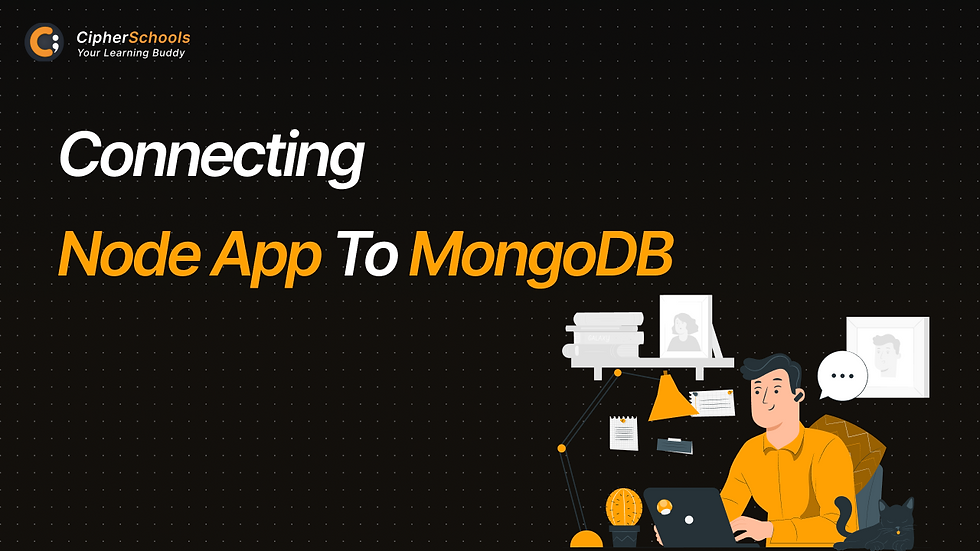
Node.js, a popular JavaScript runtime, offers a stable and scalable framework for developing server-side applications. When designing Node.js web applications, persisting data in a database is frequently required for efficient storage and retrieval. MongoDB, a NoSQL document database, provides a scalable and versatile alternative for managing data in JSON-like format. In this post, we will look at how to connect a Node.js application to MongoDB, allowing the application and the database to communicate seamlessly.
Installing the necessary prerequisites, specifying the connection settings, and interacting with the database using the MongoDB driver for Node.js are all processes in connecting a Node.js app to MongoDB. This MongoDB-approved driver provides a high-level API for executing database operations such as inserting, updating, and querying documents.
This article will show you how to set up and connect a Node.js application to a MongoDB database. We'll review the fundamentals, such as installing the required packages, specifying the connection string, establishing the connection, executing CRUD (Create, Read, Update, Delete) operations, and dealing with problems.
Understanding how to connect your application to a database is critical whether you are a newbie or an experienced Node.js developer. By the end of this lesson, you'll have the knowledge and tools you need to integrate MongoDB into your Node.js projects, allowing you to create robust and data-driven applications.
MongoDB is a free and open-source NoSQL database management system. NoSQL databases are convenient when dealing with massive amounts of scattered data. We can store data in this database, manage it, and then retrieve and show it to app users.
To connect to a Mongo database from a Node.js server, you can use one of two methods:
Mongo, the official npm driver package
Mongoose is a beautiful object modeling tool for MongoDB written in NodeJS.
Most online tutorials show how to use Mongoose to set up a Node/Express app with MongoDB, which isn't helpful for novices.
In this article, we'll review the fundamentals of creating a MongoDB account, building a connection string, and connecting your MongoDB database to your project without using Mongoose. So, let's get started and see how to connect a Node.js app to MongoDB!
Step 1: Make an account.
Go to the MongoDB Atlas website and sign up with your email address.
To create your account, you may be required to complete a brief questionnaire.
Step 2: Set up your first cluster.
A cluster is a collection of machines that store copies of your database.
Select the shared database type (the free one!) when prompted to construct a database.
It will give you options to customize your cluster, but you can leave it alone. Ensure that you are still in the free tier. Then, click on Create cluster.
Step 3: Establish a username and password.
You must create a database user while your cluster is formed in the background. The username and password differ from those you used to log into MongoDB Atlas; they will only be used for this database (this helps keep the data secure and allows you to restrict who has access to what data).
Please keep your password safe since we'll need it to connect to the database.
Step 4: Enter your IP address.
Before connecting to your cluster, you must whitelist your IP address. This is a built-in security feature of MongoDB Atlas. Proceed to click. Fill up your current IP address.
After that, click Finish and Close, and then, when the dialogue box appears, click Go to Databases.
Step 5: Return to the Home Page
Welcome to your database site; you should notice your cluster there. Establishing a collection within it would be best before connecting it to your app.
Step 6: Make a collection.
Browse Collections, then Add My Own Data.
Enter the name of your database and the name of the collection. In my example, the database name will be 'to-do-app,' and the collection name will be 'tasks.' Select Create.
You have successfully created a collection in which all your objects will be saved.
Step 7: Make a connection string.
Return to your database homepage (top left, under DEPLOYMENT), and then select Connect on your cluster's main page. You'll be given three options for connecting to your database.
Select the "Connect your application" option.
The connection string given will be utilized by your app (to connect to and manipulate the database).
Step 8: Begin your project.
Launch your IDE (It is always recommended to VS Code here) and launch your app.
If this is your first time creating a full-stack online application, you must install Node.js on your computer.
It is available for download from the official node website, where it will also install npm (Node Package Manager).
npm is a Node.js package manager containing hundreds of thousands of packages. Although it creates some of your directory structure/organization, there are other goals than this.
Its primary objective is to manage dependencies and packages automatically. This means you can include your project's dependencies in the package.json file.
When you (or anyone else) need to start your project, they can execute the npm install, and all requirements will be installed. Furthermore, it is possible to indicate which versions your project depends on to prevent changes from breaking your project.
Create a project folder and change the directory to your project's folder to get started with npm.
Once this is completed, you are ready to begin your first project with the following:
npm init ## triggers the initialization of a project
Step 9: Set up local packages
When you install a package with npm, it is installed as a dependency in your app within your package. The node_modules subdirectory contains the JSON file and its modules.
The typical NPM command for installing a package is npm install.
npm install <package name>
Let's now install the packages we'll need for this project.
● In Express:
npm install express
● In MongoDB
npm install mongodb
● In Dotenv
npm install dotenv
Note: The dotenv package contains sensitive data we do not wish to share. It sets environment variables and conceals them from our main code, allowing you to secure private keys in an env file and keep them out of sight when publishing to GitHub.
Check your package-lock.json (under packages) to see if everything was correctly installed.
Step 10: Establish dependencies
Now that the packages have been installed open your code editor and create a new server.js file. Then, include the following code:
const express = require('express') #1
const app = express() #2
const MongoClient = require('mongodb').MongoClient. #3
Explanation of the Code:
You utilize the required function in your first line of code to incorporate the "express module."
It would be best to create an object of the express module before using it.
MongoDB module exports MongoClient, which you'll use to connect to a MongoDB database. You can use a MongoClient instance to connect to a cluster, access the database, and then disconnect from that cluster.
Step 11: Connect to the database
You must declare a variable for the string supplied by MongoDB. IMPORTANT: Replace with the same password you created earlier.
const connectionString = 'mongodb+srv://juliafm:<password>@cluster0.qvesujr.mongodb.net/?retryWrites=true&w=majority'
Add the following lines of code to complete the connection to the external database:
MongoClient.connect(connectionString)
.then(client => {
console.log(`Connected to Database`)
const db = client.db('to-do-app')
const tasksCollection = db.collection('tasks')
})
//CRUD requests
.catch(error => console.error(error))
Explanation of the Code:
Make a function that includes a promise.
Please tell me what I should do next (then). client
It should be passed as an argument to the arrow function.
Make a console.log statement to ensure that you are connected.
Indicate which database and collection you want to connect to.
Add a catch statement to see if any mistakes occur.
That's fantastic; you're all set. If you restart your server, you should see the message "Connected to Database" on your terminal.
So far, you have the following code:
const express = require('express')
const app = express()
const MongoClient = require('mongodb').MongoClient
const connectionString = 'mongodb+srv://juliafm:helloworld@cluster0.jgbyhks.mongodb.net/?retryWrites=true&w=majority'
MongoClient.connect(connectionString)
.then(client => {
console.log('Connected to database')
const db = client.db('to-do-app')
const taskCollection = db.collection('tasks')
//CRUD request
})
.catch(error=> console.error(error))
Step 12: Create a .env file.
Now, we like to show you how to relocate your MongoDB connection string to a.env file for security reasons.
To do so, create a.env file in the folder containing your project. Create a variable DB_STRING in this file and insert your connection string (remember to replace the placeholder with your actual password).
Return to your server.Remove the variable that carries the connection string from the js file and replace it with this:
require('dotenv').config()
const connectionString = process.env.DB_STRING;
Today, you used a Node.js script to connect to a MongoDB database, receive a list of databases in your cluster, and examine the results in your console. Nice!
Now that you've established a connection to your database proceed to the next post in this series to learn how to do each of the CRUD (create, read, update, and delete) actions.
Why should you link your Node app to MongoDB?
There are various advantages to connecting a Node.js application to MongoDB. Here are a few of the main reasons:
Data Storage that is Flexible and Scalable: MongoDB is a NoSQL document database that enables flexible data modeling. It saves data in a JSON-like format called BSON (Binary JSON), compatible with Node.js' JavaScript objects.
This adaptability allows you to modify your data structure as your application evolves. Furthermore, MongoDB is built to grow horizontally, allowing you to handle massive amounts of data and significant traffic loads easily.
JavaScript Integration: JavaScript is the primary programming language used by both Node.js and MongoDB. This same language enables seamless integration of the two technologies. You can use the same syntax and structures to deal with MongoDB in Node.js, making it easier to design, manage, and debug your application.
Non-blocking and asynchronous Nature: Because of its asynchronous and non-blocking I/O style, Node.js is well-suited to handle concurrent processes. The MongoDB Node.js driver is designed to work with this architecture, allowing you to conduct database operations asynchronously without interrupting the application's execution. This asynchronous approach improves your application's overall performance and responsiveness.
Rich Querying and Aggregation features: MongoDB has robust querying and aggregation features that allow you to access and analyze data effectively. You can use these functionalities in your Node.js application using the MongoDB driver for Node.js.
MongoDB delivers a comprehensive collection of functionalities to satisfy your needs, whether you need to search for individual documents, perform complicated aggregations, or apply data transformations.
Active and supportive developer communities: Node.js and MongoDB have healthy and vibrant developer communities. This means numerous tools, tutorials, frameworks, and community assistance are available to assist you in connecting your Node.js app to MongoDB.
The accumulated knowledge and experience of the community can help you overcome obstacles and improve the quality and performance of your application.
Connecting a Node.js application to MongoDB allows you to take advantage of a flexible and scalable database solution that works in tandem with your Node.js development environment.
This integration enables you to manage and retrieve data more efficiently, grow your application, and benefit from the thriving community surrounding Node.js and MongoDB.
Conclusion
In this article, we looked at how to connect a Node.js application to MongoDB, a robust NoSQL database. We learned the essential procedures for securing, such as installing the required prerequisites, configuring the connection string, and using the MongoDB driver for Node.js.
We obtained access to a flexible and scalable database solution capable of efficiently managing our Node.js app's data by connecting it to MongoDB. Using the MongoDB driver's simple API, we found how to do CRUD tasks such as creating, reading, updating, and deleting documents.
You now have the knowledge and tools to quickly construct data-driven applications after learning to connect Node.js to MongoDB. Whether creating a web application, an API, or another server-side solution, using MongoDB can dramatically improve your capacity to store and retrieve data.
When working with MongoDB, consider best practices, such as error handling, security precautions, and efficient query architecture. Using the expansive ecosystem of libraries, frameworks, and tools available for Node.js with MongoDB to streamline your development process further.
Now that you know how to connect your Node.js app to MongoDB, you can use the power of a flexible and scalable database to create solid and data-driven applications. Have fun exploring the possibilities, and keep honing your Node.js and MongoDB abilities to develop even more remarkable projects in the future!
Interesting F.A.Qs
What is the MERN Stack Developer Roadmap?
There are several paths to becoming a full-stack developer. To become a fullstack developer, you can work with a variety of technologies. We will discuss a path that begins with HTML, CSS, and JS and then progresses to learning the MERN stack.
The MERN stack stands for MongoDB, Express, React, and Nodejs. The backend is built with Nodejs and Express, the frontend is built with React, and the database is MongoDB. The MERN stack has grown in popularity due to its ease of use and speed in developing applications.
What Is the Role of a MERN Stack Developer?
MERN developers are in charge of producing reusable, testable, and efficient code, as well as developing and designing front end web applications. They are in charge of incorporating CSS approaches and technologies into the development of full websites.
Stay tuned to CipherSchools for more interesting tech articles.
Comments