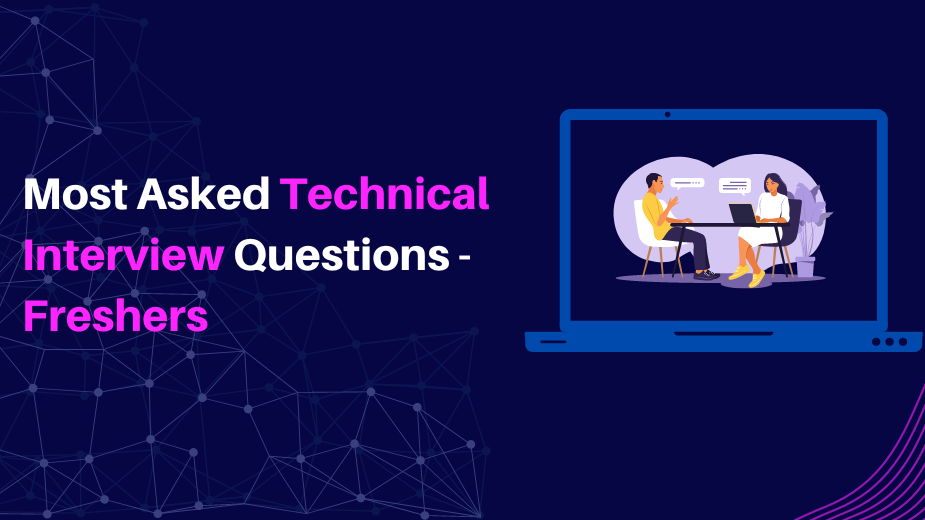
Common Technical Interview Questions
Most commonly asked campus placement technical interview questions, mostly asked by the companies like Infosys, Capgemini, Cognizant, Capgemini, DXC, Accenture etc.
1. Explain four major OOP concepts
Abstraction: Abstraction is the process of hiding complex implementation details and exposing only the essential features of an object to the outside world. This allows the object to be viewed as a simplified model of a real-world entity, making it easier to understand and use.
Inheritance: Inheritance is a mechanism that allows an object to inherit properties and behaviour from a parent object. This allows for code reuse and helps to eliminate redundant code.
Polymorphism: Polymorphism is the ability of an object to take on multiple forms. In OOP, polymorphism allows objects of different classes to be treated as objects of a common class, making it possible to write code that can handle objects of different types in a generic manner.
Encapsulation: Encapsulation is the process of wrapping data and behaviour within an object, making it protected from external access and modification. This helps to maintain the integrity of the data and ensure that objects can only be modified in a controlled and predictable manner.
2. Can we implement multiple inheritances in java?
No, Java does not support multiple inheritances. Java uses a single inheritance model, where a class can only inherit from one parent class.
3. What are classes and objects in C++?
Classes and objects are fundamental concepts in object-oriented programming (OOP), and they are also important in the C++ programming language.
A class in C++ is a blueprint or a template for creating objects. It defines a set of attributes and behaviors that describe the properties and actions of an object of that class.
An object is an instance of a class. It is a unique, individual representation of the class, and it contains its own values for the attributes defined in the class.
4. What is operator overloading?
Operator overloading is a feature in object-oriented programming that allows a programmer to redefine the behavior of an operator for a user-defined data type. For example, the + operator can be overloaded to perform addition on two objects of a user-defined type.
5. What is the difference between struct and class?
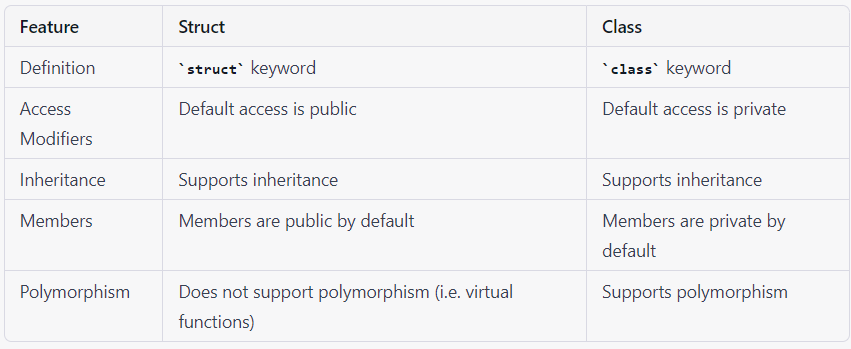
6. What is a virtual function?
A virtual function is a member function in an object-oriented programming language, such as C++ or Java, that is declared with the virtual keyword. Virtual functions allow for dynamic dispatch, which means that the function to be called is determined at runtime rather than at compile time.
The purpose of virtual functions is to provide a mechanism for creating objects that have a base type but can be treated as objects of derived types. Virtual functions enable you to write generic code that works with objects of different types, as long as they are related through inheritance.
7. Explain inheritance.
Inheritance is a concept in object-oriented programming (OOP) where a subclass or derived class inherits the attributes and behavior of its parent or base class. This allows for code reusability and helps in creating a hierarchy of classes, where the subclass can inherit properties and methods of its parent class and add its own unique properties and methods.
There are several types of inheritance in object-oriented programming, including:
Single Inheritance: This type of inheritance occurs when a subclass inherits from a single base class.
Multiple Inheritance: This type of inheritance occurs when a subclass inherits from multiple base classes. This is not supported by many object-oriented programming languages, including Java and C#, due to the ambiguity that can occur when multiple base classes have methods with the same name.
Hierarchical Inheritance: This type of inheritance occurs when a single base class is inherited by multiple subclasses.
Multi-level Inheritance: This type of inheritance occurs when a subclass inherits from a class that is itself a subclass of another class.
Hybrid Inheritance: This type of inheritance is a combination of multiple inheritance and hierarchical inheritance, where a subclass inherits from multiple base classes and also serves as a base class for other subclasses.
8. What are friend class and friend function?
In object-oriented programming, a friend class or friend function is a mechanism that allows a class or function to access the private or protected members of another class. This is done by declaring the class or function as a friend of the class whose members it needs to access.
A friend class is a class that is declared as a friend of another class. This means that the friend class has the ability to access the private and protected members of the class that it is declared a friend of.
Here is an example of a friend class in C++:
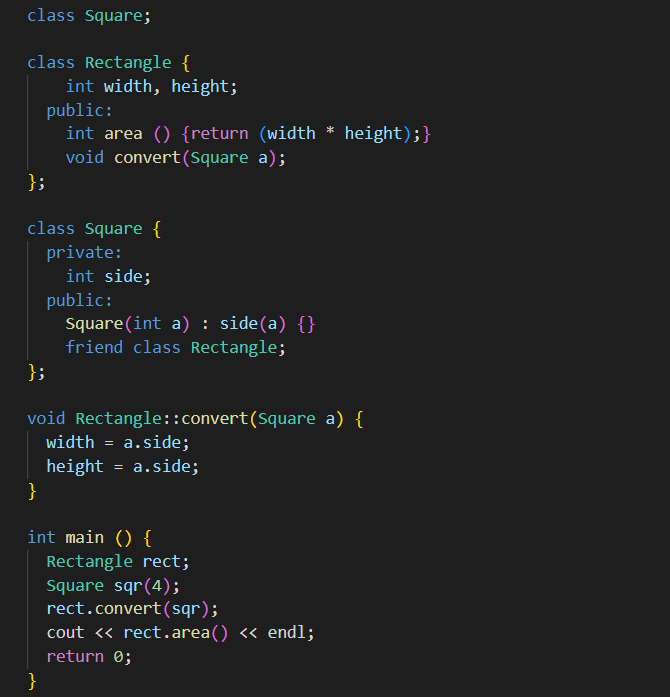
A friend function is a function that is declared as a friend of a class. This means that the friend function has the ability to access the private and protected members of the class that it is declared a friend of.
And here is an example of a friend function in C++:
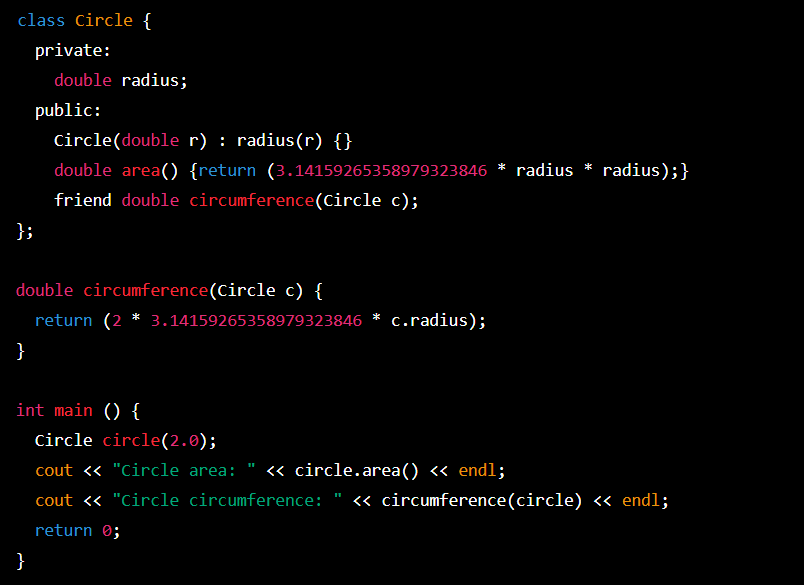
9. What are access specifiers?
Access specifiers are keywords used in object-oriented programming languages to control the visibility and accessibility of class members (such as variables and methods). They determine which class members can be accessed from outside the class and which members can only be accessed from within the class.
There are three main access specifiers in most object-oriented programming languages:
public: Members declared as public can be accessed from anywhere, both inside and outside the class.
private: Members declared as private can only be accessed from within the class. They are not accessible from outside the class, even by subclasses.
protected: Members declared as protected can be accessed from within the class and by subclasses, but not from outside the class hierarchy.
10. What is an inline function?
The inline function in C++ is an enhancement feature that improves the execution time and speed of the program.
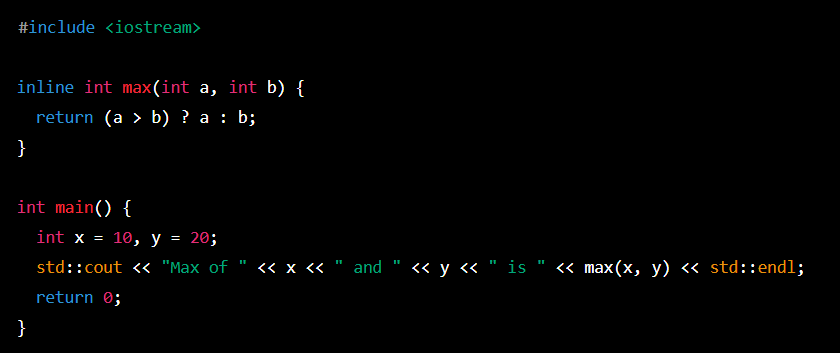
11. What are constructors and destructors in c++?
A constructor is a particular member function having the same name as the class name. It calls automatically whenever the object of the class is created.
There are four types of constructors used in C++.
Default constructor
Dynamic constructor
Parameterized constructor
Copy constructor
Destructors have the same class name preceded by (~) tilde symbol. It removes and destroys the memory of the object, which the constructor allocated during the creation of an object.
12. What are the static members and static member functions?
Static data members are class members that are declared using static keywords.
Static member functions in C++ are the functions that can access only the static data members.
13. What is an enum?
An enum is a special "class" that represents a group of constants (unchangeable variables, like final variables).
14. What are the different types of polymorphism in C++?
Types of Polymorphism
Compile-time Polymorphism - Compile-time polymorphism, also known as static polymorphism, is a type of polymorphism in C++ that allows you to use functions or operators in multiple ways. It is achieved through techniques such as function overloading, operator overloading, and template functions.
Runtime Polymorphism - Runtime polymorphism, also known as dynamic polymorphism, is a type of polymorphism in C++ that allows you to use objects of different classes in a polymorphic manner. It is achieved through virtual functions.
A virtual function is a member function in a base class that can be overridden in derived classes.
15. Define namespace in C++
In C++, a namespace is a container that holds a set of identifiers (such as variable names, function names, class names, etc.) and provides a way to organize code and prevent naming conflicts between different parts of a program.
16. What is the difference between an array and a list?
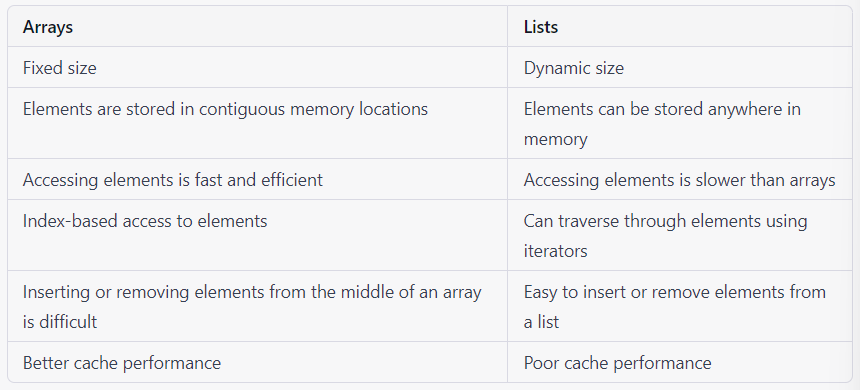
17. What is function overriding?
Function overriding is a feature in object-oriented programming (OOP) where a subclass provides a new implementation for a method that is already defined in its superclass. The new implementation in the subclass "overrides" the implementation of the method in the superclass. This allows the subclass to inherit the methods and fields from the superclass but provides a different implementation for certain methods.
Function overriding is achieved through polymorphism, which allows objects of different classes to be treated as objects of the same class.
18. What does the Scope Resolution operator do?
The scope resolution operator is a symbol used to specify which class a method or property belongs to when it is called from within another class. It is represented by two colons '::'.
In object-oriented programming languages, the scope resolution operator is used to access class-level variables, constants, and methods, including those defined in parent classes. This allows you to use a method or property from a parent class without having to instantiate an object of that class.
19. What is the difference between struct and class?
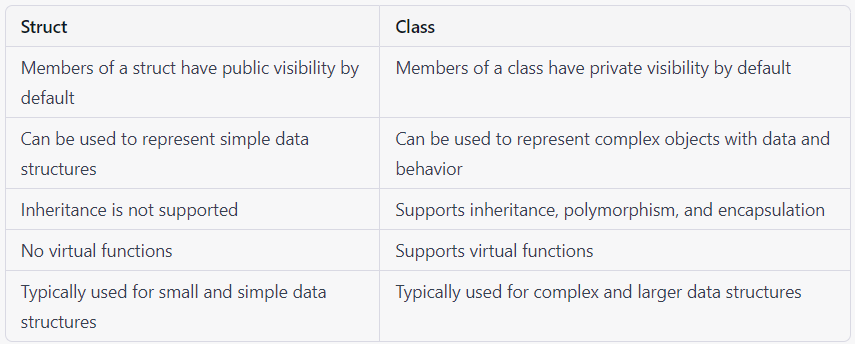
20. Can you compiler a program without the main function in java?
Yes, you can compile and execute without main method by using a static block.
21. Difference between compile time and runtime polymorphism.
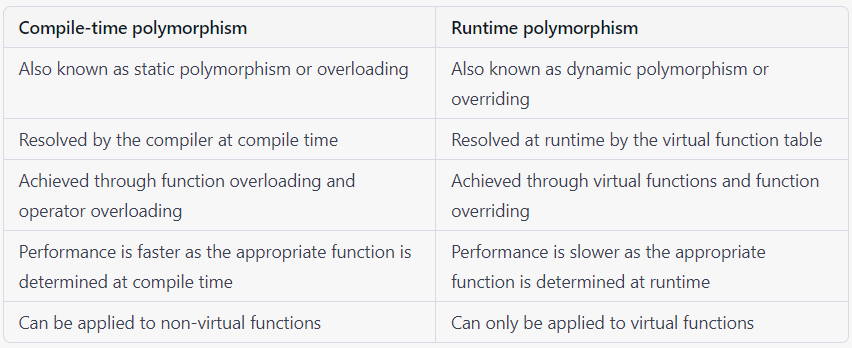
22. Is it possible to overload a deconstructor?
No, it is not possible to overload a destructor in most programming languages. A destructor is a special method in a class that is called when an object of that class is destroyed or goes out of scope. Most programming languages, including C++ and Java, only allow a single destructor to be defined for a class.
23. What is call by value and class by reference in C++?

24. Difference Between Java and C++
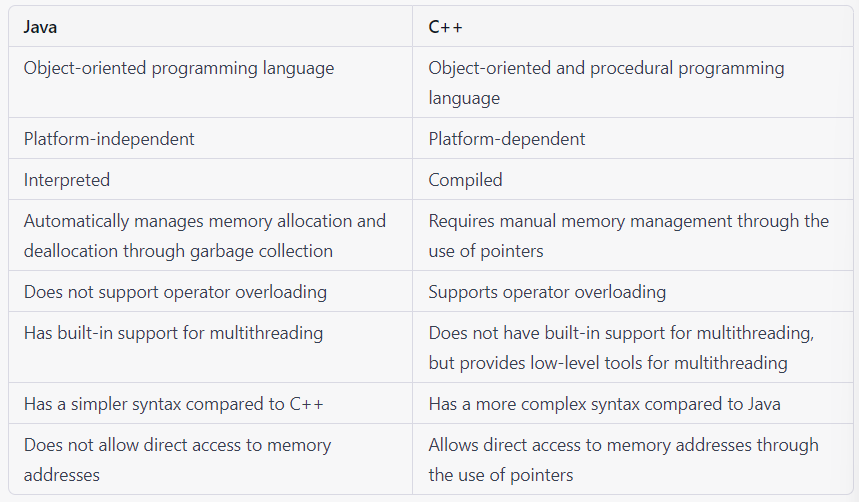
25. What is the difference between JDK, JRE, and JVM?
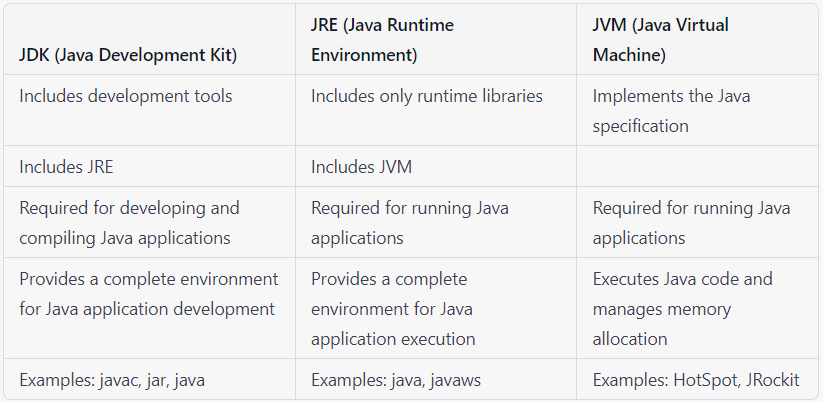
26. What Gives Java its 'write once and run anywhere' nature?
The "write once, run anywhere" (WORA) nature of Java is due to the Java Virtual Machine (JVM). The JVM is a software platform that runs on a variety of hardware platforms and operating systems. When you write a Java program, it is compiled into bytecode, which is a machine-independent format. The bytecode is then interpreted by the JVM, which executes the code on the specific hardware and operating system where it is running.
27. Difference between a constructor and a method
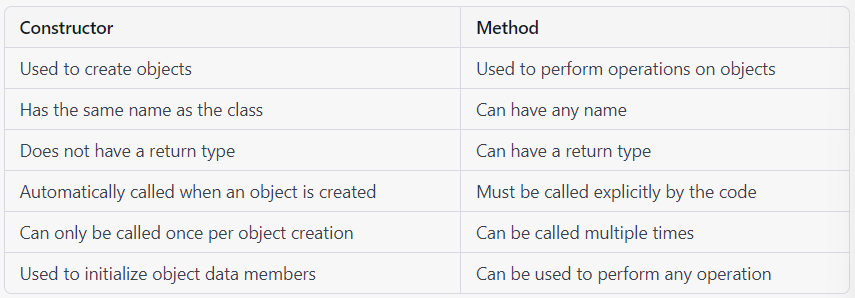
28. What is 'this' keyword in java?
The 'this' keyword in Java is a reference to the current object of a class. It is used to refer to the instance variables and instance methods of the current object.
29. Why does Java not support pointers?
Java does not support pointers because they can cause security and reliability issues. Pointers are a powerful feature of C and C++, but they also allow direct memory access, which can lead to a number of security and reliability problems.
For example, incorrect use of pointers can result in memory leaks, buffer overflows, and access to uninitialized memory. These types of bugs can be difficult to detect and can result in unpredictable behavior, crashes, and even security vulnerabilities.
30. What is super in Java?
In Java, the super keyword is used to refer to the parent class or the superclass of an object. It can be used to access the members of the parent class, including instance variables, instance methods, and constructors.
There are two common uses for the super keyword:
To access the instance variables and instance methods of the parent class: If a subclass needs to access the instance variables or instance methods of its parent class, it can use the super keyword to do so.
To call the constructor of the parent class: If a subclass needs to call the constructor of its parent class, it can use the super keyword followed by the arguments for the constructor. This is useful when a subclass needs to perform some additional initialization that is not handled by the parent class constructor.
31. Difference between this and super keyword
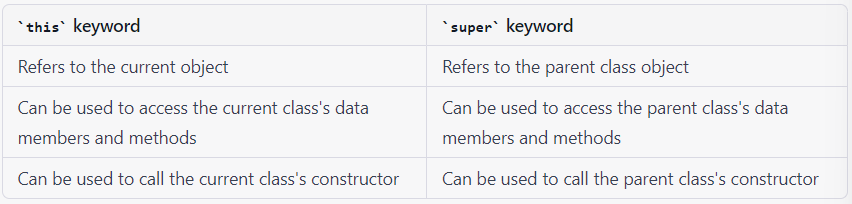
32. What is the final variable?
In Java, a final variable is a variable that can be assigned a value only once. Once a final variable has been assigned a value, its value cannot be changed. final variables are useful in a variety of situations, including:
Constant values: You can use the final keyword to define constant values that cannot be changed.
Immutable objects: By declaring an object reference as final, you can prevent it from being re-assigned to a different object.
33. What is exceptional handling in java?
Exception Handling is a mechanism to handle runtime errors such as ClassNotFoundException, IOException, SQLException, RemoteException, etc.
34. Why is Java not a pure object-oriented language?
A fully object-oriented language needs to have all 4 oops concepts. In addition to that, all predefined and, user-defined types must be objects, and, all the operations should be performed only by calling the methods of a class.
Though java follows all four object-oriented concepts,
Java has predefined primitive data types (which are not objects).
You can access the members of a static class without creating an object of it.
Therefore, Java is not considered a fully object-oriented Technology.
35. What is a ClassLoader?
A ClassLoader is a Java class that is responsible for loading class files into the Java Virtual Machine (JVM) at runtime. When a Java program is executed, the JVM loads the required classes into memory, and the ClassLoader is responsible for finding and loading those classes.
In Java, there are several built-in ClassLoaders, including the bootstrap ClassLoader, the extension ClassLoader, and the system ClassLoader. Each ClassLoader has a different responsibility and a different classpath, and the ClassLoaders work together to load classes into the JVM.
36. Difference between a String and a StringBuffer
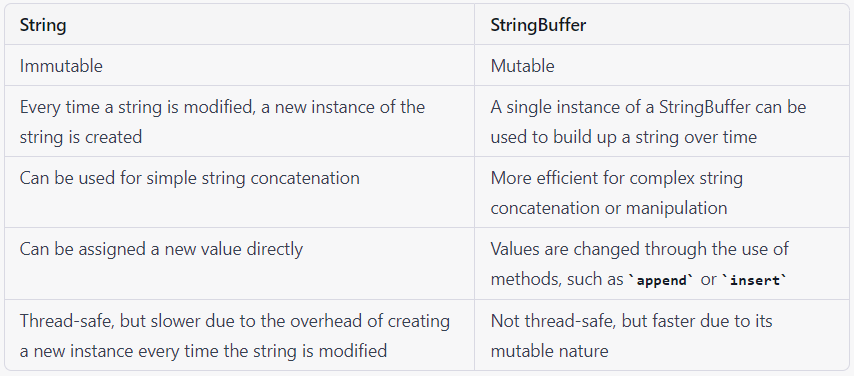
37. What are threads in Java?
A Thread is a very light-weighted process, or we can say the smallest part of the process that allows a program to operate more efficiently by running multiple tasks simultaneously.
38. Difference between the 'throw' and 'throws' keywords in java?

Opmerkingen