Function Overloading in Python
- Pushp Raj
- Feb 12, 2024
- 3 min read
Updated: Apr 11, 2024
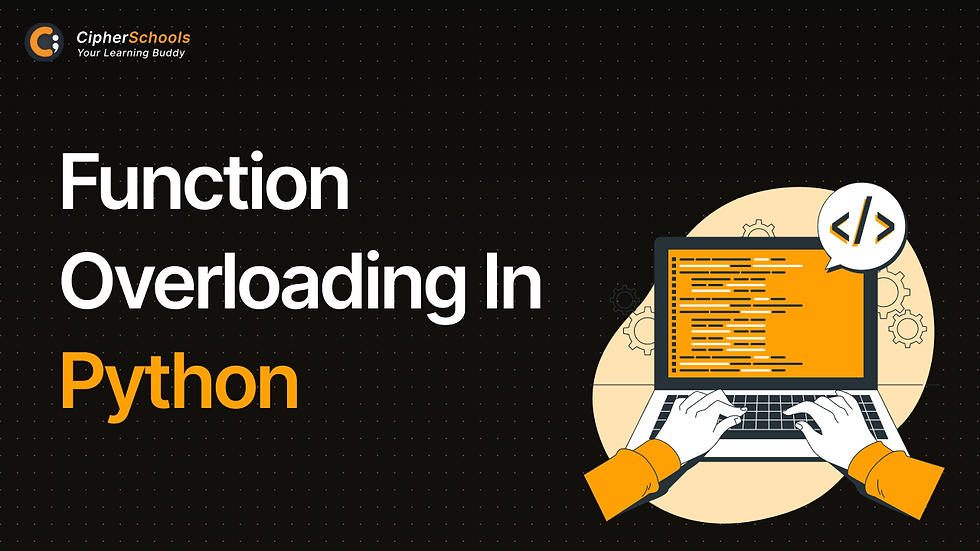
Function overloading is a powerful concept in programming that allows developers to define multiple functions with the same name but with different parameters or types. In Python, although function overloading is not directly supported as in languages like C++ or Java, developers can achieve similar behavior using various techniques. In this comprehensive guide, we will explore the intricacies of function overloading in Python, its implementation, examples, alternatives, and practical applications.
Introduction
Python is known for its simplicity and flexibility, allowing developers to write concise and expressive code. Function overloading enhances this flexibility by enabling the creation of functions with the same name but different parameters or types. This promotes code reuse, improves readability, and enhances the overall design of the program.
What is Function Overloading in Python?
Function overloading refers to the ability to define multiple functions with the same name but different parameter signatures. In languages like C++ or Java, function overloading is supported natively. However, in Python, function overloading is achieved through various techniques such as default arguments, variable-length arguments, and function annotations.
How Does Function Overloading Work in Python?
In Python, function overloading is implemented using techniques such as:
Default Arguments:
python
def add(a, b=0):
return a + b
print(add(2)) # Output: 2
print(add(2, 3)) # Output: 5
Variable-Length Arguments:
python
def add(*args):
return sum(args)
print(add(2)) # Output: 2
print(add(2, 3)) # Output: 5
print(add(2, 3, 4)) # Output: 9
Function Annotations:
python
def add(a: int, b: int) -> int:
return a + b
print(add(2, 3)) # Output: 5
Overloading Built-in Functions in Python with Examples
Python allows overloading of built-in functions by defining methods with special names like add, sub, etc. This is commonly known as operator overloading.
python
class ComplexNumber:
def init(self, real, imaginary):
self.real = real
self.imaginary = imaginary
def add(self, other):
return ComplexNumber(self.real + other.real, self.imaginary + other.imaginary)
def str(self):
return f"{self.real} + {self.imaginary}i"
num1 = ComplexNumber(2, 3)
num2 = ComplexNumber(4, 5)
result = num1 + num2
print(result) # Output: 6 + 8i
Different Methods of Function Overloading in Python
Besides the techniques mentioned above, function overloading can also be achieved using libraries like functools.singledispatch or by explicitly checking the types of arguments within the function.
python
from functools import singledispatch
@singledispatch
def greet(name):
print(f"Hello, {name}!")
@greet.register(int)
def _(age):
print(f"You are {age} years old!")
greet("Alice") # Output: Hello, Alice!
greet(25) # Output: You are 25 years old!
Alternatives to Function Overloading
While function overloading can improve code readability and flexibility, it's essential to consider alternatives such as:
Named Arguments: Providing descriptive names to function parameters can eliminate the need for overloading in many cases.
Function Composition: Breaking down complex functions into smaller, reusable components can lead to cleaner code without the need for overloading.
Syntax of Function Overloading in Python
In Python, there is no specific syntax for function overloading. Instead, developers utilize techniques like default arguments, variable-length arguments, function annotations, or method overloading using decorators.
Conclusion
Function overloading in Python enhances code flexibility and readability by allowing the definition of multiple functions with the same name but different parameter signatures. While Python does not natively support function overloading, developers can achieve similar behavior using techniques like default arguments, variable-length arguments, function annotations, or method overloading. By understanding these concepts and techniques, developers can write more expressive and maintainable code.
FAQs
What is function overloading with an example?
Function overloading refers to defining multiple functions with the same name but different parameter signatures. For example, you can define an add function that accepts different numbers of arguments or types.
What are the drawbacks of using "overloading" techniques?
Overloading techniques can sometimes lead to code complexity and confusion if not used judiciously. It's essential to maintain clarity and readability when employing these techniques.
Where can I find practical examples and tutorials on using these techniques?
You can find numerous resources online, including tutorials, documentation, and community forums, that provide practical examples and guidance on implementing function overloading and related techniques in Python.
Comments