its abstract methods, they need to define and implement them.
What are Base and derived classes?
In inheritance, the existing class is called the base or parent class and the newly created class is called the derived or child class. A derived class can be inherited from more than one class, it all the thing depends on the requirements.
What is the difference between method overloading and method overriding?
The primary difference between method overloading and method overriding is that method overloading is done within the same class, while method overriding is done in two different classes that have an inheritance relationship.
Memory management
It is the process of allocating and deallocating memory during the runtime of a program. This process is essential for the efficient use of memory and for ensuring that the program does not run out of it. It can be done manually using “new” and “delete” operators or through the use of smart pointers.
Memory management also involves cleaning up memory that is no longer needed, such as freeing the memory associated with objects that have been deleted from a container.
New Operator - is used to dynamically allocate memory for an object at runtime. The operator returns a pointer to the newly allocated memory, which can be used to construct an object of the specified type.
Syntax – pointer_variable = new type;
Delete Operator - is used to deallocate memory that was previously allocated by the "new" operator. The operator is used to free up the memory and prevent memory leaks.
Syntax - delete pointer_variable;
Questions –
What is a memory leak in C++?
A memory leak in C++ occurs when the memory that is no longer needed by a program is not deallocated, resulting in a loss of available memory. This can happen if the delete operator is not used to deallocate the memory that was allocated using the new operator.
What is a smart pointer in C++?
Smart pointers are a C++ feature that provides an alternative to raw pointers. They are objects that act like pointers, but also manage the lifetime of the memory they point to, automatically deallocating the memory when it is no longer needed.
What are the types of memory management in C++?
There are two primary types of memory management in C++: static memory management, which is done at compile time; and dynamic memory management, which is done at runtime.
What is the difference between static and dynamic memory management?
In static memory management, memory is allocated for a program at compile time and is not changed during the program's execution. In dynamic memory management, memory is allocated and deallocated at runtime as needed.
Can you explain how garbage collection works?
When an object is no longer being used by the program, the garbage collector will automatically free up the memory that was being used by that object. The garbage collector runs periodically, so it may not happen immediately when the object is no longer needed.
Files and Streams –
Files and Streams in C++ Files and streams are important components of the C++ language, and understanding how to use them effectively is key to writing efficient and effective code.
A file is a collection of data stored in a computer’s memory or on a storage device, such as a hard drive. It can store text, images, audio, or video. Streams, on the other hand, are used to manipulate data from files. It provides a way to read and write data to a file.
The "fstream" library provides classes for working with files and streams, including "ifstream" for reading from files, "ofstream" for writing to files, and "fstream" for both reading and writing.
Library functions allow the programmer to open, read, and write files, as well as manipulate streams to read and write data.
Questions –
What is a file in C++?
A file is a sequence of bytes stored on a secondary storage device, such as a hard drive or CD, which can be read and written by a program.
What is the use of the fstream class in C++?
The fstream class is used to read and write to files, and to access the streams associated with them.
What is a stream in C++?
A stream is an interface that allows data to be transferred between a program and an external device (e.g. a file or a network connection).
What is the difference between a file stream and a string stream in C++?
A file stream is used to read and write data from a file, while a string stream is used to read and write data from a string.
What are the different types of streams in C++?
The different types of streams in C++ are Input Streams, Output Streams, File Streams, String Streams, and Network Streams.
What is the difference between an input and an output stream?
An input stream is used to read data from a source, while an output stream is used to write data to a destination.
What is the purpose of the tellg() and tellp() functions?
The tellg() function is used to get the current position of the get pointer in an input stream, while the tellp() function is used to get the current position of the put pointer in an output stream.
What is the purpose of the seekg() and seekp() functions?
The seekg() function is used to set the position of the get pointer in an input stream, while he seekp() function is used to set the position of the put pointer in an output stream.
What is the purpose of the write() function?
The write() function is used to write data to an output stream.
What is the purpose of the read() function?
The read() function is used to read data from an input stream.
What is the purpose of the open() function?
The open() function is used to open a file or device for reading or writing.
What is the purpose of the close() function?
The close() function is used to close a file or device that was previously opened for reading or writing.
C++ (Namespaces)
Namespaces in C++ are a way of grouping together related code and data.
Namespaces in C++ are used to organize code and prevent name collisions. They allow different pieces of code to use the same name without causing confusion. Namespaces are declared using the keyword 'namespace'. They can contain variables, functions, classes, and other namespaces. Namespaces can be nested within other namespaces, and they can be imported using the 'using' keyword.
Namespaces can also be used to provide a scope for variables. This means that variables declared in a namespace are visible only to code within the same namespace. They are an essential part of C++ programming and should be used whenever possible.
Questions -
What is a namespace in C++?
A namespace is a container that holds a set of identifiers (such as variable names, function names, etc.) that are used to organize and group related code together.
How do you define a namespace in C++?
A namespace is defined using the "namespace" keyword, followed by the name of the namespace, and a set of curly braces containing the code that belongs to that namespace
How do you access an identifier in a namespace?
To access an identifier in a namespace, you use the "namespace_name::identifier" syntax.
What is the purpose of the "using" keyword in a namespace?
The "using" keyword is used to bring the identifiers from a namespace into the current scope so that they can be used without the namespace prefix.
Can a namespace contain other namespaces?
Yes, a namespace can contain other namespaces. This is known as a nested namespace.
What is the difference between using "using namespace" and "using namespace_name::identifier"?
"using namespace" brings all identifiers in a namespace into the current scope, while "using namespace_name::identifier" only brings the specific identifier into the current scope.
How do you define a global namespace in C++?
There is no need to define a global namespace in C++, as all identifiers not defined within a namespace are automatically part of the global namespace.
Can a namespace have multiple names?
No, a namespace can only have one name.
Can a namespace have a variable or function as a member?
Yes, a namespace can have variables and functions as members
STL (Standard Template Library) –
STL stands for the Standard Template Library. It is a collection of C++ template classes that provide common data structures and functions with which to manipulate them. These data structures include vectors, lists, queues, and stacks, among others. The functions provided by the STL include operations such as sorting, searching, and manipulating the data stored in these data structures. The STL is designed to be efficient, flexible, and easy to use, making it a valuable tool for C++ programmers.
For more detailed STL documentation – Click here
Question –
What is the difference between a vector and a list?
A vector is a sequence container that stores elements in contiguous memory locations. A list is a doubly linked list container that stores elements in non-contiguous memory locations.
What are the advantages of using STL?
The advantages of using STL are: code reusability, increased productivity, improved performance, and portability of code.
What is the difference between a stack and a queue?
A stack is a LIFO (last-in-first-out) data structure, while a queue is a FIFO (first-in-first-out) data structure.
What is the purpose of the std::sort() algorithm?
The std::sort() algorithm is used to sort elements in a container. It uses the quicksort algorithm by default but can be customized to use other sorting algorithms.
Also Read: Explore the Fundamentals of Game Development
Commenti