Stack in Data Structure
- Pushp Raj
- Oct 23, 2024
- 2 min read
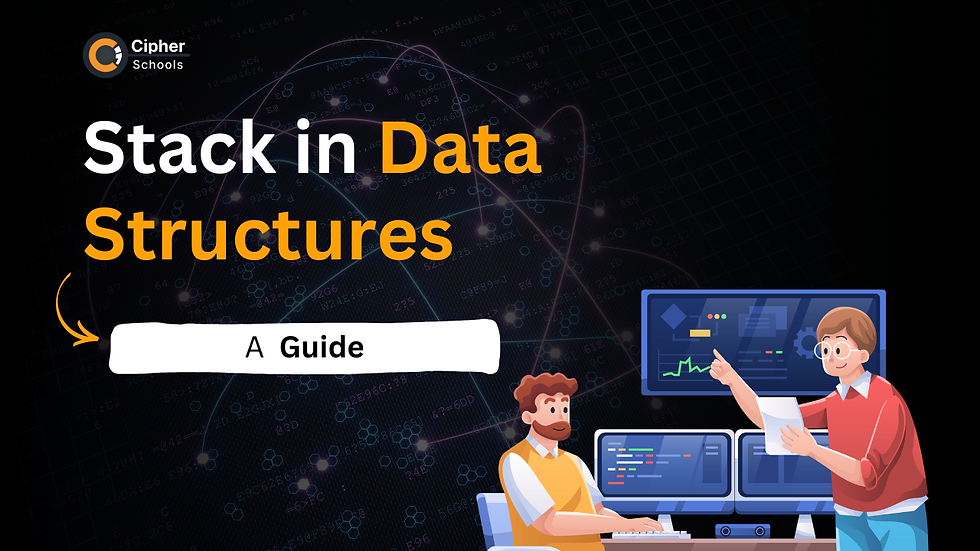
Introduction
Data structures are crucial in managing and organizing data effectively, and one of the most foundational ones is the stack. The stack in the data structure operates on a Last-In-First-Out (LIFO) principle, meaning the last item added is the first to be removed. This simple yet powerful structure has various applications in everyday programming and complex algorithm design.
What is a Stack in Data Structure?
A stack is a linear data structure where elements are added and removed from the same end, known as the "top" of the stack. Two primary operations define how stacks work:
Push: Adds an element to the top of the stack.
Pop: Removes the topmost element from the stack.
This LIFO principle makes stacks useful in scenarios where the most recent data needs to be accessed first.
Code Example: Basic Stack Operations in C++
#include<iostream>
#include<stack>
using namespace std;
int main() {
stack<int> s; // Create a stack of integers
// Push elements onto the stack
s.push(10);
s.push(20);
s.push(30);
// Pop the top element
cout << "Popped element: " << s.top() << endl;
s.pop();
// Display the current top element
cout << "Current top element: " << s.top() << endl;
return 0;
}
Applications of Stack
Stacks have numerous real-world applications:
Function Call Management: When a function is called, it gets pushed onto the stack. It is popped off when it completes, allowing nested function calls to be managed easily.
Undo Mechanism: In applications like text editors, each action is pushed onto a stack, enabling users to undo their actions step-by-step.
Expression Evaluation: Stacks are essential for converting infix expressions to postfix and for evaluating those expressions efficiently in compilers and calculators.
Implementation of Stack
Stacks can be implemented in two primary ways:
Array-Based Stack: A fixed-size stack where elements are stored in a continuous memory block. It's simple but has a predefined size limit.
Linked List-Based Stack: A dynamic stack where each element points to the next, making it flexible in size.
Conclusion
The stack in the data structure is an indispensable tool for managing data in various programming scenarios. Whether it's managing function calls, providing undo options, or evaluating expressions, stacks simplify complex operations. Exploring stack implementation is a great step toward mastering fundamental data structures.
Comments