
Mongoose is a MongoDB and Node.js Object Data Modelling (ODM) module. It manages data associations, does schema validation, and is used to translate between objects in code and their representation in MongoDB.
MongoDB is a NoSQL document database with no schema. It means that JSON documents can be stored in it, and the structure of these documents might vary because it is not enforced like SQL databases. This is one of the benefits of utilizing NoSQL because it speeds up application development and decreases deployment complexity.
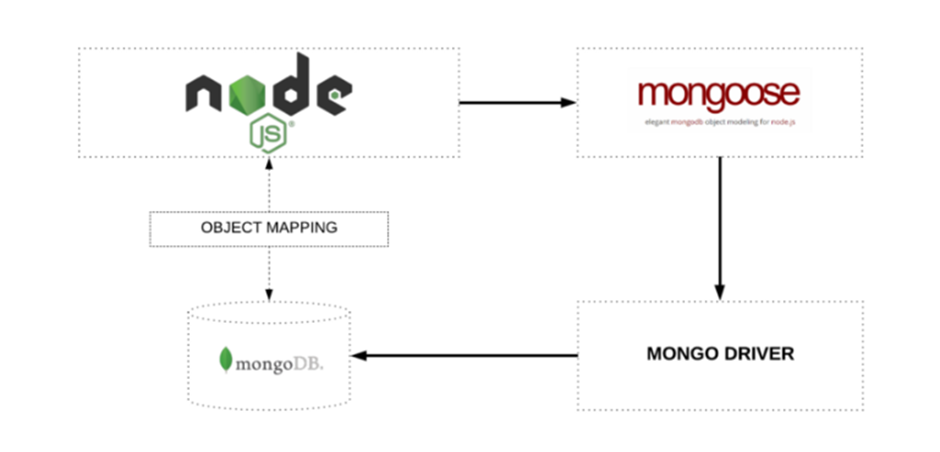
Using the Mongoose library in Node.js applications to interface with MongoDB requires the creation of Mongoose instances. Mongoose is an Object Data Modelling (ODM) library that defines schemas, models, and documents to work with MongoDB and clearly.
When using Mongoose, you start by creating a schema that defines your data's structure and validation rules. The schema is a blueprint for creating model instances and individual documents that follow the stated template. These are the data instances that will be saved in the MongoDB database.
Developers can use Mongoose to access capabilities like data modeling, validation, middleware, and a high-level API for database operations. Mongoose makes it easier to create, query, update, and delete data in MongoDB, allowing developers to focus on developing powerful and scalable applications.
During this phase, developers construct schemas that specify the attributes, their data types, and any validation criteria. They then build models based on these schemas, which serve as interfaces to the database. These models can generate instances of documents that conform to the given schema, allowing for a more convenient and structured approach to working with data.
Overall, using Mongoose instances allows developers to exploit the power of MongoDB in a more organized and effective manner. It encourages consistency, validity, and flexibility in data management, making it a must-have tool for Node.js developers working with MongoDB as the underlying database.
Starting Over
Let's get Mongo up and running first. You can select one of the following alternatives (we'll go with option #1 for this article):
Step 1: Download the MongoDB version appropriate for your operating system from the MongoDB website and follow the installation instructions.
Step 2: Create a free mLab sandbox database subscription (optional).
Step 3: If you want, you can install Mongo using Docker.
Step 4: Let's go through some of the fundamentals of Mongoose by creating a model that represents data for an introductory address book.
Basic Terminologies
1. Collections
Mongo collections are analogous to relational database tables. They can hold several JSON documents.
2. Documents
In SQL, documents are equivalent to records or rows of data. While a SQL row can relate to data in other tables, Mongo documents often integrate such information into a single document.
3. Fields
Fields, often properties or attributes, are similar to SQL table columns. FirstName, LastName, Email, and Phone are all fields in the image above.
4. Schema
While Mongo has no schema, SQL defines one through the table definition. A Mongoose schema is a document data structure (or document shape) enforced through the application layer.
5. SchemaTypes
While Mongoose schemas determine a document's general structure or shape, SchemaTypes specify the anticipated data type for specific fields (String, Number, Boolean, and so on).
You can also throw in important parameters such as those needed to make a field non-optional, default to specify the field's default value, and many more.
6. Models
Models are higher-order constructors that take a schema and build a document instance corresponding to relational database records.
Example
Here's a little code piece to demonstrate some of the terminology mentioned earlier:
const puppySchema = new Mongoose.Schema({
name: {
type: String,
required: true
},
age: Number
});
const Puppy = mongoose.model('Puppy', puppySchema);
PuppySchema determines the shape of the document, which contains two fields, name, and age, in the code above.
The name SchemaType is String, while the age SchemaType is Number. You may define the SchemaType for a field by using an object with a type attribute, just like you do with a name. Alternatively, as with age, you can apply a SchemaType directly to the field.
Also, the SchemaType for the name has the needed option set to true. To use necessary and lowercase options for a field, you must use an object to set the SchemaType.
PuppySchema is compiled at the bottom of the sample into a model named Puppy, which can be used to generate documents in an application.
Creating instances of Mongoose
Creating Mongoose instances entails first defining a schema for your data and then utilizing that schema to construct model instances. Mongoose is a MongoDB Object Data Modelling (ODM) module that provides an easy way to interface with the database by defining schemas, models, and documents.
Here are the steps for creating Mongoose instances:
Step 1: Setup Mongoose: First, install the Mongoose package in your project. You may install it with npm (Node Package Manager) by running the following command in your project directory:
npm install Mongoose
Step 2: Import Mongoose: Using the required line, import the Mongoose module into your Node.js application:
const mongoose = require('mongoose');
Step 3: Connecting to MongoDB: Use the Mongoose.connect() method to connect to your database. Check that MongoDB is installed and operating locally, or supply the correct connection URL if using a remote database.
mongoose.connect('mongodb://localhost/mydatabase', {
useNewUrlParser: true,
useUnifiedTopology: true
})
.then(() => {
console.log('Connected to MongoDB');
})
.catch((error) => {
console.error('Error connecting to MongoDB:', error);
});
Step 4 Defining a Schema: A schema defines your data's structure and validation criteria. Using the Mongoose, create a new Mongoose Schema.Declare the properties and their types in the Schema() constructor.
const userSchema = new Mongoose.Schema({
name: String,
age: Number,
email: String
});
String, Number, Boolean, Date, and other types can be defined for your properties. Mongoose also lets you specify different validation settings for each property, such as mandatory fields, minimum and maximum values, custom validation functions, etc.
Step 5 Construct a Model: After specifying the schema, use the Mongoose.model() method to construct a model. The model enables database operations on documents depending on the stated schema.
const User = mongoose.model('User', userSchema);
The unique name of the collection that will be built for the model is supplied as the first argument to Mongoose.model(). To discover or build the relevant collection in the database, Mongoose will automatically pluralize the name.
Step 6 Construct Instances: Now that you've defined the model, you may construct instances (documents) based on it. To make a new instance, instantiate the model as an ordinary JavaScript object and fill in the values for its properties.
const newUser = new User({
name: 'John Doe',
age: 25,
email: 'john@example.com'
});
Based on your schema definition, you can assign values to each property. You can also change the document's properties before saving it and perform any other necessary procedures.
Step 7 Save the Instance: To save the newly formed instance to the database, use the instance's save() method. This will save the document to the MongoDB database asynchronously.
newUser.save()
.then(() => {
console.log('User saved successfully!');
})
.catch((error) => {
console.error('Error saving user:', error);
});
The save() method returns a Promise, which allows you to manage the save operation's success or failure using.then() and.catch(), respectively.
Mongoose supports a variety of ways for querying, updating, and deleting documents from the database in addition to saving them. You may use Mongoose's capabilities to deal with MongoDB efficiently in your Node.js apps by following these instructions and reviewing the documentation.
The Benefits of Creating Mongoose Instances
Creating Mongoose objects and using them in your Node.js application has various benefits:
Data Modelling: Using schemas, Mongoose formally defines data models. Schemas help specify your data's structure, data types, and validation criteria, assuring consistency and integrity in your application.
Object-Oriented Programming (OOP) Paradigm: Mongoose models and instances adhere to OOP concepts, allowing you to interact with data object-oriented. Model instances can be created, data can be manipulated via object methods, and links between models can be established.
Simple MongoDB Interaction: Mongoose simplifies the complexities of working with MongoDB by providing a high-level API. It makes creating, reading, updating, and deleting (CRUD) operations, indexing, querying, and aggregating easier. Mongoose's methods and query builders make designing more readable and straightforward MongoDB queries easy.
Mongoose provides middleware capabilities that allow you to construct before and post hooks for various processes, such as saving, updating, and removing documents. This feature allows you to run custom logic or change data before or after specified database events, giving you more flexibility and control over your data flow.
Validation and Data Consistency: Mongoose includes built-in validation features for MongoDB data. Validation rules can be defined within the schema, such as necessary fields, minimum and maximum values, data types, custom validators, and more. This aids in preserving data consistency and integrity by preventing invalid or inconsistent data from being kept in the database.
Mongoose supports data population and referencing, allowing you to populate fields with actual data rather than merely references. You can build relationships between distinct models and use those ties to populate fields. This feature makes it easier to query and get related data from many collections.
Flexibility and Scalability: Mongoose provides a scalable and versatile way to work with MongoDB. As your application matures, you can modify your data models and schemas.
Fields, indexes, relationships, and validation rules can be quickly added or modified without requiring sophisticated database changes.
Mongoose has a strong and active community that provides assistance, tools, and plugins. The ecosystem includes a variety of plugins and extensions that extend Mongoose's capability, enabling features such as geospatial querying, full-text search, data encryption, and more.
Using Mongoose to interface with MongoDB adds structure, simplicity, and flexibility to your Node.js application, simplifying data management and increasing overall productivity.
Conclusion
Finally, establishing Mongoose instances and using them in your Node.js application provides various benefits for dealing with MongoDB. Mongoose uses a data modeling approach called schemas to promote organization and consistency, allowing you to define your data's structure, data types, and validation criteria. This object-oriented paradigm allows for more intuitive and organized data manipulation.
Mongoose simplifies database processes such as CRUD, querying, indexing, and aggregating by abstracting MongoDB's intricacies. Its high-level API and query builders make designing readable and maintainable MongoDB queries easy.
The middleware and hooks feature in Mongoose allows you to perform custom logic before or after specified database actions, giving you more control and flexibility over your data flow.
Built-in validation tools assure data consistency and integrity by enforcing schema-defined rules and constraints.
The ability to build model associations and populate data allows for quick querying and retrieval of relevant data from many collections.
The flexibility and scalability of Mongoose allow for easy adaption of data models and schemas as your application evolves, without the need for complex database updates. Furthermore, Mongoose's active community and extensive ecosystem provide resources, support, and plugins to extend its capability and answer specific application needs.
To summarise, using Mongoose instances adds structure, ease, and efficiency to your Node.js application's interaction with MongoDB. It makes data management easier, streamlines complex tasks, and allows you to construct scalable and robust applications easily.
Interesting F.A.Qs
What are the fundamental abilities of a MERN stack developer?
We, in CipherSchools believe here are the fundamental skills of a MERN stack developer:
· JavaScript knowledge
· React (React.js) knowledge
· Understanding of Node.js and experience with Express.js
· Knowledge of MongoDB
· HTML/CSS expertise
· Git (version control) abilities
· RESTful API comprehension
· Understanding of web development ideas
· Excellent problem-solving and debugging skills
· These abilities serve as the foundation for a MERN stack developer and give the experience required to construct applications with the MERN stack.
What does a MERN stack developer do in India?
The MERN stack is a prominent online application development technology stack that includes MongoDB, Express, React, and Node.js. The MERN stack has a promising future in India and around the world, owing to the growing demand for web apps and the advantages of developing them with the MERN stack.
According to Quora, the MERN stack has a promising future in India, with numerous prospects for MERN stack engineers in a variety of businesses. To take advantage of these opportunities, developers must continue to learn about new technologies and best practises.
Stay tuned to CipherSchools for more interesting tech articles.
Read More: Understanding Mongoose Schemas and Models
Comments