Understanding Encapsulation in Python
- Pushp Raj
- May 5, 2024
- 2 min read
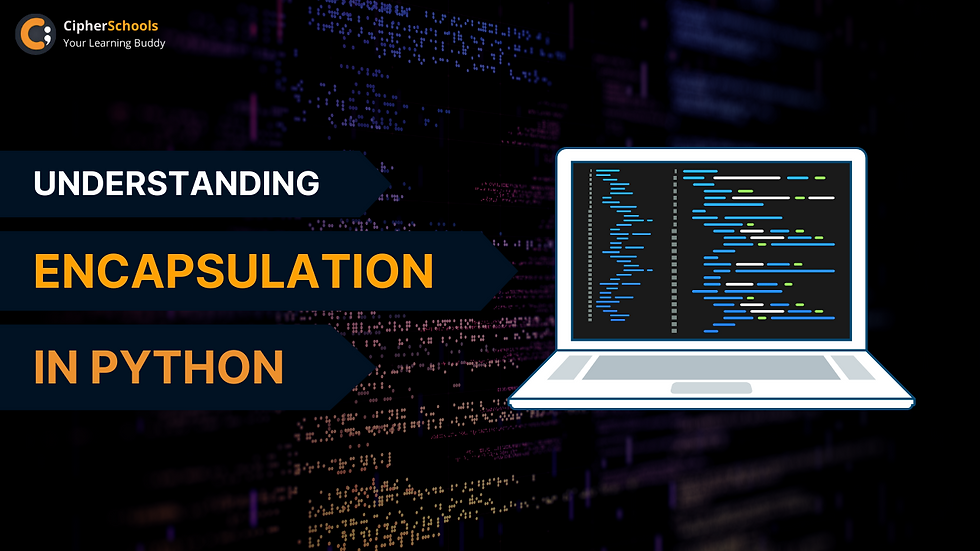
Introduction to Encapsulation in Python
Encapsulation is one of the fundamental concepts in object-oriented programming (OOP) that allows bundling data and methods that operate on the data into a single unit, known as a class. In Python, encapsulation is achieved by using classes and access modifiers to control the visibility of class members.
What is Encapsulation in Python with Example?
Encapsulation in Python involves wrapping the data and methods within a class and restricting access to them from outside the class. This ensures that the internal state of the object is protected and can only be accessed through the defined interfaces.
class Car:
def __init__(self, model, year):
self.__model = model # Private attribute
self.__year = year # Private attribute
def get_model(self):
return self.__model
def get_year(self):
return self.__year
car = Car("Toyota", 2022)
print(car.get_model()) # Output: Toyota
Why do we need Encapsulation in Python?
Well-Defined, Readable Code: Encapsulation helps in organizing code into logical units, making it easier to understand and maintain.
Prevents Accidental Modification or Deletion: By hiding the implementation details, encapsulation prevents unintended changes to the internal state of objects.
Provides Security: Encapsulation restricts direct access to sensitive data, enhancing security and preventing unauthorized manipulation.
Provides Reusability: Encapsulation promotes code reusability by encapsulating functionality within classes, allowing them to be reused in different parts of the program.
Access Modifiers in Python Encapsulation
In Python, access modifiers are used to control the visibility of class members. There are three types of access modifiers:
Public: Members are accessible from outside the class.
Protected: Members are accessible within the class and its subclasses.
Private: Members are accessible only within the class itself.
Advantages:
Enhanced security and data protection
Improved code organization and readability
Promotes code reusability and maintainability
Drawbacks:
Overuse of encapsulation can lead to excessive complexity
Increased overhead in terms of memory and performance
Conclusion
Encapsulation is a powerful concept in Python that allows for better organization, security, and reusability of code. By encapsulating data and methods within classes and controlling access to them, Python enables developers to build robust and maintainable applications.
FAQs
Q: What is encapsulation with an example?
A: Encapsulation is the bundling of data and methods within a class, restricting access to them from outside. For example, in Python, we can define a class Car with private attributes model and year, and provide getter methods to access them.
Q: What is a real-life example of encapsulation in Python?
A: A real-life example of encapsulation in Python can be seen in a bank account system, where the account balance is encapsulated within a class, and access to it is controlled through methods like deposit and withdrawal.
Q: What are abstraction and encapsulation in OOPs Python?
A: Abstraction is the process of hiding the implementation details and showing only the essential features of an object. Encapsulation, on the other hand, is the bundling of data and methods within a class to restrict access to them from outside.
Q: How will a class protect the code inside it?
A: By encapsulating data and methods within a class and controlling access to them using access modifiers, a class ensures that the internal state of objects is protected from unauthorized access and manipulation.
Comments