Parameterized Constructor in Java
- Pushp Raj
- May 10, 2024
- 3 min read
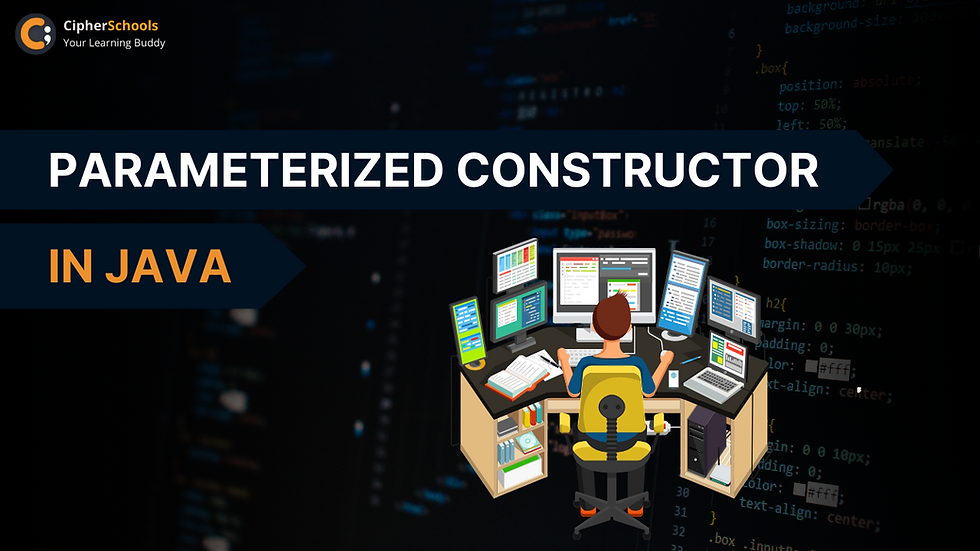
Learn Parameterized Constructors in Java with Examples
Parameterized constructors in Java play a crucial role in initializing objects with custom values, adding flexibility to Java programming. This comprehensive guide delves into the concept of parameterized constructors, providing insights, examples, and practical applications to enhance your Java skills.
What is a Parameterized Constructor in Java with Example?
A parameterized constructor in Java is a special type of constructor that accepts parameters, allowing developers to initialize objects with specific values during instantiation. Through examples, we'll explore how parameterized constructors enhance object initialization in Java programming.
public class Student {
private String name;
private int age;
// Parameterized Constructor
public Student(String name, int age) {
this.name = name;
this.age = age;
}
// Getter methods
public String getName() {
return name;
}
public int getAge() {
return age;
}
}
public class Main {
public static void main(String[] args) {
// Creating objects using parameterized constructor
Student student1 = new Student("John", 20);
Student student2 = new Student("Alice", 22);
// Accessing object properties
System.out.println("Student 1: Name - " + student1.getName() + ", Age - " + student1.getAge());
System.out.println("Student 2: Name - " + student2.getName() + ", Age - " + student2.getAge());
}
}
Uses of Parameterized Constructors in Java:
Parameterized constructors offer versatility and customization in object creation, facilitating tailored initialization based on input parameters. We'll discuss various scenarios and applications where parameterized constructors prove invaluable in Java programming.
Types of Constructors in Java:
We'll elucidate the different types of constructors in Java, including default constructors, parameterized constructors, and constructor overloading. Understanding these constructor types is essential for mastering object initialization in Java.
Difference Between Default Constructor and Parameterized Constructor in Java:
Differentiating between default constructors and parameterized constructors is crucial for Java developers. We'll dissect the distinctions, highlighting their respective roles, syntax, and applications in Java programming.
Advantages of Parameterized Constructor in Java:
Parameterized constructors offer several benefits, such as enhanced object initialization, improved code readability, and increased flexibility. We'll delve into these advantages, showcasing how parameterized constructors streamline Java programming.
Default Constructor in Java Examples:
While parameterized constructors provide custom initialization, default constructors serve as a fallback option. We'll provide examples demonstrating default constructor usage and its role in object initialization.
Constructor Overloading in Java:
Constructor overloading enables Java developers to define multiple constructors with different parameter lists. We'll explore how constructor overloading enhances code reusability and flexibility in Java programming.
Error Thrown by Default Constructor in Java:
In scenarios where a default constructor is expected but not provided, Java compilers throw errors. We'll discuss common errors related to default constructor absence and strategies to resolve them.
Conclusion:
Parameterized constructors play a pivotal role in Java programming, offering flexibility, customization, and improved object initialization. By mastering parameterized constructors, developers can elevate their Java skills and build robust, efficient applications.
FAQs:
How can I write a parameterized constructor code in Java?
To write a parameterized constructor in Java, you need to define a constructor method within a class that accepts parameters. Here's an example:
public class MyClass {
private int number;
// Parameterized constructor
public MyClass(int num) {
this.number = num;
}
}
What is a parameterized constructor called?
A parameterized constructor in Java is called a constructor with parameters. It allows you to initialize objects with specific values during instantiation by passing parameters to the
constructor.
What is a default parameterized constructor?
A default constructor in Java is a constructor with no parameters. It is automatically provided by the compiler if no other constructors are explicitly defined in the class. A parameterized constructor, on the other hand, is a constructor with parameters, allowing for custom initialization of objects.
Commenti