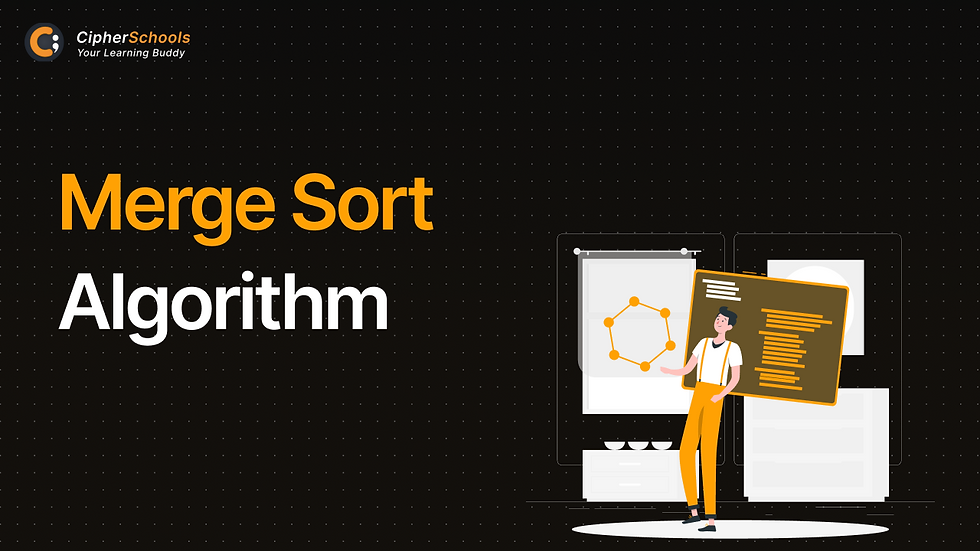
Introduction:
Merge Sort stands tall among sorting algorithms for its efficiency and simplicity. In this guide, we'll delve into the intricacies of Merge Sort, exploring its working principles, implementation details, time and space complexities, and practical applications. Whether you're a beginner or an experienced developer, understanding Merge Sort is crucial for mastering data structures and algorithms.
What is the Merge Sort Algorithm?
Merge Sort is a divide-and-conquer algorithm that divides the input array into smaller subarrays, sorts them recursively, and then merges the sorted subarrays to produce a fully sorted array. Its efficiency lies in its ability to efficiently handle large datasets and its consistent time complexity of O(n log n) for all cases.
How does the Merge Sort Algorithm work?
The Merge Sort Algorithm works by recursively dividing the input array into halves until each subarray contains only one element. Then, it merges adjacent subarrays in a sorted manner until the entire array is sorted. This process ensures that smaller subarrays are sorted before merging, resulting in a fully sorted array.
Why Merge Sort Algorithm Important?
Merge Sort is essential for its efficiency, stability, and scalability. It guarantees a worst-case time complexity of O(n log n) and is well-suited for sorting large datasets efficiently. Additionally, its stability ensures that equal elements maintain their relative order after sorting, making it suitable for applications requiring stability.
The Concept Behind Merge Sort: Breaking Down and Conquering:
Merge Sort follows the "Divide and Conquer" paradigm, which involves breaking down a problem into smaller subproblems, solving them independently, and then combining the solutions to obtain the final result. This approach ensures efficient problem-solving by breaking down complex tasks into manageable chunks.
Advantages & Drawbacks of Merge Sort:
Merge Sort offers several advantages, including stable sorting, consistent time complexity, and suitability for large datasets. However, it also has drawbacks, such as its space complexity, as it requires additional memory for merging subarrays, and its recursive nature, which may lead to stack overflow for extremely large datasets.
Steps of Merge Sort in Divide and Conquer Algorithm:
Divide: Split the array into two halves recursively until each subarray contains only one element.
Conquer: Sort each subarray independently using Merge Sort.
Combine: Merge adjacent sorted subarrays to produce a fully sorted array.
Implementation of Merging Algorithm:
Here's a C++ implementation of the merging algorithm used in Merge Sort:
cpp
#include <iostream>
#include <vector>
void merge(std::vector<int>& arr, int l, int m, int r) {
int n1 = m - l + 1;
int n2 = r - m;
std::vector<int> L(n1), R(n2);
for (int i = 0; i < n1; i++)
L[i] = arr[l + i];
for (int j = 0; j < n2; j++)
R[j] = arr[m + 1 + j];
int i = 0, j = 0, k = l;
while (i < n1 && j < n2) {
if (L[i] <= R[j]) {
arr[k] = L[i];
i++;
} else {
arr[k] = R[j];
j++;
}
k++;
}
while (i < n1) {
arr[k] = L[i];
i++;
k++;
}
while (j < n2) {
arr[k] = R[j];
j++;
k++;
}
}
void mergeSort(std::vector<int>& arr, int l, int r) {
if (l < r) {
int m = l + (r - l) / 2;
mergeSort(arr, l, m);
mergeSort(arr, m + 1, r);
merge(arr, l, m, r);
}
}
int main() {
std::vector<int> arr = {12, 11, 13, 5, 6, 7};
int arrSize = arr.size();
mergeSort(arr, 0, arrSize - 1);
std::cout << "Sorted array: ";
for (int i = 0; i < arrSize; i++)
std::cout << arr[i] << " ";
std::cout << std::endl;
return 0;
}
Time Complexity Analysis in Merge Sort Algorithm:
The time complexity of Merge Sort is O(n log n) in all cases, making it one of the most efficient sorting algorithms available. This efficiency stems from its divide-and-conquer approach, which ensures that the array is divided into smaller subarrays logarithmically with each recursion level.
Space Complexity Analysis of Merge Sort Algorithm with Example:
The space complexity of Merge Sort is O(n) due to the additional memory required for merging subarrays. However, its recursive nature may lead to stack overflow for extremely large datasets, limiting its practical scalability.
Iterative Merge Sort Algorithm:
While Merge Sort is typically implemented recursively, it can also be implemented iteratively using a bottom-up approach. This approach iteratively merges sorted subarrays of increasing size until the entire array is sorted.
Important Points of the Merge Sorting Algorithm:
Merge Sort is a stable sorting algorithm that maintains the relative order of equal elements.
It guarantees a worst-case time complexity of O(n log n) and is well-suited for sorting large datasets efficiently.
Merge Sort requires additional memory for merging subarrays, leading to a space complexity of O(n).
Conclusion:
Merge Sort stands as a testament to the power of divide-and-conquer algorithms in efficiently solving complex problems. By understanding its principles, implementation details, and practical implications, you'll be equipped to tackle sorting challenges with confidence and efficiency.
FAQs:
Which sorting algorithm makes use of Merge Sort?
Merge Sort is used in various applications, including sorting large datasets efficiently and merging sorted sequences.
What is the merge insertion sort algorithm?
The merge insertion sort algorithm combines Merge Sort and Insertion Sort to achieve better performance for small subarrays by switching to Insertion Sort for arrays below a certain size.
Is Merge Sort classified as an in-place algorithm?
No, Merge Sort is not classified as an in-place algorithm due to its space complexity of O(n) for merging subarrays.
What practical applications benefit from the use of Merge Sort?
Merge Sort is widely used in various applications, including sorting databases, external sorting, and parallel computing, where efficient sorting of large datasets is essential.
Comments