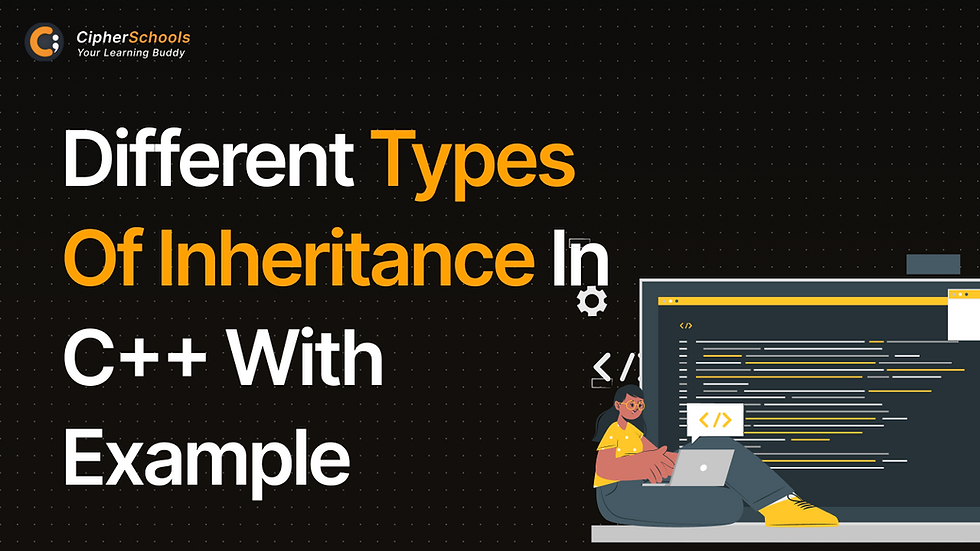
Inheritance is a fundamental concept in object-oriented programming (OOP) that allows a class to inherit properties and behaviors from another class. In C++, there are several types of inheritance, each serving a specific purpose and offering unique features. In this comprehensive guide, we'll delve into the various types of inheritance in C++ with syntax, examples, and explanations.
What is Inheritance in C++?
Inheritance is a mechanism in C++ where a class (derived class) inherits properties and behaviors from another class (base class). It promotes code reusability, extensibility, and modularity by allowing classes to build upon existing functionality.
What are the Different Types of Inheritance in C++?
Single Inheritance in C++:
Single inheritance involves a derived class inheriting from only one base class. It forms a linear hierarchy, where each class has only one parent class. Here's an example:
class Base {
// Base class members
};
class Derived : public Base {
// Derived class members
};
Multiple Inheritance in C++:
Multiple inheritance allows a derived class to inherit from multiple base classes. It enables a class to combine features from different sources. However, it can lead to the diamond problem, where ambiguity arises due to overlapping features.
Example:
class Base1 {
// Base1 class members
};
class Base2 {
// Base2 class members
};
class Derived : public Base1, public Base2 {
// Derived class members
};
Multilevel Inheritance in C++:
Multilevel inheritance involves a chain of inheritance, where a derived class inherits from another derived class. It forms a hierarchical structure, enabling classes to specialize further.
Example:
class Base {
// Base class members
};
class Derived1 : public Base {
// Derived1 class members
};
class Derived2 : public Derived1 {
// Derived2 class members
};
Hierarchical Inheritance in C++:
Hierarchical inheritance occurs when multiple derived classes inherit from a single base class. It allows for specialization and diversification of classes.
Example:
class Base {
// Base class members
};
class Derived1 : public Base {
// Derived1 class members
};
class Derived2 : public Base {
// Derived2 class members
};
Hybrid Inheritance in C++:
Hybrid inheritance combines multiple types of inheritance, such as single, multiple, and hierarchical. It results in complex class relationships and should be used judiciously.
Example:
class Base1 {
// Base1 class members
};
class Base2 {
// Base2 class members
};
class Derived1 : public Base1 {
// Derived1 class members
};
class Derived2 : public Base1, public Base2 {
// Derived2 class members
};
Diagram of Inheritance in C++:
Inheritance in C++ can be represented using UML (Unified Modeling Language) diagrams, showcasing the relationships between classes and their inheritance hierarchy.
Modes of Inheritance in C++:
In C++, inheritance can occur in three modes: public, protected, and private. These modes determine the visibility of inherited members in the derived class.
Public mode: In public inheritance, public members of the base class remain public in the derived class, protected members remain protected, and private members remain inaccessible.
Protected mode: In protected inheritance, both public and protected members of the base class become protected in the derived class, while private members remain inaccessible.
Private mode: In private inheritance, both public and protected members of the base class become private in the derived class, while private members remain inaccessible.
Difference between Single and Multiple Inheritance in C++:
Single inheritance involves a derived class inheriting from only one base class, while multiple inheritance allows a derived class to inherit from multiple base classes.
Conclusion:
Inheritance is a powerful mechanism in C++ that enables code reuse, extensibility, and modularity. By understanding the different types of inheritance and their characteristics, developers can design more flexible and maintainable software systems.
FAQs:
What are the 6 types of inheritance?
The six types of inheritance are single, multiple, multilevel, hierarchical, hybrid, and multipath inheritance.
What is single and multilevel inheritance in C++?
Single inheritance involves a derived class inheriting from only one base class, while multilevel inheritance involves a chain of inheritance, where a derived class inherits from another derived class.
What is inheritance in object-oriented programming?
Inheritance in object-oriented programming is a mechanism where a class (derived class) inherits properties and behaviors from another class (base class), promoting code reuse and extensibility.
Comments