
In Java, HashMap and HashTable are both data structures used for storing key-value pairs. While they serve similar purposes, there are significant differences between the two. In this guide, we'll explore the nuances of HashMap and HashTable, their syntax, behavior, and when to use each.
What is HashMap in Java with Examples?
HashMap is a part of the Java Collections Framework and implements the Map interface. It stores key-value pairs and allows null keys and values. Let's consider an example:
import java.util.HashMap;
public class HashMapExample {
public static void main(String[] args) {
HashMap<String, Integer> map = new HashMap<>();
map.put("apple", 10);
map.put("banana", 20);
map.put("orange", 30);
System.out.println(map.get("banana")); // Output: 20
}
}
What is Hashtable in Java with examples?
Hashtable is a legacy class in Java that also stores key-value pairs but predates the Collections Framework. It does not allow null keys or values. Here's an example:
import java.util.Hashtable;
public class HashTableExample {
public static void main(String[] args) {
Hashtable<String, Integer> table = new Hashtable<>();
table.put("apple", 10);
table.put("banana", 20);
table.put("orange", 30);
System.out.println(table.get("banana")); // Output: 20
}
}
Difference Between HashMap and HashTable:
Null Values: HashMap allows null keys and values, whereas Hashtable does not.
Synchronization: Hashtable is synchronized, making it thread-safe, while HashMap is not synchronized.
Performance: HashMap typically performs better than Hashtable in most scenarios.
Iteration: HashMap allows an iterator over its elements, while Hashtable uses an enumerator.
Inheritance: HashMap is part of the Java Collections Framework, while Hashtable is a legacy class.
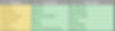
Explaining Why HashTable Doesn’t Allow Null and HashMap Do?
Hashtable is designed to be thread-safe by default, and allowing null keys or values could lead to synchronization issues. In contrast, HashMap prioritizes flexibility and allows null keys and values.
Difference Between Hashtable and ConcurrentHashMap in Java:
ConcurrentHashMap is an alternative to Hashtable that offers better scalability and performance in multi-threaded environments. It achieves this by partitioning the map into segments, reducing contention during concurrent access.
Program displaying HashMap:
import java.util.HashMap;
public class HashMapDemo {
public static void main(String[] args) {
HashMap<String, Integer> map = new HashMap<>();
map.put("apple", 10);
map.put("banana", 20);
map.put("orange", 30);
for (String key : map.keySet()) {
System.out.println(key + ": " + map.get(key));
}
}
}
Uses of HashMap and Hashtable in Java:
HashMap and Hashtable are commonly used for tasks like caching, memoization, and storing configuration settings. They are also suitable for implementing associative arrays and data caching.
Conclusion:
In summary, HashMap and Hashtable are both useful data structures for storing key-value pairs in Java, but they have distinct characteristics and use cases. Understanding their differences and when to use each can help developers make informed decisions in their projects.
FAQs:
Which is better HashMap Hashtable?
HashMap is generally considered better due to its better performance and flexibility. However, Hashtable may be preferred in scenarios requiring thread safety.
Which is faster: HashMap or Hashtable?
HashMap is typically faster than Hashtable due to its unsynchronized nature. Hashtable's synchronization can introduce overhead in multi-threaded environments.
What is some real-life example of HashMap?
A real-life example of HashMap usage is storing and retrieving user preferences in a web application. Each preference (key) is associated with a specific user (value).
Why HashMap is preferred in Java?
HashMap is preferred in Java for its performance, flexibility, and compatibility with the Java Collections Framework. It offers better scalability and allows null keys and values, making it suitable for a wide range of use cases.