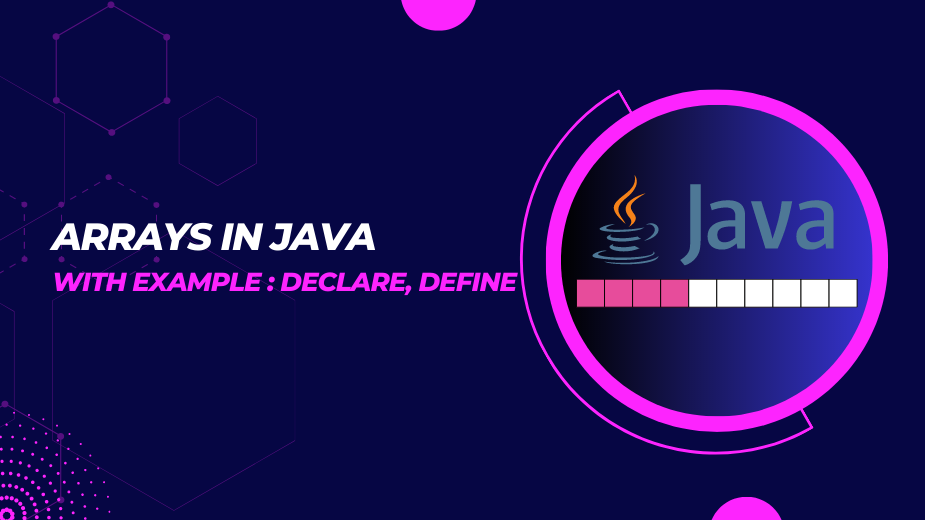
To understand the concept of arrays, you must first understand why arrays are needed in the first place. Your birthday is approaching, and you have a room full of mates to treat to chocolates. They are all at college/school with you. But you recall having 70 classmates. But you can hardly hold ten chocolates in both hands. The most you can carry is seven. So, do you make round trips from school/college to the store until every one of your buddies has one chocolate? No!
You acquire a large plastic jar and place all the chocolates inside. This makes transporting all the chocolates easier and reduces the possibility of spillage!
And Arrays in Java serve the same purpose! Let's get started.
Arrays in Java
What are Arrays? Arrays are groups of data objects with the same data type referred to by the same name. Each array element is kept to be determined using a mathematical formula applied to its index. An Integer array, for example, will have all integer elements, whereas a String array will include Strings for each array element.
The memory elements are stored in a contiguous memory location, which means they are stored one after the other.
However, there are some differences between Java arrays and those in other languages:
In Java, all arrays are dynamically allocated.
The length of an array in Java is calculated with .length. This may appear to be identical to the string length() method. The principle is that the length is a final variable that puts the length of arrays. This is not true for strings because the string provides a length() method that calculates the length of the string. In Java, arrays are declared the same way as other variables. The only change is that at the end of the declaration, a [] is inserted.
The array's size must be an integer value that is neither short nor long.
The variables in the array begin at index 0.
Java can also be used as a parameter in methods and local and static variables.
An Object is a superclass of arrays.
Arrays implement Cloneable and Serializable interfaces.
When arrays contain primitive datatypes, they are stored in contiguous memory regions. Arrays of objects, on the other hand, are kept in heap memory.
Indexes are always present in arrays. These indices typically represent the position of each array element. Because the items are continuous, indexing is done from 0 (the first element) to n-1, where n is the array's length. For example, an array of length five would have indices from 0 to 4. (5-1).
Why are Java Arrays required?
Memory is a costly resource in today's society, and good memory usage saves a lot of money and work. As a result, arrays can be used in programming to store a large amount of data with similar data types. For example, if we need to hold a hundred integer variables, we can define an array of size 100 and type int instead of saying "int a,b,c........".
This way, we don't have to give each variable a unique name, and they can all be accessed independently by their index in the array. We can use arr[87] to get the 87th integer, where arr is the array's name.
Arrays Index Out of Bounds Exception in Java
A common error all programmers make when utilizing arrays is attempting to access indexes beyond the limit. For instance, if an array has a length of 6, the program can use any index in the array between 0 and 5. However, the program will occasionally attempt to access items outside this range. The compiler then throws an Arrays Index Out Of Bounds Exception error.
Example:
package com.dataflair.arrayclass;
class ExceptionArrayIndex {
public static void main(String[] args) {
int arr[] = new int[] {
1,
2,
3,
4,
5
};
int j;
for (j = 0; j <= arr.length; j++) {
System.out.println(arr[j]);
}
}
}
Output:
1
2
3
4
5
Exception:
java.lang exception in thread "main" ArrayIndexOutOfBoundsException: Index 5 is outside the bounds of the array for length 5.
Array Declaration in Java
Arrays are declared in a straightforward method. It includes the variable type, name, and closed box brackets.([]).
The following is the syntax:
type var_name []
or
type[] var_name;
Writing this code, however, does not allocate memory to the array. It just tells the compiler that the array exists. You must use the new keyword to enable memory to the array, just like we did with objects.
The syntax then becomes:
type var_name [] = new type [size_of_array];
The data type of the array to be declared is indicated by type, and square brackets indicate the array size.
When you declare an array, all of its items are saved as the default values for that particular datatype. For example, if you declare an integer array, all of the items within the array will be set to 0, which is the datatype's default value.
However, the values are set to null if the data type is a reference datatype.
This method of allocating memory to an array in Java makes all arrays dynamically allocated.
A for loop and a for each loop can both access Java items. However, the array elements cannot be changed by using the for each loop.
Literals can be used to declare arrays in Java.
If all of the items of an array are known in advance, they can be added to an array literal.
The array literal declaration is used in the following way:
datatype <var_name>[] = new datatype[] {element1,element2,element3….element n};
Java for-each loop to iterate over arrays
Like the for loop, the for-each loop can traverse over arrays. When accessible by a for-each loop, the elements of the loop cannot be changed.
Syntax:
for ( < datatype > <variable > :<collection > ) {
//code to be executed
}
Example for illustrating for-each loop to iterate over arrays:
class ForEachArray {
public static void main(String[] args) {
int arr[] = new int[] {
1,
2,
3,
4,
5
};
for (int i: arr) {
System.out.println(i);
}
}
}
Output:
1
2
3
4
5
Java Program for illustrating Arrays in programs
public class BasicArray {
public static void main(String[] args) {
System.out.println("Today’s program shows declaration and use of java arrays");
int i;
//Declaring array literal
int arr_lit[] = new int[] {
69,
666,
420,
117
};
//Declaring array object
int arr_obj[] = new int[6];
//Using for loop to access elements in arr_obj
for (i = 0; i < 6; i++) {
arr_obj[i] = i;
}
//using for each loop to iterate through arr_lit
System.out.println("The array literal has specific values");
for (int j: arr_lit) {
System.out.print(j + " ");
}
System.out.println("");
//using for loop to iterate over each element in arr_obj
System.out.println("The array object has some values");
for (i = 0; i < 6; i++) {
System.out.print(arr_obj[i] + " ");
}
//We go from 0 to the number before the length of the array
//because arrays are indexed from 0 to n-1
//n is the size of the array
}
}
Output:
Today's program shows declaration and use of java arrays
The array object has some values
69 666 420 117
The array object has values:-
0 1 2 3 4 5
Java memory representation for single-dimensional arrays
When the compiler runs the code int a[] = new int[5], it first constructs a reference variable called a'. This variable refers to the data stored in the array. The data elements are continuous and numbered from 0 through (length of the array -1). It ranges from 0 to 4 in this situation.
Anonymous Arrays in Java
You do not need to give an array a name when passing it to a method. Anonymous arrays are arrays that have no names. They can be delivered directly from the function call to the method.
Example:
class AnonymousArray {
static void printArray(int arr[]) {
for (int i: arr) {
System.out.println(i);
}
}
public static void main(String[] args) {
printArray(new int[] {
1,
2,
3,
4,
5,
0
});
}
}
Array members in Java
Arrays are objects within a class, and the Array class is a direct subclass of the Object class. As a result, each component of the array object must meet the following requirements:
The array's final variable length must be either positive or zero.
Each member inherits all of the Object class's methods except the clone method.
This clone function replaces the process in the Object class and throws no checked exceptions.
Java Array of Objects
Remember how we started the chapter with your birthday and you carrying a box of chocolates instead of taking each one by hand? Consider candy to be a class. This candy comes in various forms, such as lollipops in flavors like chocolate or vanilla.
As a result, instead of having distinct objects, all of these can be shown as an array of candy-type objects.
The syntax for declaring an array of objects is the same:
<datatype> var_name[] = new <datatype>[size];
Example:
package com.dataflair.arrayclass;
public class Candy {
//class to define candy.
public String candy_name;
public String flavour;
Candy(String cname, String flav) {
candy_name = cname;
flavour = flav;
}
public static void main(String[] args) {
int i;
Candy arr_obj[] = new Candy[5];
//created five objects of candy
arr_obj[0] = new Candy("Alpenliebe", "Strawberry");
arr_obj[1] = new Candy("Alpenliebe", "Mango");
arr_obj[2] = new Candy("Eclairs", "Dark-chocolate");
arr_obj[3] = new Candy("Mars", "White-Chocolate");
arr_obj[4] = new Candy("Snickers", "Nuts-Caramel");
//declared objects in the array candy
for (i = 0; i < 5; i++) {
System.out.println(arr_obj[i].candy_name + " the taste of " + arr_obj[i].flavour);
}
}
}
Output:
Alpenliebe has the taste of Strawberry
Alpenliebe has the taste of Mango
Eclairs has the taste of Dark-Chocolate
Mars has the taste of White-Chocolate
Snickers has the taste of Nuts-Caramel
Multidimensional Arrays
Arrays are not confined to a single dimension. There are multidimensional arrays that are utilized in mathematical calculations. Each element of one array holds a reference to another array, resulting in these.
In programming, two-dimensional arrays are relatively common. On the other hand, the JVM restricts the maximum number of dimensions that can be produced to 255.
Syntax:
<datatype>[][]<var_name>= new <datatype>[size1][size2];
or
<datatype><var_name>[][]= new <datatype>[size1][size2];
or
<datatype>[]<var_name>[]= new <datatype>[size1][size2];
Example:
public class DoubleDimArray {
public static void main(String[] args) {
//Using multidimensional array literal
int arr_lit[][] = {
{
1,
2,
3
},
{
4,
5,
6
},
{
7,
8,
9
}
};
//using multidimensional array object
int val = 1;
int matrix[][] = new int[4][4];
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
matrix[i][j] = val;
val++;
}
}
//printing the matrix
System.out.println("The matrix is");
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
System.out.print(matrix[i][j] + "\t");
}
//Adding a blank line after every row
System.out.println("");
}
}
}
Output:
The matrix is
1 2 3 4
5 6 7 8
9 10 11 12
13 14 15 16
Cloning Array
Cloning is a complex notion in Java. Producing another array with the same values is known as cloning an array. However, the cloning procedure differs between single-dimensional and multidimensional arrays.
Single-Dimension Cloning: When a single-dimensional array is cloned, every element from the original array is copied. It should be noted that all of the elements have been copied, but the references are different. This signifies that the array members that have been cloned have the same value but distinct references. This is referred to as a deep copy.
Multidimensional Cloning: Arrays that contain arrays are referred to as multidimensional arrays. Simply put, each element in a multidimensional array is a pointer to another array. When cloning, a new array with the array items is produced, but the subarrays are shared via references. Only a single array is made when cloning multidimensional arrays, with all sub-arrays referenced. This is referred to as shallow copy.
Syntax:
<array2> = <array1>.clone();
class CloningArrays {
public static void main(String[] args) {
int newarr[] = new int[] {
1,
2,
3,
4,
5
};
int clonearr[] = newarr.clone();
for (int i: clonearr) {
System.out.println(i);
}
System.out.println("Check if references are equal");
System.out.println(clonearr == newarr);
//cloning multidimensional arrays
int multnew[][] = new int[][] {
{
1,
2,
3
},
{
4,
5,
6
},
{
7,
8,
9
}
};
int clonemult[][] = multnew.clone();
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++) {
System.out.print(clonemult[i][j] + " ");
}
System.out.println("");
}
System.out.println("Check if references to the outer array are the same. ");
System.out.println(multnew == clonemult);
System.out.println("Check if references of the inner array are the same. ");
System.out.println(multnew[0] == clonemult[0]);
}
}
Output:
1
2
3
4
5
Check if references are equal
false
1 2 3
4 5 6
7 8 9
Check if references to the outer array are the same.
false
Check if the references of the inner array are the same.
true
Arrays' Benefits and Drawbacks in Java
Arrays are widely used in distributed systems and for linearly storing data. This data format has the advantage of allowing data members to be easily retrieved and indexed. Any element in the array can be retrieved at random.
However, there is one disadvantage: the array's size is fixed. It stays the same throughout the operation.
Conclusion
Finally, arrays are a fundamental data structure in Java programming that allows storing and manipulating numerous components of the same data type. Arrays are an effective and user-friendly way to organize and access data in a programme.
Java includes built-in functions for sorting, searching, and altering arrays and various methods and tools for working with arrays. Developers can also write custom methods to modify arrays based on their needs.
While arrays have advantages, they also have certain restrictions, such as their fixed size and inability to adjust their size once established. However, Java has other data structures, such as ArrayLists and LinkedLists, can circumvent these constraints.
Understanding arrays is critical for any Java developer. Arrays are a robust data management and organization tool, and understanding how to utilize them properly can lead to more efficient and productive programming.
Read, Also: Java DataTypes, Loops & more
Comments