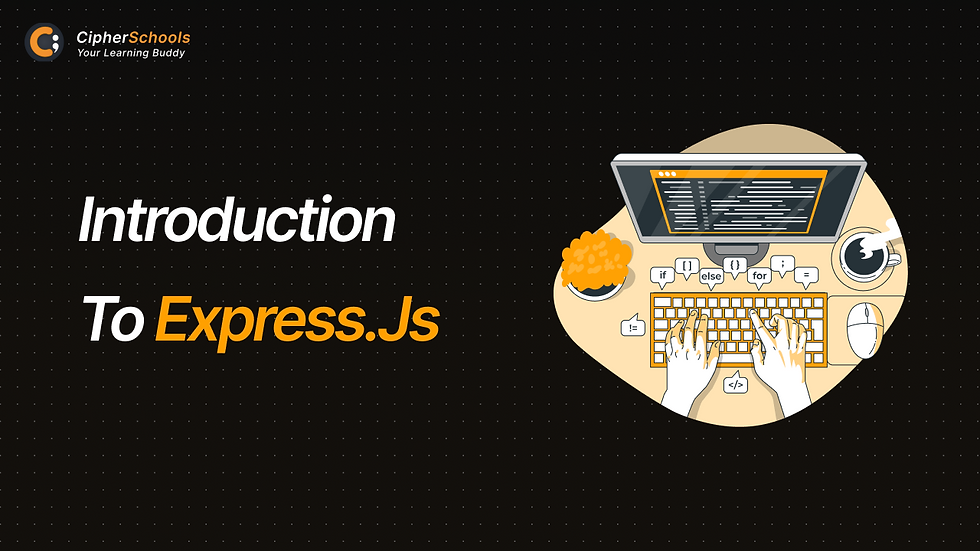
Why Use Express?
Express.js is a popular and minimalist web application framework for Node.js. It simplifies the process of building robust and scalable web applications and APIs. Here is why you should consider using Express.js:
1. Simplicity: Express provides a straightforward and unopinionated structure that makes it easy to get started with web development in Node.js. It allows you to focus on writing code rather than dealing with boilerplate.
2. Middleware: Express comes with a powerful middleware system that simplifies tasks like parsing requests, handling cookies, and implementing authentication. You can use built-in middleware or create custom middleware to tailor your application's behavior.
3. Routing: Express offers a clean and efficient routing system that enables you to define API endpoints and handle HTTP requests with ease. It supports various HTTP methods like GET, POST, PUT, and DELETE.
4. Ecosystem: Express has a vast ecosystem of third-party middleware and extensions, allowing you to add functionality to your application quickly. Whether you need authentication, database integration, or logging, there is likely a middleware package available.
5. Performance: Express is known for its high performance and efficiency, making it a popular choice for building web servers and APIs that need to handle a high volume of requests.
Setting Up Express in a Node.js Application
Installing Express
To add Express to your Node.js application, start by initializing a new Node.js project if you have not already. Then, follow these steps:
1. Navigate to your project directory
cd your-project-directory
2. Install Express using npm
npm install express
Initializing Express Middleware
To set up Express middleware, import Express and create an instance of it in your application. Here is a basic example:
const express = require('express');
const app = express();
You can now use `app` to define routes, configure middleware, and handle HTTP requests.
Creating Routes with Express
Defining API Routes
Express makes it easy to define routes for your application. You can specify routes and associate functions with them to handle requests. Here is a simple example:
app.get('/', (req, res) => {
res.send('Hello, Express!');
});
app.get('/about', (req, res) => {
res.send('About Us');
});
In this code, two routes are defined—one for the root path `'/'` and another for `/about`. When a user accesses these paths, Express responds with the specified messages.
Handling Route Parameters and Queries
Express allows you to capture route parameters and query parameters from incoming requests. For example:
app.get('/user/:id', (req, res) => {
const userId = req.params.id;
res.send(`User ID: ${userId}`);
});
app.get('/search', (req, res) => {
const query = req.query.q;
res.send(`Search query: ${query}`);
});
In the first route, `:id` is a route parameter that can be accessed using `req.params.id`.
In the second route, the query parameter `q` is extracted from the URL using `req.query.q`.
Express provides a flexible and intuitive way to define routes, handle parameters, and build APIs quickly.
Interacting with Clients in Express.js
Handling HTTP Requests
Parsing Request Data (Body, Query Parameters)
Handling and parsing data from HTTP requests is a fundamental aspect of web development. In Express.js, you can easily parse request data, whether it's from the request body or query parameters:
Request Body:
To parse data from the request body, you can use middleware like express.json() for JSON data or express.urlencoded() for form data. Here's how you can set it up:
const express = require('express');
const app = express();
// Middleware for parsing JSON requests
app.use(express.json());
// Middleware for parsing form data
app.use(express.urlencoded({ extended: true }));
Now, you can access request data from req.body.
Query Parameters:
To parse query parameters from the URL, you can use req.query. For example:
app.get('/search', (req, res) => {
const query = req.query.q;
res.send(`Search query: ${query}`);
});
Sending JSON Responses
To send JSON responses in Express.js, you can use the res.json() method. Here's an example:
app.get('/api/data', (req, res) => {
const data = { message: 'Hello, JSON!' };
res.json(data);
});
This sends a JSON response to the client with the specified data.
Error Handling in Express
Handling Errors Gracefully
Error handling is crucial in any web application. Express provides mechanisms for handling errors gracefully, such as using try...catch blocks or middleware functions.
For synchronous code, you can use a middleware function with four parameters to handle errors:
app.use((err, req, res, next) => {
console.error(err.stack);
res.status(500).send('Something went wrong!');
});
Implementing Custom Error Handling Middleware
You can also create custom error-handling middleware for specific error scenarios. For example, if you have an authentication error, you can handle it like this:
function authenticationError(err, req, res, next) {
if (err.name === 'UnauthorizedError') {
res.status(401).json({ error: 'Unauthorized' });
} else {
next(err);
}
}
app.use(authenticationError);
CORS (Cross-Origin Resource Sharing)
Configuring CORS in Express for Cross-Origin Requests
Cross-Origin Resource Sharing (CORS) is essential when dealing with web applications that make requests to different domains. To enable CORS in Express.js, you can use the Cors middleware package.
Installation:
Start by installing the Cors package:
npm install cors
Configuration:
Then, configure it in your Express application:
const express = require('express');
const cors = require('cors');
const app = express();
// Enable CORS for all routes
app.use(cors());
// Optionally, configure specific CORS options
const corsOptions = {
origin: 'https://example.com',
methods: 'GET,POST',
};
app.use(cors(corsOptions));
The Cors middleware allows you to control which origins are allowed to access your server and which HTTP methods are permitted. This helps enhance security while enabling cross-origin requests.
In conclusion, interacting with clients in Express.js involves handling HTTP requests, parsing request data, sending JSON responses, handling errors gracefully, and configuring CORS for cross-origin requests. These features are essential for building secure and efficient web applications and APIs using Express.js.
Comments