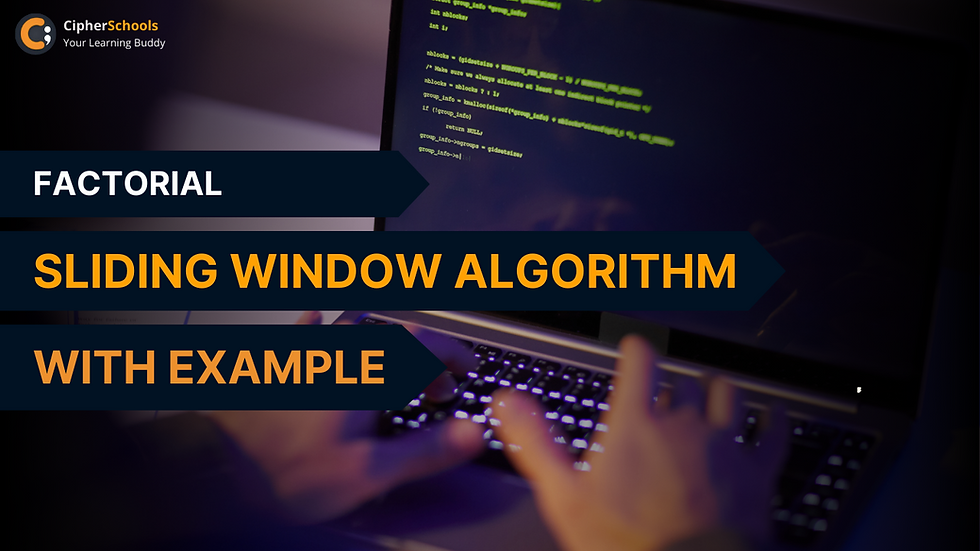
The Sliding Window Algorithm is a powerful technique used in computer science to solve various problems efficiently. It involves defining a window of a specific size that moves across an array or a sequence of elements. This window helps condense two nested loops into a single loop, reducing the time complexity of algorithms.
How to use Sliding Window Technique with Example?
Let's understand the Sliding Window Algorithm with an example. Consider a problem where we need to find the maximum sum of a subarray of size k in an array of integers. We can efficiently solve this problem using the sliding window technique.
public int maxSubArray(int[] nums, int k) {
int maxSum = Integer.MIN_VALUE;
int currentSum = 0;
for (int i = 0; i < nums.length; i++) {
currentSum += nums[i];
if (i >= k - 1) {
maxSum = Math.max(maxSum, currentSum);
currentSum -= nums[i - (k - 1)];
}
}
return maxSum;
}
In this example, we maintain a window of size k and move it across the array. At each step, we calculate the sum of elements within the window. If the window size exceeds k, we slide the window by subtracting the element at the beginning of the window.
How to Solve a Problem With the Sliding Window Algorithm?
To solve a problem using the Sliding Window Algorithm, follow these basic steps:
Define the problem and understand the requirements.
Determine the size of the window and initialize any necessary variables.
Iterate through the array or sequence, updating the window at each step.
Process the elements within the window to solve the problem.
Adjust the window as needed based on the problem constraints.
Continue iterating until the end of the array or sequence is reached.
To identify problems suitable for the Sliding Window Algorithm, look for the following characteristics:
The problem involves finding a subarray, substring, or subsequence with certain properties.
The problem requires iterating over the array or sequence while maintaining a window of fixed or variable size.
The problem can be optimized by avoiding redundant computations using the sliding window technique.
Fixed Size Sliding Window
In some cases, the window size remains fixed throughout the iteration process. This is known as a fixed-size sliding window. Problems involving fixed-size windows often require efficiently processing elements within the window to solve the problem.
public class FixedSizeSlidingWindow {
public static int[] findMaxSumSubarray(int[] nums, int k) {
int n = nums.length;
if (n == 0 || k <= 0 || k > n) {
return new int[0];
}
int[] result = new int[n - k + 1];
int windowSum = 0;
// Calculate the sum of the first window
for (int i = 0; i < k; i++) {
windowSum += nums[i];
}
result[0] = windowSum;
// Slide the window and update the result array
for (int i = 1; i <= n - k; i++) {
windowSum = windowSum - nums[i - 1] + nums[i + k - 1];
result[i] = windowSum;
}
return result;
}
public static void main(String[] args) {
int[] nums = {1, 3, -1, -3, 5, 3, 6, 7};
int k = 3;
int[] maxSumSubarray = findMaxSumSubarray(nums, k);
System.out.println("Max sum subarrays of size " + k + ":");
for (int num : maxSumSubarray) {
System.out.print(num + " ");
}
}
}
In this code:
The findMaxSumSubarray method takes an array nums and an integer k representing the size of the sliding window. It returns an array containing the maximum sum of subarrays of size k.
We first calculate the sum of the first window of size k.
Then, we slide the window by subtracting the element leaving the window and adding the new element entering the window. We update the result array with the sum of each window.
Finally, we return the result array containing the maximum sum of subarrays of size k.
You can test this code with different inputs to find the maximum sum subarrays of different sizes in the given array.
Variable Size Sliding Window Problem
In other cases, the window size may vary based on certain conditions or constraints. This is known as a variable-size sliding window. Problems involving variable-size windows often require dynamically adjusting the window size to meet the problem requirements.
public class VariableSizeSlidingWindow {
public static int[] findMaxSumSubarray(int[] nums, int target) {
int n = nums.length;
if (n == 0) {
return new int[0];
}
int start = 0, end = 0;
int windowSum = 0;
int maxSum = Integer.MIN_VALUE;
while (end < n) {
windowSum += nums[end];
while (windowSum >= target) {
maxSum = Math.max(maxSum, windowSum);
windowSum -= nums[start];
start++;
}
end++;
}
return new int[] { maxSum };
}
public static void main(String[] args) {
int[] nums = { 1, 4, 20, 3, 10, 5 };
int target = 33;
int[] maxSumSubarray = findMaxSumSubarray(nums, target);
System.out.println("Maximum sum subarray with sum >= " + target + ": " + maxSumSubarray[0]);
}
}
In this code:
The findMaxSumSubarray method takes an array nums and an integer target representing the target sum. It returns an array containing the maximum sum subarray with a sum greater than or equal to the target.
We use two pointers, start and end, to define the window boundaries.
We move the end pointer forward, adding elements to the window until the sum of the window is greater than or equal to the target.
Once the sum exceeds the target, we update the maximum sum if necessary and shrink the window by moving the start pointer forward until the sum is less than the target.
We continue this process until the end pointer reaches the end of the array.
Finally, we return the maximum sum subarray found.
You can test this code with different inputs to find the maximum sum subarray with a sum greater than or equal to the target value. Adjust the target variable to find subarrays with different target sums.
Conclusion
The Sliding Window Algorithm is a versatile technique used to solve a wide range of problems efficiently. By defining a window of a specific size and moving it across an array or sequence, we can optimize algorithms and solve complex problems with ease.
FAQs
What is a sliding window algorithm?
A sliding window algorithm is a technique used to efficiently solve problems involving arrays or sequences by defining a window of a specific size that moves across the data set.
What is the mechanism of sliding window?
The sliding window mechanism involves maintaining a window of fixed or variable size while iterating over an array or sequence. At each step, the window is updated, and elements within the window are processed to solve the problem.
What are the two types of sliding windows?
The two types of sliding windows are fixed-size sliding windows, where the window size remains constant, and variable-size sliding windows, where the window size can vary based on certain conditions or constraints.
Comments