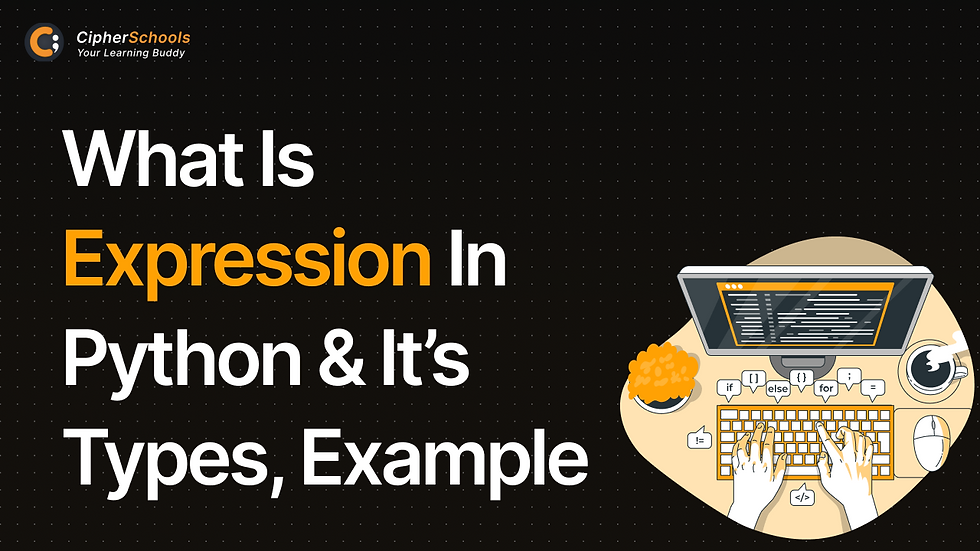
Introduction to Expression in Python with Types and their Example
Expressions play a crucial role in Python programming, representing combinations of variables, operators, and method calls that produce a value. In this comprehensive guide, we'll explore expressions in Python, including their types, examples, and practical applications.
What is Expression in Python?
An expression in Python is a combination of values, variables, operators, and function calls that evaluates to a single value. It can be as simple as a variable or as complex as a mathematical formula. Expressions are the building blocks of Python programs, used to perform calculations, evaluate conditions, and manipulate data.
Types of Expressions in Python with Examples:
Constant Expressions:
Constant expressions consist of literal values, such as numbers or strings, which do not change during program execution. Examples include:
5
"Hello, World!"
Arithmetic Expressions:
Arithmetic expressions involve mathematical operations, such as addition, subtraction, multiplication, and division. Examples include:
2 + 3
10 - 5
3 * 4
20 / 5
Integral Expressions:
Integral expressions involve integer values and operations, producing integer results. Examples include:
15 // 4 # Integer division
25 % 7 # Modulus operation
Floating Expressions:
Floating expressions involve floating-point values and operations, producing floating-point results. Examples include:
3.14 + 2.71
8.0 / 2.0
Relational Expressions:
Relational expressions compare values and produce Boolean results (True or False). Examples include:
10 > 5
5 == 5
Logical Expressions:
Logical expressions involve Boolean values and logical operators (and, or, not). Examples include:
True and False
not True
Bitwise Expressions:
Bitwise expressions manipulate binary representations of integers using bitwise operators (&, |, ^, ~, <<, >>). Examples include:
5 & 3
10 | 7
Combinational Expressions:
Combinational expressions combine multiple types of expressions using parentheses and operators. Examples include:
(3 + 4) * (5 - 2)
What is the use of regular expression in Python?
Regular expressions (regex) in Python are powerful tools for pattern matching and string manipulation. They allow for flexible and efficient searching, replacing, and validation of text data.
Comparison Expression in Python:
Comparison expressions evaluate conditions and return Boolean values based on the comparison result. Examples include:
x > y
a == b
Multiple Operators in Python Expression:
Python expressions can involve multiple operators, such as arithmetic, relational, and logical operators, to perform complex computations. Examples include:
(2 + 3) * (4 - 1) / 2
x > 0 and x < 10
Difference between Statement and Expression in Python:
A statement in Python is a complete unit of execution, while an expression is a piece of code that evaluates to a value. Statements perform actions, while expressions produce results.
Conclusion:
Expressions are fundamental elements of Python programming, enabling the manipulation and transformation of data through various operations and functions. By understanding the types and characteristics of expressions, developers can write efficient and expressive Python code.
FAQs:
What is an expression and a statement in Python?
An expression in Python is a combination of values, variables, and operators that evaluates to a single value, while a statement is a complete unit of execution that performs an action.
What is a math expression in Python?
A math expression in Python involves mathematical operations, such as addition, subtraction, multiplication, and division, to perform calculations and produce numeric results.
What are operators and expressions in Python with example?
An operator in Python is a symbol or keyword used to perform operations on operands, while an expression is a combination of values, variables, and operators that evaluates to a single value. Example:
x = 5
y = 10
result = x + y
Which module in Python supports regular expressions?
The 're' module in Python supports regular expressions, providing functions and methods for pattern matching and string manipulation using regex syntax.
Comments