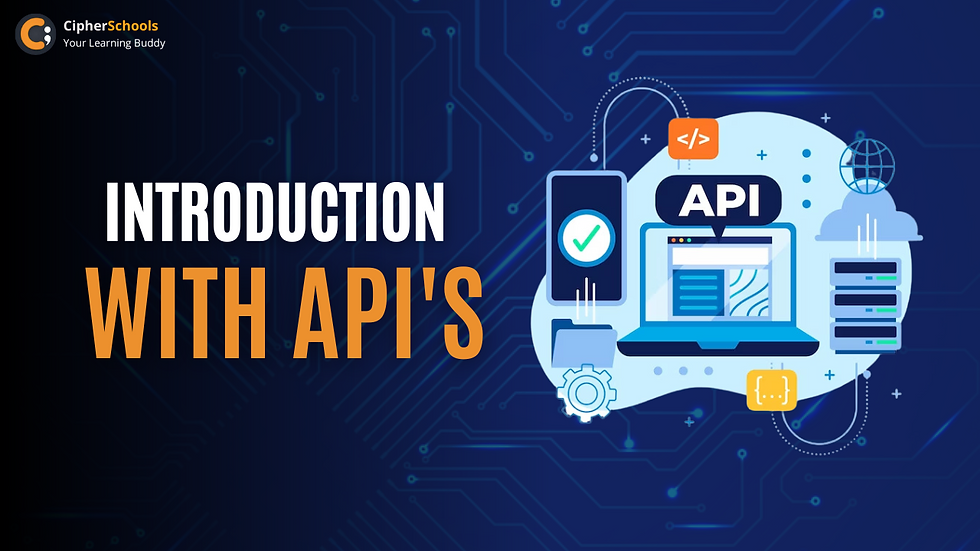
First, we'll take a high-level look at APIs: what they are, how they function, how to utilize them in your code, and how they are constructed. We'll also look at what the various API classes are and what kinds of usage they have.
A.P.I.s hide complexity from developers, extend systems to partners, arrange code, and make components reusable.
APIs are frequently conceived of as contracts, with documentation representing an agreement between parties: if one party sends a remote request organized in a certain way, the second party's program will answer similarly.
In this article "Introduction with APIs" we’ll learn about APIs, their functions, and how APIs can be created.
What exactly are APIs?
Application Programming Interfaces (APIs) are features made available in programming languages to enable developers to design complicated functionality more quickly. They abstract more difficult code away from you, replacing it with simpler syntax.
Consider the electricity supply in your home, flat or other residences. If you want to utilize an appliance in your home, plug it into a power outlet, and it will function. You don't try to hook it straight into the power source because it's inefficient, complicated, and dangerous if you're not an electrician.
Similarly, you want to program some 3D graphics. In that case, it is much easier to do so through an API written in a higher-level language such as JavaScript or Python than to write low-level code that directly controls the computer's GPU or other graphics functions.
A Short History
APIs first appeared in computing history long before the personal computer. An API was typically utilized as a library for operating systems at the time. Although it occasionally carried messages between mainframes, the API was usually local to the computers on which it functioned.
APIs emerged from their confines after roughly 30 years. They were becoming a key technology for distant data integration by the early 2000s.
Client-side JavaScript APIs
Client-side JavaScript has a plethora of APIs available; they are not built into the JavaScript language itself but instead on top of it, providing you with more superpowers to use in your JavaScript code. They are often classified into two types:
Browser APIs
Browser APIs are primarily built into your web browser. They can disclose data from the browser and surrounding computer environment and do complicated practical tasks. The Web Audio API, for example, includes JavaScript tools for editing audio in the browser, such as taking an audio track and changing its volume or applying effects to it.
The browser uses some complex lower-level code (e.g., C++ or Rust) to do the audio processing in the background. However, the API abstracts this complexity away from you.
Third-Party APIs
Third-party APIs are not incorporated into browsers by default. You must generally obtain their code and information from elsewhere on the Internet. For example, the Twitter API lets you display your most recent tweets on your website. It provides a unique set of constructs for querying the Twitter service and retrieving specified information.
JavaScript's relationship with APIs and other JavaScript Tools
So far, we've discussed what client-side JavaScript APIs are and how they connect to the JavaScript programming language. Let's go over this again for clarity and also highlight where other JavaScript tools fall in:
JavaScript is a high-level scripting language incorporated into browsers, allowing you to add functionality to web pages/apps. It should be noted that other programming environments, such as Node, also support JavaScript.
Browser APIs - Browser APIs are built-in components that sit on top of the JavaScript language and allow you to create functionality easily.
Third-party APIs - Third-party APIs are mechanisms embedded into third-party platforms (such as Twitter and Facebook) that allow you to use some of that platform's functionality on your websites (for example, displaying your most recent Tweets on your web page).
JavaScript libraries – Typically, one or more JavaScript files containing custom functions that you can use on your web page to speed up or let the creation of standard functionality. jQuery, Mootools, and React are a few examples.
JavaScript frameworks — JavaScript frameworks (e.g., Angular and Ember) are packages containing HTML, CSS, JavaScript, and other technologies you install and then use to construct a whole web application from scratch. "Inversion of Control" is the primary distinction between a library and a framework. The developer has control when calling a method from a library. The command is inverted with a framework: the framework calls the developer's code.
What are the capabilities of APIs?
In modern browsers, a plethora of APIs are available that allow you to do a wide range of things in your code. The most common types of browser APIs you'll utilize (and which we'll go over in greater detail in this module) are:
APIs for modifying documents that have been loaded into the browser. The most obvious example is the D.O.M. (Document Object Model) API, which lets you edit HTML and CSS by adding, removing, and updating HTML, applying new styles to your website dynamically, and so on. The D.O.M. is in motion whenever you see a popup window displaying on a page or fresh content presented, for example. Learn more about these APIs in Manipulating documents.
APIs that retrieve data from the server on their own to update specific areas of a webpage are relatively frequent. This seemingly minor detail has had a significant impact on site performance and behavior — if you only need to update a stock listing or list of available new stories, doing so instantly rather than reloading the complete page from the server, can make the site or app feel much more responsive and "snappy."
The Fetch API is the most commonly used for this. However, older code may still utilize the XMLHttpRequest API. You may also hear the phrase Ajax, which refers to this procedure. Learn more about such APIs in the section Fetching data from the server.
The most popular APIs for drawing and editing images are Canvas and WebGL. These allow you to dynamically update the pixel data stored in an HTML canvas> element for creating 2D and 3D scenarios. For example, you could use the Canvas API to draw objects like rectangles or circles, load an image onto the canvas, apply a filter like sepia or grayscale, or use WebGL to construct a complicated 3D scene with lighting and textures.
These APIs are frequently coupled with APIs for building animation loops (such as a window.requestAnimationFrame()) and others to create continuously updated scenes such as cartoons and games.
Audio and video APIs such as HTMLMediaElement, the Web Audio API, and WebRTC enable you to do exciting things with multimedia, such as creating custom U.I. controls for playing audio and video, displaying text tracks such as captions and subtitles alongside your videos, grabbing video from your web camera to be manipulated or displayed on someone else's computer in a web conference. It can also add effects to audio tracks (such as gain, distortion, panning, and pausing).
Device APIs let you interface with the device hardware, for as using the Geolocation API to determine the user's location.
Client-side storage APIs allow you to store data on the client side, allowing you to design an app that saves its state between page loads and may even run when the device is offline.
Numerous alternatives exist, such as simple name/value storage using the Web Storage API and more complicated database storage using the IndexedDB API.
Most Common Third-party APIs
Third-party APIs come in a wide range; some of the most popular ones that you will most certainly utilize at some point are:
The Twitter API allows you to show your most recent tweets on your website.
Map APIs, such as Mapquest and the Google Maps API, allow you to do various things with maps on your website.
The Facebook API suite allows you to profit from various components of the Facebook ecosystem, such as offering app login using Facebook login, accepting in-app purchases, rolling out tailored ad campaigns, etc.
The Telegram APIs enable you to incorporate content from Telegram channels on your website while supporting bots.
The YouTube API allows you to embed videos on your website, search YouTube, create playlists, and many other things.
The Pinterest API offers tools for managing and incorporating Pinterest boards and pins into your website.
The Twilio API provides a framework for incorporating audio and video call features into your app and sending SMS/MMS from your app.
The Mastodon API allows you to change features of the Mastodon social network programmatically.
How do APIs Function?
APIs facilitate infrastructure connection via cloud-native app development. Still, they also enable data sharing with consumers and other external users.
When you use a mobile phone application, it will connect to the Internet and will send data to a server
The server then retrieves the data, interprets it, takes the required actions, and returns it to your phone.
The data on your phone is never totally exposed to the server, and the server is never fully exposed to your phone. Instead, each interacts with little data packets, sharing only what is required.
The application then interprets that data and displays the information you requested legibly. This is what an API is - everything happens through an API.
An Actual API Sample
When you look for flights online, you have several options, including different cities and departure and return dates.
To book your flight, you interact with the airline's website to check their database and check if any seats are available or not on those dates, along with the costs.
You may, however, not be utilizing the airline's website. You could be using a channel that has direct access to the information, such as the online travel provider Expedia, which collects data from multiple airline databases.
In this scenario, the travel service interacts with the airline's API. The API is the mechanism through which the online travel provider can obtain information from the airline's database to book seats and luggage options.
The API then returns the airline's response to your request to the online travel service, which displays the most recent, relevant information.
Methodologies for API release policies
Private: The API is only available for internal use. This provides businesses with the most control over their API.
Partner: The API is made available to specific business partners. This can provide additional revenue without sacrificing quality.
Public: The API is open to the general public. This enables third-party developers to create apps that interface with your API, which can be a source of innovation.
What are Public APIs and API integration?
APIs are a long-standing idea in computer programming and have long been a part of developers' toolkits. APIs were traditionally used to connect code components running on the same machine. With the growth of ubiquitous networking, many public APIs (also known as open APIs) have become available.
Public APIs are outward-facing and Internet-accessible, allowing you to build code that interacts with the code of other suppliers online; this process is known as API integration. These code mashups enable customers to combine features from several providers on their platforms. For example, if you use Marketo for marketing automation, you can sync your data with Salesforce CRM capability.
'Open' or 'public' should not be understood as 'free of charge' in this context. You must still be a Marketo and Salesforce customer for this to function. However, the availability of these APIs makes integration more manageable than it would otherwise be.
What are Remote APIs?
Remote APIs are intended to communicate via a communications network. The popular term remote refers to the fact that the resources being changed by the API are located somewhere other than the computer initiating the request.
Because the Internet is the most extensively used communication network, most APIs are built using web standards. Although not all remote APIs are web APIs, it is reasonable to presume they are remote.
Web APIs typically use HTTP for request messages and give a structural specification for response messages. These responses are often in the form of an XML or JSON file. XML and JSON have favored formats because they display data in a way other applications can easily manipulate.
The Modern API/Digital API
Over the years, the term 'API' has frequently been used to refer to any generic connectivity access to a program. However, modern APIs have recently acquired some qualities that make them desirable and valuable:
Modern APIs adhere to developer-friendly, easily accessible, and widely understood standards (usually HTTP and REST).
They are treated as though they were goods rather than codes. They are intended for a specific audience (for example, smartphone developers).
Modern APIs are defined and versioned so that consumers can have reasonable expectations about their maintenance and lifecycle.
They have a much greater discipline for security and governance since they are more uniform.
Modern APIs have their software development lifecycle (SDLC) that includes designing, testing, creating, managing, and versioning.
Application of API
Assume your small business's website offers a form for clients to sign up for appointments. You want to give clients the option of immediately creating a Google calendar event with the appointment details.
The aim is for your website's server to communicate directly with Google's server, requesting the creation of an event with the specified details. After receiving Google's response, the server evaluates it.
It returns pertinent information to the browser, such as a confirmation message to the user. In addition, the browser can frequently make an API request directly to Google's server, bypassing your server.
What distinguishes this Google Calendar API from any other remote server API? The distinction in technical terms is the format of the request and answer. Your browser expects a response in HTML that includes presentational code to render the entire webpage.
In contrast, Google Calendar's API call would deliver the data (presumably in JSON format).
If your website's server is making the API call, your website's server is the client (just like when you use your browser to navigate to a website). APIs, from the perspective of your users, allow them to finish the action without leaving your website.
Conclusion
You should now understand and be clear with the concept of APIs, it's working, and how to get them in your JavaScript code. You're undoubtedly excited to start doing exciting things with specific APIs, so let's get started!
Choosing whether to build your solution with APIs or messaging is not an either/or choice; sometimes, both are required. APIs and messaging both offer numerous advantages for creating sophisticated, engaging apps.
Read More: A Beginner's Guide on Working with APIs
Comments