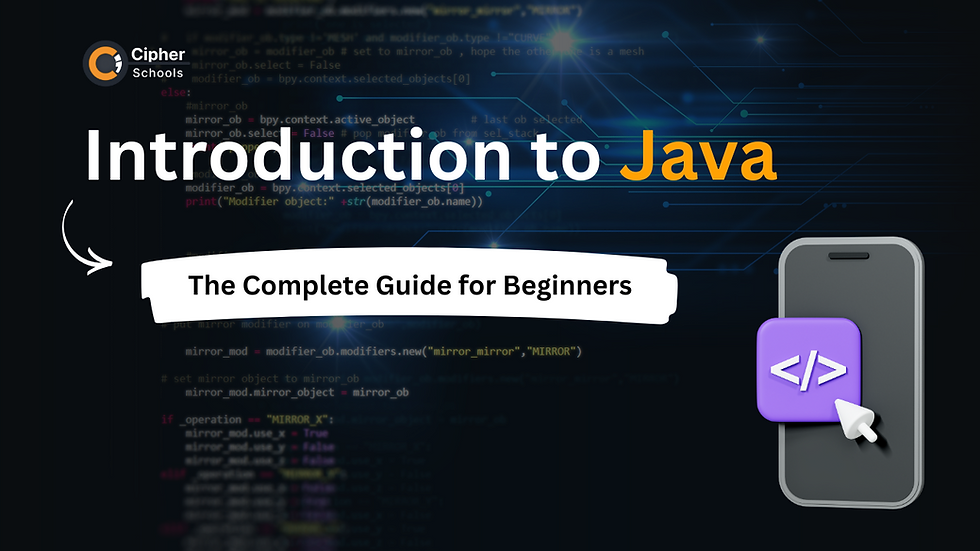
Introduction
Java, one of the most popular programming languages in the world, continues to dominate the software development landscape with its versatility, reliability, and vast ecosystem. Java offers a robust foundation, whether you're developing web applications, Android apps, or large-scale enterprise software. This introduction to Java serves as a comprehensive guide for beginners, providing everything you need to know to start your programming journey.
In this guide, we'll explore Java's history, its standout features, and why it's a must-learn language for aspiring developers. You'll gain insights into its syntax, key concepts like object-oriented programming (OOP), and practical applications. By the end of this guide, you'll have a solid understanding of Java's core features and how to apply them in real-world scenarios, making it an essential resource for anyone venturing into software development.
History and Evolution of Java
Java has a rich history that underscores its relevance and enduring popularity in the programming world. Created in 1995 by Sun Microsystems, Java was initially designed to enable interactive television, but its creators quickly realised its potential as a versatile programming language. With its “Write Once, Run Anywhere” (WORA) capability, Java became a game-changer in software development, enabling developers to write code that could run on any platform with a Java Virtual Machine (JVM).
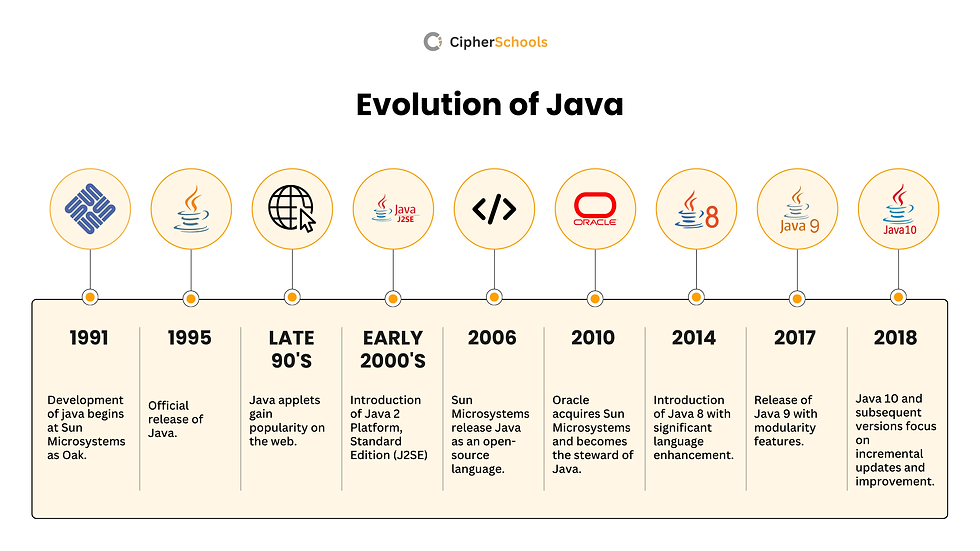
Over the decades, Java has evolved significantly. From its humble beginnings to becoming the backbone of enterprise software, Java has consistently adapted to the needs of developers and businesses. Regular updates and the introduction of powerful features, such as lambdas and modules, have ensured its relevance in a competitive programming landscape.
In the modern world, Java powers a vast range of applications, including web development, Android mobile apps, enterprise solutions, and even Internet of Things (IoT) devices. Its adaptability makes it a critical skill for developers across industries.
The journey of Java is marked by its major versions:
Java SE (Standard Edition): The core platform for general-purpose programming.
Java EE (Enterprise Edition): Tailored for enterprise-level applications, now known as Jakarta EE.
Java ME (Micro Edition): Designed for resource-constrained devices.
Java's history is a testament to its resilience and versatility, making it a foundational tool for developers aiming to create reliable and scalable solutions.
Why Learn Java?
Java is a language that every aspiring programmer should consider mastering, thanks to its unique features and vast applications in the tech industry. Here's why learning Java is a wise choice for beginners and experienced developers alike.
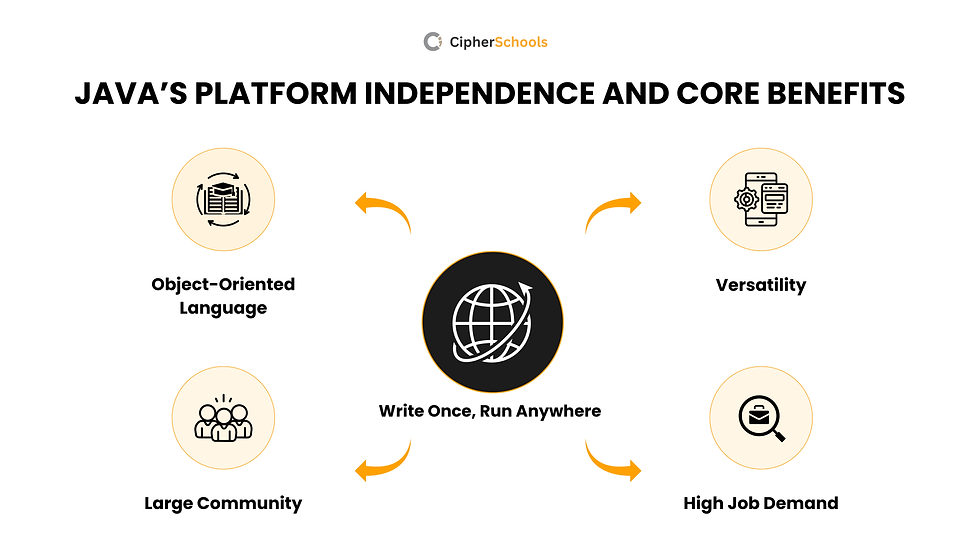
1. Platform Independence
Java’s hallmark feature, “Write Once, Run Anywhere” (WORA), allows developers to write code that can run on any platform with a Java Virtual Machine (JVM). This cross-platform compatibility makes Java ideal for developing software that operates seamlessly across multiple devices and operating systems.
2. Object-Oriented Language
Java is an object-oriented programming (OOP) language, making it easier to write modular and reusable code. Core OOP principles like encapsulation, inheritance, and polymorphism are embedded in Java, helping developers build scalable and maintainable applications.
3. Large Community and Support
Java boasts one of the largest developer communities in the world. This means a wealth of resources, libraries, frameworks, and tutorials are available to support learners. Whether you’re troubleshooting issues or exploring advanced features, the Java community is always there to help.
4. High Demand in the Job Market
Java continues to be a sought-after skill in the tech industry. With applications spanning web development, mobile apps, enterprise software, and cloud computing, proficiency in Java opens doors to lucrative job opportunities.
5. Versatility
Java is incredibly versatile. It’s used to build everything from web applications and Android apps to server-side systems and IoT devices. This makes it a go-to language for projects of all sizes and complexities.
Learning Java equips developers with a powerful toolset to tackle diverse challenges, making it a cornerstone of modern software development.
Setting Up Java Development Environment
Starting with Java begins with setting up the right tools for coding, compiling, and running your programs. Here’s a step-by-step guide to setting up your Java development environment.

1. Installing Java Development Kit (JDK)
The Java Development Kit (JDK) is essential for writing and running Java programs. Follow these steps to install it:
Windows:
Download the latest JDK from the Oracle website.
Run the installer and follow the prompts.
Set the JAVA_HOME environment variable in your system properties.
macOS:
Use Homebrew: Run brew install openjdk.
Update your shell profile to include the JAVA_HOME path.
Linux:
Use the package manager for your distribution (e.g., sudo apt install openjdk-17-jdk for Ubuntu).
Verify the installation by running java -version in the terminal.
2. Setting Up an Integrated Development Environment (IDE)
An IDE simplifies coding by providing tools like syntax highlighting, debugging, and project management. Popular choices include:
IntelliJ IDEA: Known for its smart code completion and powerful tools.
Eclipse: A long-standing favorite with extensive plugin support.
NetBeans: Great for beginners, with a user-friendly interface.Install your preferred IDE and configure it to recognize the JDK you installed.
3. Writing Your First Java Program
Start with a simple “Hello World” program to familiarize yourself with the setup:
Open your IDE and create a new Java project.
Create a new class file named HelloWorld.
Write the following code:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
Running and Debugging Java Code
Compile your program by clicking the "Run" button in your IDE or using the javac
command in the terminal:
javac HelloWorld.java
Run the program with:
java HelloWorld
Use debugging tools in your IDE to inspect variables, step through code, and identify
issues.
By following these steps, you’ll have a fully functional Java development environment ready to explore the language further.
Understanding Java Syntax and Structure
Grasping Java’s syntax and structure is essential for writing effective programs. Here’s a concise overview of its core components:
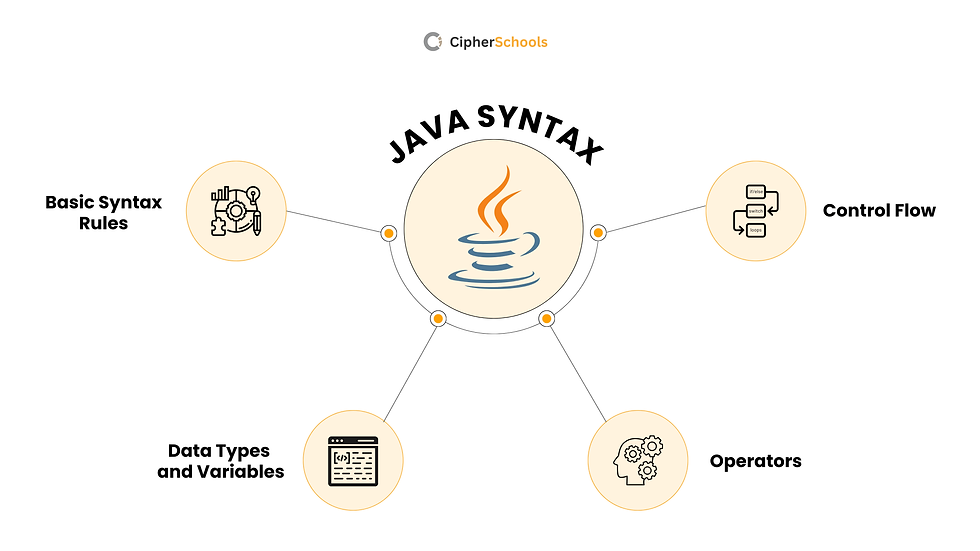
1. Basic Syntax Rules
Class Definition: Every Java program starts with a class (e.g., public class HelloWorld).
Method Declaration: The main method (public static void main(String[] args)) is the program’s entry point.
Semicolons: Statements end with a semicolon (;).
2. Java Keywords
Important keywords include:
class (defines a class), public (sets visibility), static (belongs to the class), and void (no return value).
3. Data Types and Variables
Java supports:
Primitive Types: int, float, boolean, char.
Reference Types: Objects and arrays.Example: int age = 25; boolean isActive = true;.
4. Operators and Control Flow
Operators: Arithmetic (+, -), Relational (>, <), Logical (&&, ||).
Conditional Statements:
if: if (age > 18) { System.out.println("Adult"); }
switch: For multiple cases.
Loops:
for: Iterates over a range.
while/do-while: Repeats until a condition changes.
This basic understanding of Java’s syntax will pave the way for exploring more advanced features and building functional programs.
Object-Oriented Programming (OOP) in Java
Java is based on Object-Oriented Programming (OOP) principles, which help create modular and reusable code.

Here are the core OOP concepts in Java:
1. Class and Objects
A class is a blueprint for objects, and an object is an instance of a class.
2. Encapsulation
Encapsulation hides data inside classes, using private variables and public methods to access them.
3. Inheritance
Inheritance allows one class to inherit properties and methods from another, promoting code reuse.
4. Polymorphism
Polymorphism lets objects behave differently based on their class, through method overloading and overriding.
5. Abstraction
Abstraction hides implementation details using abstract classes or interfaces, focusing on functionality.
Here’s an example that demonstrates all of these concepts:
abstract class Animal { // Abstraction
abstract void sound(); // Abstract method
}
class Dog extends Animal { // Inheritance
private String breed; // Encapsulation
Dog(String breed) {
this.breed = breed;
}
void sound() { // Polymorphism (Overriding)
System.out.println("Bark");
}
public String getBreed() {
return breed; // Encapsulation
}
}
public class Main {
public static void main(String[] args) {
Dog myDog = new Dog("Bulldog");
myDog.sound();
System.out.println("Breed: " + myDog.getBreed());
}
}
This code illustrates how OOP concepts work together: encapsulation for data protection, inheritance for reusing code, polymorphism for method overriding, and abstraction to hide the specific sound-making method in the abstract class.
Important Java Concepts
Understanding key Java concepts is essential for building efficient and scalable applications. Here are some important concepts you should know:
1. Arrays
Arrays are used to store multiple values in a single variable. Once created, arrays are fixed in size in Java.
Example:
int[] numbers = {1, 2, 3, 4};
System.out.println(numbers[0]); // Output: 1
2. String Handling
Java provides a String class for handling text. Common methods include substring(), concat(), and equals().
Example:
String greeting = "Hello";
String name = "World";
String message = greeting.concat(" " + name);
System.out.println(message); // Output: Hello World
3. Collections Framework
Java’s Collections Framework includes classes and interfaces for storing and manipulating data. Key collections are:
List: An ordered collection (e.g., ArrayList).
Set: A collection that does not allow duplicates (e.g., HashSet).
Map: A collection of key-value pairs (e.g., HashMap).
Example with a HashMap:
Map<String, Integer> map = new HashMap<>();
map.put("Alice", 30);
map.put("Bob", 25);
System.out.println(map.get("Alice")); // Output: 30
4. Exception Handling
Java handles errors using exceptions. The try-catch block is used to catch exceptions and handle them gracefully.
Example:
try {
int result = 10 / 0;
} catch (ArithmeticException e) {
System.out.println("Error: " + e.getMessage());
// Output: Error: / by zero
}
5. Custom Exceptions
You can create your own exceptions to handle specific errors. Example:
class InvalidAgeException extends Exception {
public InvalidAgeException(String message) {
super(message);
}
}
public class Main {
public static void main(String[] args) {
try {
throw new InvalidAgeException("Age cannot be negative");
} catch (InvalidAgeException e) {
System.out.println(e.getMessage()); // Output: Age cannot be negative
}
}
}
These concepts form the foundation for working effectively with Java, enabling you to handle data, errors, and complex structures with ease.
Java Memory Management
Java's memory management system plays a crucial role in ensuring efficient program execution. Here's an overview of how memory is handled in Java:
1. Heap and Stack Memory
Java manages memory through two main areas:
Heap Memory: Used for dynamic memory allocation, where objects are stored. The heap is managed by the garbage collector.
Stack Memory: Stores method calls and local variables. Each thread in a Java program has its own stack.
2. Garbage Collection
Java’s garbage collector automatically frees up memory by removing objects that are no longer in use. This helps avoid memory leaks. The garbage collector runs in the background and manages memory without requiring explicit calls to free it.
3. Tips for Efficient Memory Use
To optimize memory usage in Java:
Use primitive types where possible (they use less memory than objects).
Avoid creating unnecessary objects; reuse objects when possible.
Use StringBuilder for concatenating strings to reduce memory overhead.
By understanding how Java handles memory and utilizing best practices, you can improve the performance of your applications.
Java Input and Output (I/O)
Handling input and output (I/O) is an essential part of Java programming, allowing your program to interact with users and external systems like files.
Here’s how you can work with Java I/O:
1. Streams in Java
Java uses streams for reading and writing data. A stream is a sequence of data that can be read from or written to a source, such as the console or a file.
Input Stream: Reads data (e.g., System.in for user input).
Output Stream: Writes data (e.g., System.out for displaying output).
2. Reading from Console
To read user input, you can use the Scanner or BufferedReader classes:
import java.util.Scanner;
Scanner scanner = new Scanner(System.in);
System.out.print("Enter your name: ");
String name = scanner.nextLine();
System.out.println("Hello, " + name);
3. Writing to Files
Java provides classes like FileWriter, BufferedWriter, and PrintWriter for writing to files.
Example with FileWriter:
import java.io.FileWriter;
import java.io.IOException;
try (FileWriter writer = new FileWriter("output.txt")) {
writer.write("Hello, file!");
} catch (IOException e) {
System.out.println("Error writing to file: " + e.getMessage());
}
By understanding Java's I/O mechanisms, you can effectively manage user input, output to the console, and work with external files.
Java Multithreading and Concurrency
Multithreading allows Java programs to execute multiple tasks simultaneously, improving efficiency and performance. Here’s a breakdown of how multithreading works in Java:
1. What is Multithreading?
Multithreading is the ability of a program to perform multiple operations at once, with each thread executing independently but sharing the same memory space.
2. Creating Threads
Threads can be created in Java by either extending the Thread class or implementing the Runnable interface.
Using the Thread class:
class MyThread extends Thread {
public void run() {
System.out.println("Thread is running");
}
}
public class Main {
public static void main(String[] args) {
MyThread thread = new MyThread();
thread.start(); // Starts the thread
}
}
Using the Runnable interface:
class MyRunnable implements Runnable {
public void run() {
System.out.println("Runnable thread is running");
}
}
public class Main {
public static void main(String[] args) {
Thread thread = new Thread(new MyRunnable());
thread.start();
}
}
3. Thread Life Cycle
A thread in Java can be in one of the following states:
New: The thread has been created but has not yet started.
Runnable: The thread is ready to run and waiting for CPU time.
Blocked: The thread is waiting to acquire a resource.
Waiting: The thread is waiting for another thread to perform a particular action.
Terminated: The thread has completed its execution.
4. Synchronization and Locks
When multiple threads access shared resources, synchronization ensures that only one thread can access the resource at a time to avoid conflicts.
class Counter {
private int count = 0;
public synchronized void increment() { // Synchronize method
count++;
}
public int getCount() {
return count;
}
}
public class Main {
public static void main(String[] args) {
Counter counter = new Counter();
Thread thread1 = new Thread(() -> counter.increment());
Thread thread2 = new Thread(() -> counter.increment());
thread1.start();
thread2.start();
}
}
Multithreading and concurrency in Java are vital for building high-performance applications that can handle multiple tasks at once.
Java GUI Development
Java provides powerful libraries for creating graphical user interfaces (GUIs). The two most common libraries are Swing and JavaFX, allowing you to build interactive applications with buttons, windows, and other GUI components.
1. Introduction to JavaFX and Swing
Swing: Older library, still widely used for building GUIs in Java.
JavaFX: A modern, feature-rich library for creating GUI applications.
2. Creating Basic Windows
In JavaFX, you can create a simple window using Stage and Scene.
Here’s an example of a basic JavaFX application that displays a window with a button:
import javafx.application.Application;
import javafx.stage.Stage;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.StackPane;
public class HelloWorld extends Application {
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage primaryStage) {
Button btn = new Button("Click Me");
btn.setOnAction(e -> System.out.println("Hello, World!"));
StackPane root = new StackPane();
root.getChildren().add(btn);
Scene scene = new Scene(root, 300, 250);
primaryStage.setTitle("JavaFX Hello World");
primaryStage.setScene(scene);
primaryStage.show();
}
}
This code creates a window with a button. When clicked, the button prints "Hello, World!" to the console.
3. Event Handling
Handling user input (like mouse clicks or keyboard presses) is essential in GUI applications.
In JavaFX, you can register event handlers for components like buttons:
Button btn = new Button("Click Me");
btn.setOnAction(e -> System.out.println("Button clicked"));
Java GUI development in JavaFX is an excellent way to build modern desktop applications with rich interfaces, making it easier to engage with users interactively.
Introduction to Java Frameworks
Java frameworks simplify application development by providing pre-built solutions for common tasks like database handling, web development, and dependency management. Some of the most popular Java frameworks include Spring, Hibernate, and Apache Maven/Gradle.
1. Spring Framework
The Spring Framework is a powerful, lightweight framework used for building enterprise-level applications. It provides comprehensive infrastructure support, including:
Dependency Injection (DI): Simplifies object creation and reduces tight coupling between components.
Aspect-Oriented Programming (AOP): Helps separate cross-cutting concerns (e.g., logging, security).
Spring Boot: A tool to quickly set up Spring-based applications with minimal configuration.
Example of a simple Spring Bean:
@Component
public class HelloService {
public void sayHello() {
System.out.println("Hello, Spring!");
}
}
2. Hibernate
Hibernate is an Object-Relational Mapping (ORM) framework that simplifies database interaction by mapping Java objects to database tables. It eliminates the need for complex SQL queries and improves code maintainability.
Example of a simple Hibernate entity:
@Entity
public class Employee {
@Id
private int id;
private String name;
// getters and setters
}
3. Apache Maven and Gradle
Apache Maven and Gradle are popular build automation tools used for managing project dependencies and building Java applications.
Maven uses XML configuration files to manage dependencies and project builds.
Gradle is a more flexible build tool that uses Groovy-based scripts.
Example Maven dependency in pom.xml:
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>5.3.10</version>
</dependency>
Using Java frameworks accelerates development, ensures best practices, and provides built-in solutions for common challenges, allowing developers to focus on business logic rather than infrastructure.
Best Practices in Java Programming
Writing clean, efficient, and maintainable code is essential for long-term project success. Following best practices in Java programming ensures that your code is reliable, scalable, and easier to understand.
1. Writing Clean Code
Use meaningful variable and method names: Names should describe the purpose of the variable or method.
Keep methods short and focused: Each method should do one thing and do it well.
Follow coding conventions: Adhere to Java's standard naming conventions (e.g., camelCase for variables, methods, and PascalCase for classes).
Avoid code duplication: Reuse code wherever possible, and use functions to avoid repeating logic.
2. Testing and Debugging
Unit testing: Use JUnit to write automated tests for your methods, ensuring code correctness.
Debugging: Use a debugger to step through your code, identify issues, and understand the flow of execution.
Example of a simple unit test using JUnit:
import org.junit.Test;
import static org.junit.Assert.assertEquals;
public class CalculatorTest {
@Test
public void testAdd() {
Calculator calc = new Calculator();
assertEquals(5, calc.add(2, 3));
}
}
3. Version Control
Using Git for version control is essential for tracking changes, collaborating with others, and maintaining code history.
Commit frequently: Make small, logical commits to track progress and changes.
Branching: Use branches for new features or bug fixes, and merge them into the main branch when complete.
By following these best practices, you ensure that your Java code is clean, testable, and maintainable, ultimately leading to more efficient and collaborative development.
Conclusion
In this guide, we've covered the essentials of Java programming, from its history and evolution to key concepts and best practices. Whether you're a beginner or an experienced developer, understanding Java's core features, syntax, and capabilities is crucial for building powerful and scalable applications.
To recap:
Java's versatility makes it suitable for everything from mobile apps to enterprise software and IoT devices.
Object-oriented programming (OOP) principles like encapsulation, inheritance, and polymorphism form the foundation of Java.
Java's rich libraries, frameworks, and tools (such as Spring, Hibernate, and Maven) simplify development and help you build robust applications.
Following best practices ensures that your code is clean, maintainable, and scalable.
Call to Action: Now that you have a solid understanding of Java, it's time to start coding! Dive into building your own Java projects, explore more advanced topics like networking, RESTful APIs, and microservices, and continue to hone your skills as you grow as a Java developer.
Further Reading:
Explore advanced Java topics like concurrency, design patterns, and Java Streams.
Look into popular Java frameworks like Spring Boot and Hibernate for enterprise development.
Learn about building web services and APIs using Java.
By mastering Java, you'll be well-equipped for a rewarding career in software development!
FAQ
What is the basic introduction of Java?
Java is a high-level, object-oriented programming language designed to have as few implementation dependencies as possible. Developed by Sun Microsystems (now owned by Oracle), it is known for its portability across platforms, due to the principle "write once, run anywhere."
How is Java for beginners?
What is Java and its types?
Can I tech myself Java?
Comments