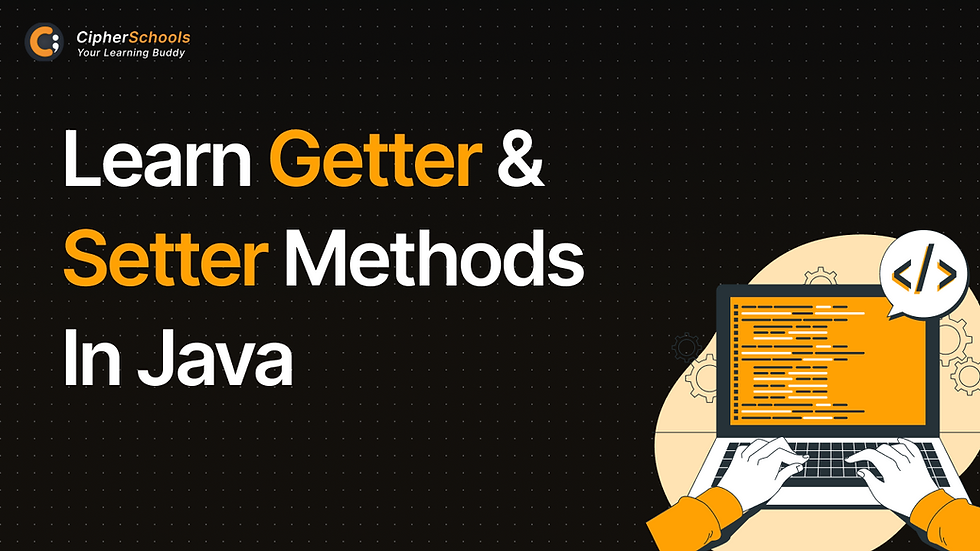
Getter and setter methods play a crucial role in Java programming, providing a mechanism for accessing and modifying class attributes while encapsulating data. In this comprehensive guide, we'll explore the concepts of getter and setter methods in Java, their usage, implementation, and best practices with practical examples.
What are Getter and Setter in Java with Examples?
Getter and setter methods, also known as accessors and mutators, are Java methods used to retrieve (get) and modify (set) the values of private variables (data members) of a class, respectively. They facilitate data encapsulation, ensuring controlled access to class attributes while maintaining class integrity and abstraction.
Uses of Getter and Setter Method in Java:
Getter and setter methods are used to implement the principle of encapsulation in Java, providing controlled access to class attributes and enabling data abstraction. They serve the following purposes:
Encapsulating class attributes
Enforcing data validation and integrity
Facilitating code maintainability and flexibility
Supporting information hiding and abstraction
Naming Convention for Getter and Setter in Java:
The naming convention for getter and setter methods in Java follows the JavaBeans naming convention, where getter methods start with "get" or "is" (for boolean attributes) and setter methods start with "set," followed by the attribute name in camel case.
Syntax for Getter Method:
The syntax for a getter method in Java is as follows:
public returnType getAttributeName() {
// return the value of the attribute
}
Syntax for Setter Method:
The syntax for a setter method in Java is as follows:
public void setAttributeName(attributeType attributeName) {
// set the value of the attribute
}
Advantages and Drawbacks of Using Getter and Setter in Java:
Advantages:
Encapsulation: Protects class attributes and promotes data integrity.
Flexibility: Allows controlled access to class attributes.
Maintenance: Facilitates code maintenance and debugging.
Abstraction: Hides internal implementation details from external users.
Drawbacks:
Boilerplate code: Requires writing additional code for each attribute.
Reduced performance: May incur slight performance overhead due to method calls.
Violation of encapsulation: Overuse of getter and setter methods may expose internal details of the class.
Bad Practices in Getter and Setter Methods:
Excessive mutability: Avoid excessive modification of class attributes through setter methods to maintain object consistency.
Direct attribute access: Minimize direct access to class attributes from external classes to ensure encapsulation and data integrity.
Conclusion:
Getter and setter methods are essential components of object-oriented programming in Java, providing a means to encapsulate data, enforce data validation, and maintain class integrity. By following naming conventions and best practices, developers can leverage getter and setter methods effectively to enhance code readability, flexibility, and maintainability.
FAQs:
What is getter and setter in Java?
Getter and setter methods in Java are used to retrieve and modify the values of private variables (data members) of a class, respectively, promoting data encapsulation and abstraction.
What are default getters and setters in Java?
Default getters and setters in Java are automatically generated by the compiler for each private variable of a class if not explicitly defined by the programmer. They follow the standard naming convention and provide access to class attributes.
What is the final getter and setter in Java?
Final getter and setter methods in Java are declared with the final keyword, indicating that subclasses cannot override or modify them. They provide a mechanism for controlled access to immutable class attributes.
How to use Lombok for getter and setter?
Lombok is a Java library that provides annotations to generate boilerplate code, including getter and setter methods, automatically during compilation. By annotating class attributes with @Getter and @Setter, developers can eliminate the need to write getter and setter methods manually.
What are default getters and setters in Java?
Default getters and setters in Java are automatically generated by the compiler for each private variable of a class if not explicitly defined by the programmer. They follow the standard naming convention and provide access to class attributes.
Why do we use getter and setter in Java?
Getter and setter methods in Java are used to encapsulate class attributes, promoting data abstraction, encapsulation, and information hiding. They enable controlled access to class attributes, enhancing code maintainability, flexibility, and security.
Comments