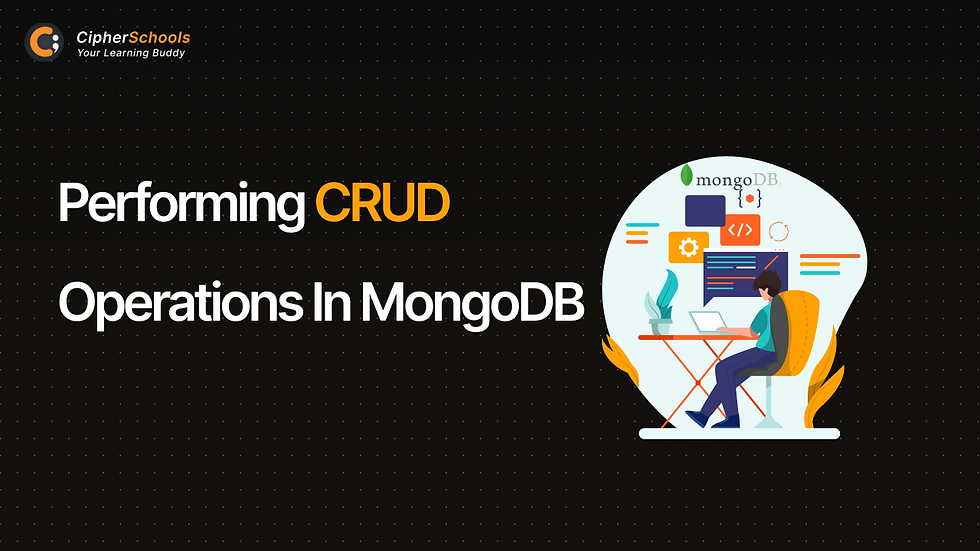
If you want to learn MERN Stack development then databases are the foundational components that power modern applications. I.T. teams are transitioning to JSON-based document databases like MongoDB to minimize complexity and remain agile since they allow them to accommodate constant changes in their data structures.
As we know, we can use MongoDB for various purposes, such as building an application (including web and mobile), data analysis, or as an administrator of a MongoDB database. In all of these cases, we must interact with the MongoDB server to perform specific operations such as entering new data into the application, updating data in the application, deleting data from the application, and reading the data. MongoDB provides basic yet fundamental activities known as CRUD operations, allowing you to interact with the MongoDB server quickly.
In this post, you will master the fundamentals of MongoDB CRUD operations. This tutorial will help you manage your MongoDB instance (a modern NoSQL database with a flexible JSON-like data model, sophisticated querying, and indexing).
What is the structure of MongoDB?
The best place to begin is to compare MongoDB to a typical RDBMS. The idea behind MongoDB is, to begin with a server that can host several databases. MongoDB, on the other hand, stores data as collections of documents instead of an SQL database, which saves data in tables and rows. So you get collections beneath your database and papers under your collections. Fields in documents are just key/value pairs.
Because JSON documents feature embedded fields, related data and lists of data can be kept as arrays/lists within the document rather than in an external table. As a result, developers may evaluate data associations without disseminating data across tables and conducting joins, allowing them to work fast and efficiently. Consider the following tabular format to demonstrate this concept:
From the above example, it is clear that there are fields in this table that contain many values and would be difficult to query or show if modeled in a single column (for example, size and tags). You may need to model your data differently in an RDBMS by building a relational table for those columns.
A MongoDB document, on the other hand, may address the same problem in the following way:
{
"name": "postcard",
"qty": 17,
"rating": 10,
"size": {"height": 10, "width": 5, "unit": "cm"},
"status": "P",
"tags": ["double-sided", "blue"]
}
What are MongoDB CRUD Operations?
MongoDB CRUD Operations are the storage management operations exposed by MongoDB. The abbreviation CRUD stands for Create, Read, Update, and Delete. You can use these four fundamental techniques to view, search, and change resources in your database.
The following is a summary of what each operation does:
Create: Create is the operation to insert or add new documents to the database.
Read: This action is used to view or retrieve documents from a database.
Update: When changing existing documents, this operation should be utilized.
Delete: As the name implies, the delete operation removes documents from the database.
These four CRUD actions constitute the fundamental communication processes with your MongoDB server.
How Do I Use MongoDB for CRUD Operations?
Now that you've connected to your MongoDB database and understand how it works and what CRUD operations with MongoDB are let's look at how to manipulate documents in a MongoDB database using CRUD methods.
Let's look at how to create, read, update, and delete documents on a MongoDB server.
Prerequisites
You will need the following items to follow along:
MongoDB is already installed on your server.
This guide will assume a basic understanding of the MongoDB shell.
Before You Start
Use the MongoDB shell to connect to your MongoDB server.
Use the use database_name> command to create a new MongoDB database or utilize an existing database.
MongoDB CRUD Operations: Create Operations
Create is the first of the CRUD Operations with MongoDB. The following are the most significant commands to use while creating documents in a MongoDB collection:
insertOne()
insertMany()
1. insertOne()
The insertion () command inserts one data object into the collection simultaneously. It accepts options in key/value pairs separated by commas, establishing the structure. Collections are formed implicitly in MongoDB; thus, they will be created if one does not exist.
You'll be working with a collection called "BooksDB" in this example. The insertion () method can insert a single entry into the BooksDB collection. Execute the following command in the MongoDB shell:
db.BooksDB.insertOne({
title: "The Innovator's Dilemma ",
author: "Clayton Christensen",
ISBN: 1250819997,
price: 18.65,
available: true
})
If the create operation is done successfully, a new document is created, and the output indicates it was successfully done. MongoDB will also produce a unique ObjectId for the new document automatically.
db.BooksDB.insertOne({
title: "The Innovator's Dilemma ",
author: "Clayton Christensen",
ISBN: 1250819997,
price: 18.65,
available: true})
})
{
"acknowledged": true,
"insertedId" : ObjectId("5fd989674e6b9ceb8665c57d")
}
2. insertMany()
Inserting several documents one at a time can soon become a laborious operation, especially with large datasets. As a result, MongoDB has the insertMany() method for inserting numerous documents at once. To do this, use square brackets ([and]) within the brackets to indicate that you are giving an array of several documents to the collection. This is known as nesting, and commas separate the documents.
Db.BooksDB.insertMany([{
title: "The Soul of a New Machine ",
author: "Tracy Kidder",
ISBN: 1250819997,
price: 8.00,
available: true},
{title: "The New New Thing",
author: "Michael Lewis ",
ISBN: 0062405802,
price: 38.45,
available: true},
{title: "The Age of A.I",
author: "Henry Kissinger",
ISBN: 0062405803,
price: 15.75,
available: false}])
Output:
This is how the output looks:
db.BooksDB.insertMany([{ title: " The Soul of a New Machine author: " Tracy Kidder ", ISBN: 1250819997,
price: 8.00, available: true}, {title: "The New New Thing ", author: "Michael Lewis ",
ISBN: 0062405802, price: 38.45, available: true}, {title: "The Age of A.I.A.I.", author: "Henry Kissinger", ISBN: 0062405803, price: 15.75, available: false}])
{
"acknowledged": true,
"insertedIds": [
ObjectId("5fd98ea9ce6e8850d88270b4"),
ObjectId("5fd98ea9ce6e8850d88270b5"),
"ObjectId("5fd98ea9ce6e8850d88270y3",
]
}
MongoDB CRUD Operations: Read Operations
The next operation in MongoDB CRUD Operations is Read. They are used to find papers in a collection. MongoDB CRUD provides the following methods for performing read operations:
find()
findOne()
Let's take a closer look at each method.
1. find()
Use the find() method on your desired collection to get all the documents from a collection. Executing the find() function without any arguments returns all records in the collection.
db.BooksDB.find()
Output:
This is how the output looks:
{"_id": "ObjectId("5fd98ea9ce6e8850d88270b4")", "title": "The Soul of a New Machine author", "author": "Tracy Kidder", "isbn": 1250819997,
"price": 8.00, "available': true}
{"_id": "ObjectId("5fd98ea9ce6e8850d88270b5")", "title": "The New New Thing", "author": "Michael Lewis",
"isbn": 0062405802, "price": 38.45, "available": true}
{"_id": "ObjectId("5fd98ea9ce6e8850d88270y3")", "title": "The Age of A.I.A.I.", "author": "Henry Kissinger",
"isbn": 0062405803, "price": 15.75, "available": false}
As shown in the output, MongoDB allocated a "_id" key to each record corresponding to a "ObjectId" value.
When executing reads, you can filter the results by key/value pair to find a more specific subsection of your information.
db.BooksDB.find({"title":" The New New Thing"})
{"_id": "ObjectId("5fd98ea9ce6e8850d88270b5")", "title": "The New New Thing ", "author": "Michael Lewis ",
"isbn": 0062405802, "price": 27.99, "available": true}
2. findOne()
The findOne() method can retrieve only one record that meets certain search parameters.
If you have more than one record that matches the query, the findOne() method may return just one result based on the order in which the data were created. The syntax for the function is as follows.
db.{collection}.findOne({query}, {projection})
For instance, consider the following query:
{"_id": "ObjectId("5fd98ea9ce6e8850d88270b4")", "title": "The Soul of a New Machine author", "author": "Tracy Kidder", "isbn": 1250819997,
"price": 8.00, "available': true}
MongoDB CRUD Operations: Update Operations
The update is the third operation in the CRUD Operations with MongoDB. It is typical for the records in your database to alter over time. A user, for example, may change their login, email address, credit card information, and so on. Other times, you may need to adapt the data structure to keep up with changing application requirements.
MongoDB supports update operations, allowing users to change field values at the document level and add new fields to documents in a collection. An update process typically includes a filter to choose the documents to be updated and an action. Because updates cannot be undone, you must proceed cautiously when installing them.
MongoDB offers two ways to update documents:
updateOne()
updateMany()
1. updateOne()
You can use the update () function on a collection to update a single document. You must give a filter as an argument and an update action to update a single document in the BooksDB collection.
The update filter indicates which items should be updated, and the action specifies how the items should be updated. Let's try changing the author's name from Tracy Kidder to Stacey. Use the updateOne() function to update a single document to accomplish this:
db.BooksDB.updateOne({author: " Tracy Kidder "}, {$set:{author: "Stacey"}})
Output:
This is how the output looks:
{"acknowledged": true, "matchedCount": 1, "modifiedCount": 1 }
{"_id": "ObjectId("5fd98ea9ce6e8850d88270b4")", "title": "The Soul of a New Machine author ", "author": "Stacey ", "isbn": 1250819997,
"price": 8.00, "available': true}
2. updateMany()
MongoDB CRUD operations offer the updateMany() method, which allows application developers to update many documents by sending in a list of objects, much as adding multiple documents. It differs from the update () method, which updates only the first document that fits the filter criteria. The syntax of the updateMany() function is the same as that of the update () method.
db.BooksDB.updateMany({author:" Michael Lewis "}, {$set: {author: " Robert Cargill"}})
Output:
This is how the output looks:
{"acknowledged": true, "matchedCount": 2, "modifiedCount": 2 }
MongoDB CRUD Operations: Delete Operations
Delete is the final operation in CRUD Operations with MongoDB. Delete procedures affect only one document. To delete a document from a collection, you must first apply a filter to the item. The syntax for delete is similar to that of read operations.
MongoDB's CRUD Operations give two methods for deleting records from a collection:
deleteOne()
deleteMany()
1. deleteOne()
To delete a document from a collection, utilize the delete () method of Crud operations with MongoDB. Normally, a filter is necessary to choose the item you wish to delete. Only the first document that matches the criterion is usually destroyed.
db.BooksDB.deleteOne({name:" Robert Cargill"})
Output:
This is how the output looks:
{"acknowledged": true, "deletedCount": 1 }
2. deleteMany()
MongoDB CRUD has the deleteMany() method for deleting multiple documents from a collection that matches a filter. This function deletes several documents from a specified collection with a single delete action. To delete all books by the author Christopher, for example, the syntax is identical to the deleteOne() method:
db.BooksDB.deleteMany({author:" Robert Cargill"})
Output:
This is how the output looks:
{"acknowledged": true, "deletedCount": 2 }
Conclusion
Congratulations on making it to the end. This article examined MongoDB's architecture, described CRUD operations with MongoDB, and provided examples of how to use the CRUD operations with MongoDB. It would help if you now had a solid understanding of utilising these concepts in your MongoDB project.
However, as a Developer, pulling sophisticated data from a wide range of data sources such as databases, CRMs, project management tools, streaming services, and marketing platforms to your MongoDB database is daunting.
Interesting F.A.Q.s
Is it necessary to do crud operations?
According to Atatus, CRUD activities are essential for any online application interacting with data. You may conduct the most common data management tasks with CRUD operations, such as adding new records, obtaining existing records, updating or altering records, and deleting records.
What are the seven components of CRUD?
Hackermoon says there are four separate CRUD processes and seven RESTful actions. CRUD actions are Create, Read, Update, Edit, and Delete. Index, New, Show, Edit and Edit, and Delete are all stored in R of CRUD. Your activities decide whether you consume or feed data; everyone else provides it.
Stay tuned to CipherSchools for more interesting tech articles.
Comments